用python正则表达式提取姓名
时间: 2023-10-31 08:56:32 浏览: 229
可以使用以下正则表达式来提取姓名:
```python
import re
text = "My name is John Doe. Nice to meet you!"
name_regex = r'\b[A-Z][a-z]+\s[A-Z][a-z]+\b'
name = re.findall(name_regex, text)
print(name)
```
输出结果为:
```
['John Doe']
```
解释一下正则表达式:
- `\b` 表示单词边界,确保我们匹配的是一个完整的单词。
- `[A-Z]` 匹配任意大写字母。
- `[a-z]+` 匹配一个或多个小写字母。
- `\s` 匹配一个空格。
- `+` 表示前面的字符可以出现一次或多次。
所以,`\b[A-Z][a-z]+\s[A-Z][a-z]+\b` 匹配的是一个由两个单词组成的、第一个单词首字母大写、第二个单词首字母也大写的字符串,即一个常见的英文姓名格式。
相关问题
Python用正则表达式提取一段文字中的姓名
可以使用Python的re模块中的正则表达式函数来提取一段文字中的姓名。
以下是提取一段文字中的姓名的示例代码:
```python
import re
text = "张三、李四和王五都是这个班的学生。"
pattern = r'[\u4e00-\u9fa5]{2,4}' # 匹配2-4个汉字,即名字的长度
match_results = re.findall(pattern, text) # 查找所有匹配的结果
names = []
for name in match_results:
if name not in names:
names.append(name)
print(names) # 输出匹配的姓名列表
```
上述代码使用了正则表达式`[\u4e00-\u9fa5]{2,4}`来匹配2-4个汉字,这个范围通常是一个名字的长度范围。接下来,使用`re.findall()`函数查找所有匹配的结果,并将其保存在一个列表中。
由于同一个人的姓名可能会在一段文字中出现多次,所以我们需要在列表中去重,最后输出匹配的姓名列表。
使用Python,编程以下问题:令字符串 s='马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You've given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!' ,通过编写函数实现下述各题功能。 1 应用正则表达式判断该字符串是否包含0~9的数字和大小写英文字母。 2 根据正则表达式使用中文逗号、中文句号、英文逗号、英文点号、英文感叹号、英文换行符号将该字符串分割成多个子串。 3 应用正则表达式提取该字符串中的日期时间字符串。 4 应用正则表达式提取该字符串中的诗词名称。 5 应用正则表达式提取该字符串中的姓名。
1.
import re
def has_alpha_digit(s):
return bool(re.search('[A-Za-z0-9]', s))
s='马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
print(has_alpha_digit(s))
2.
import re
def split_strings(s):
return re.split('[,。,.;!\n]+', s)
s='马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
print(split_strings(s))
3.
import re
def extract_datetime(s):
return re.findall('\d{4}-\d{1,2}-\d{1,2} \d{1,2}:\d{1,2}', s)
s='马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
print(extract_datetime(s))
4.
import re
def extract_poem_name(s):
return re.findall('《([^《》]+)》', s)
s='马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
print(extract_poem_name(s))
5.
import re
def extract_name(s):
return re.findall('([^\n]+)你', s)[0]
s='马丽于2022-4-29 10:10刊发了一首诗《Listen to me》,诗词的大意是:\n Mama you taught me to do the right things,\n So now you have to let your baby fly.\n You\'ve given me everything that I will need,\n To make it through this crazy thing called life,\n Thank you Mum!'
print(extract_name(s))
阅读全文
相关推荐
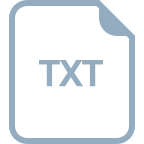
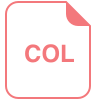
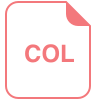
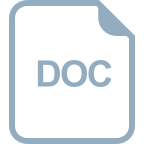
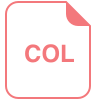
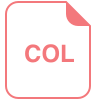
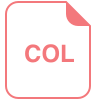
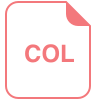
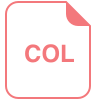
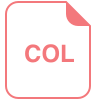
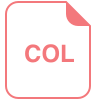
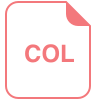


