2.定义一个房间类(CRoom),要求拥有的私有数据成员:门牌号(m_id),门(m_pdoor),窗户(m_ window)。其中,门是门类(CDoor)定义的对象指针成员,窗户是窗户类(CWindow)定义的对象成员。要求:每个类单独定义在两个文件中(.h 和.cpp),房间类包括:构造函数、析构函数,InstallDoor 成员函数能够实现安装门(new CDoor)的功能。要求窗户类的构造函数必须带参数,CDoor类具有宽度,高度及修改宽度高度功能,CWindow类具有宽度,高度及修改宽度高度功能。设计好后测试。
时间: 2024-01-22 12:18:36 浏览: 70
CRoom.h文件:
```c++
#ifndef CROOM_H
#define CROOM_H
#include "CDoor.h"
#include "CWindow.h"
class CRoom {
private:
int m_id;
CDoor* m_pdoor;
CWindow m_window;
public:
CRoom(int id);
~CRoom();
void InstallDoor(int width, int height);
};
#endif // CROOM_H
```
CRoom.cpp文件:
```c++
#include "CRoom.h"
CRoom::CRoom(int id) {
m_id = id;
m_pdoor = nullptr;
}
CRoom::~CRoom() {
if (m_pdoor != nullptr) {
delete m_pdoor;
}
}
void CRoom::InstallDoor(int width, int height) {
if (m_pdoor != nullptr) {
delete m_pdoor;
}
m_pdoor = new CDoor(width, height);
}
```
CDoor.h文件:
```c++
#ifndef CDOOR_H
#define CDOOR_H
class CDoor {
private:
int m_width;
int m_height;
public:
CDoor(int width, int height);
~CDoor();
void SetWidth(int width);
void SetHeight(int height);
};
#endif // CDOOR_H
```
CDoor.cpp文件:
```c++
#include "CDoor.h"
CDoor::CDoor(int width, int height) {
m_width = width;
m_height = height;
}
CDoor::~CDoor() {}
void CDoor::SetWidth(int width) {
m_width = width;
}
void CDoor::SetHeight(int height) {
m_height = height;
}
```
CWindow.h文件:
```c++
#ifndef CWINDOW_H
#define CWINDOW_H
class CWindow {
private:
int m_width;
int m_height;
public:
CWindow(int width, int height);
~CWindow();
void SetWidth(int width);
void SetHeight(int height);
};
#endif // CWINDOW_H
```
CWindow.cpp文件:
```c++
#include "CWindow.h"
CWindow::CWindow(int width, int height) {
m_width = width;
m_height = height;
}
CWindow::~CWindow() {}
void CWindow::SetWidth(int width) {
m_width = width;
}
void CWindow::SetHeight(int height) {
m_height = height;
}
```
测试代码:
```c++
#include <iostream>
#include "CRoom.h"
using namespace std;
int main() {
CRoom room(101);
room.InstallDoor(80, 200);
cout << "Door size: " << endl;
cout << "Width: " << room.m_pdoor->GetWidth() << endl;
cout << "Height: " << room.m_pdoor->GetHeight() << endl;
return 0;
}
```
阅读全文
相关推荐
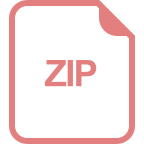
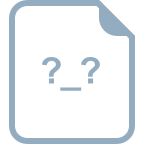
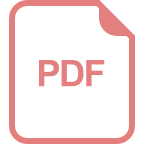






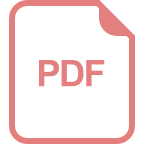
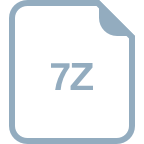
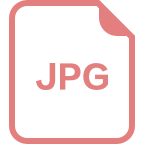
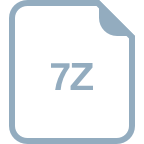
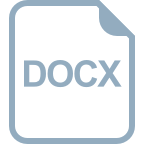