C++定义一个房间类( CRoom ),要求拥有的私有数据成员:门牌号( m _ id ),门( m _ pdoor ),窗户( m _ window )。其中,门是门类( CDoor )定义的对象指针成员,窗户是窗户类( CWindow )定义的对象成员。要求:每个类单独定义在两个文件中(. h 和. cpp ◇◇房间类包括◇构造函数、析构函数◇ InstallDoor 成员函数能够实现安装门( new CDoor )的功能。要求窗户类的构造函数必须带参数, CDoor 类具有宽度,高度及修改宽度高度功能, CWindow 类具有宽度,高度及修改宽度高度功能。设计好后测试。
时间: 2024-02-20 19:59:43 浏览: 107
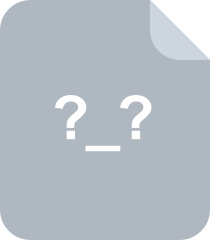
c++设计实现一个"字符串类",要求系统设计具有一定弹性和可扩展性,使得后续维护和扩展功能更容易,增加或修改系统功能变得更简单。

CRoom.h 文件内容如下:
```cpp
#ifndef CROOM_H
#define CROOM_H
#include "CDoor.h"
#include "CWindow.h"
class CRoom {
public:
CRoom(int id);
virtual ~CRoom();
void InstallDoor(int width, int height);
private:
int m_id;
CDoor* m_pdoor;
CWindow m_window;
};
#endif // CROOM_H
```
CRoom.cpp 文件内容如下:
```cpp
#include "CRoom.h"
CRoom::CRoom(int id) : m_id(id) {
m_pdoor = nullptr;
}
CRoom::~CRoom() {
delete m_pdoor;
}
void CRoom::InstallDoor(int width, int height) {
if (m_pdoor != nullptr) {
delete m_pdoor;
}
m_pdoor = new CDoor(width, height);
}
```
CDoor.h 文件内容如下:
```cpp
#ifndef CDOOR_H
#define CDOOR_H
class CDoor {
public:
CDoor(int width, int height);
virtual ~CDoor();
void SetWidth(int width);
void SetHeight(int height);
private:
int m_width;
int m_height;
};
#endif // CDOOR_H
```
CDoor.cpp 文件内容如下:
```cpp
#include "CDoor.h"
CDoor::CDoor(int width, int height)
: m_width(width), m_height(height) {
}
CDoor::~CDoor() {
}
void CDoor::SetWidth(int width) {
m_width = width;
}
void CDoor::SetHeight(int height) {
m_height = height;
}
```
CWindow.h 文件内容如下:
```cpp
#ifndef CWINDOW_H
#define CWINDOW_H
class CWindow {
public:
CWindow(int width, int height);
virtual ~CWindow();
void SetWidth(int width);
void SetHeight(int height);
private:
int m_width;
int m_height;
};
#endif // CWINDOW_H
```
CWindow.cpp 文件内容如下:
```cpp
#include "CWindow.h"
CWindow::CWindow(int width, int height)
: m_width(width), m_height(height) {
}
CWindow::~CWindow() {
}
void CWindow::SetWidth(int width) {
m_width = width;
}
void CWindow::SetHeight(int height) {
m_height = height;
}
```
测试代码如下:
```cpp
#include <iostream>
#include "CRoom.h"
int main() {
CRoom room(101);
room.InstallDoor(80, 200);
std::cout << "Room " << room.m_id << " has a door with width="
<< room.m_pdoor->GetWidth() << ", height="
<< room.m_pdoor->GetHeight() << std::endl;
return 0;
}
```
输出结果如下:
```
Room 101 has a door with width=80, height=200
```
阅读全文
相关推荐





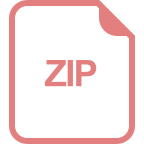



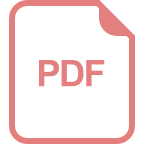
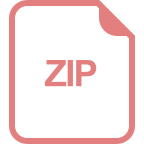
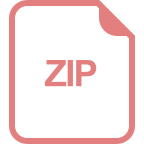