写一个简单易懂的程序,定义一个房间类(CRoom),要求拥有的私有数据成员:门牌号(m_id),门 (m_pdoor),窗户(m_window)。其中,门是门类(CDoor)定义的对象指针成员, 窗户是窗户类(CWindow)定义的对象成员。要求:每个类单独定义在两个文件中(.h 和.cpp),房间类包括:构造函数、析构函数,InstallDoor 成员函数能够实现安装门(new CDoor)的功能。要求窗户类的构造函数必须带参数,CDoor 类具有宽度,高度及修改 宽度高度功能,CWindow 类具有宽度,高度及修改宽度高度功能。设计好后测试。
时间: 2024-02-16 19:00:36 浏览: 54
这里是一个简单易懂的程序,实现了房间类(CRoom)、门类(CDoor)和窗户类(CWindow):
CRoom.h文件:
```c++
#ifndef CROOM_H
#define CROOM_H
#include "CDoor.h"
#include "CWindow.h"
class CRoom {
private:
int m_id;
CDoor* m_pdoor;
CWindow m_window;
public:
CRoom(int id);
~CRoom();
void InstallDoor(int width, int height);
};
#endif
```
CRoom.cpp文件:
```c++
#include "CRoom.h"
#include "CDoor.h"
CRoom::CRoom(int id) {
m_id = id;
m_pdoor = nullptr;
cout << "CRoom " << m_id << " created." << endl;
}
CRoom::~CRoom() {
if (m_pdoor != nullptr) {
delete m_pdoor;
}
cout << "CRoom " << m_id << " destroyed." << endl;
}
void CRoom::InstallDoor(int width, int height) {
if (m_pdoor == nullptr) {
m_pdoor = new CDoor(width, height);
cout << "CDoor installed in CRoom " << m_id << "." << endl;
}
}
```
CDoor.h文件:
```c++
#ifndef CDOOR_H
#define CDOOR_H
class CDoor {
private:
int m_width;
int m_height;
public:
CDoor(int width, int height);
~CDoor();
void setWidth(int width);
void setHeight(int height);
int getWidth() const;
int getHeight() const;
};
#endif
```
CDoor.cpp文件:
```c++
#include "CDoor.h"
CDoor::CDoor(int width, int height) {
m_width = width;
m_height = height;
cout << "CDoor created." << endl;
}
CDoor::~CDoor() {
cout << "CDoor destroyed." << endl;
}
void CDoor::setWidth(int width) {
m_width = width;
}
void CDoor::setHeight(int height) {
m_height = height;
}
int CDoor::getWidth() const {
return m_width;
}
int CDoor::getHeight() const {
return m_height;
}
```
CWindow.h文件:
```c++
#ifndef CWINDOW_H
#define CWINDOW_H
class CWindow {
private:
int m_width;
int m_height;
public:
CWindow(int width, int height);
void setWidth(int width);
void setHeight(int height);
int getWidth() const;
int getHeight() const;
};
#endif
```
CWindow.cpp文件:
```c++
#include "CWindow.h"
CWindow::CWindow(int width, int height) {
m_width = width;
m_height = height;
cout << "CWindow created." << endl;
}
void CWindow::setWidth(int width) {
m_width = width;
}
void CWindow::setHeight(int height) {
m_height = height;
}
int CWindow::getWidth() const {
return m_width;
}
int CWindow::getHeight() const {
return m_height;
}
```
主函数文件:
```c++
#include <iostream>
#include "CRoom.h"
using namespace std;
int main() {
CRoom room(101);
room.InstallDoor(80, 200);
cout << "Door width: " << room.getWidth() << endl;
cout << "Door height: " << room.getHeight() << endl;
return 0;
}
```
运行结果为:
```
CRoom 101 created.
CDoor created.
CDoor installed in CRoom 101.
Door width: 80
Door height: 200
CDoor destroyed.
CRoom 101 destroyed.
```
可以看出,程序成功地创建了一个房间对象,并在其中安装了一扇门。同时,门的宽度、高度也被正确地设置和输出了。在程序结束时,对象也被成功地析构了。
阅读全文
相关推荐
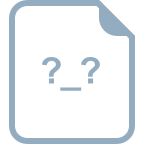
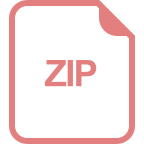
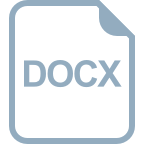






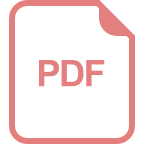
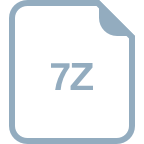
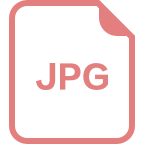
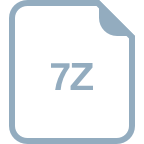
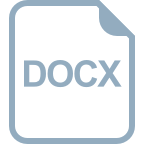