请给我一段按自定义消费策略进行分配的,有10个生产者和10个消费者的python3代码
时间: 2024-03-03 13:53:54 浏览: 40
好的,以下是一个简单的实现:
```python
import threading
import queue
import random
# 定义生产者线程
class ProducerThread(threading.Thread):
def __init__(self, queue, strategy_func):
super().__init__()
self.queue = queue
self.strategy_func = strategy_func
def run(self):
while True:
# 模拟生产任务
task = random.randint(1, 100)
# 调用策略函数进行任务分配
worker_index = self.strategy_func()
# 将任务放入对应的消费者队列中
self.queue[worker_index].put(task)
# 定义消费者线程
class ConsumerThread(threading.Thread):
def __init__(self, queue):
super().__init__()
self.queue = queue
def run(self):
while True:
# 从队列中取出任务
task = self.queue.get()
# 模拟处理任务的时间
time.sleep(random.randint(1, 3))
print("Task {} is processed by worker {}".format(task, self.name))
self.queue.task_done()
# 自定义消费策略函数
def custom_strategy():
# 实现自己的分配策略,这里简单地随机选择一个消费者进行分配
return random.randint(0, 9)
if __name__ == "__main__":
# 创建10个消费者队列
queues = [queue.Queue() for i in range(10)]
# 创建10个消费者线程并启动
for i in range(10):
worker = ConsumerThread(queues[i])
worker.start()
# 创建10个生产者线程并启动
for i in range(10):
producer = ProducerThread(queues, custom_strategy)
producer.start()
# 等待所有任务处理完成
for queue in queues:
queue.join()
```
这个代码实现了一个简单的多线程生产者消费者模型,其中生产者会根据自定义的消费策略函数将任务分配给对应的消费者进行处理。你可以根据自己的实际需求修改代码中的策略函数来实现不同的任务分配方式。注意:这个代码只是一个示例,实际场景中你需要根据实际情况进行修改和调整。
阅读全文
相关推荐
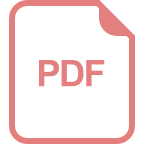

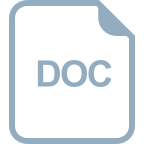
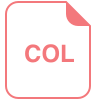
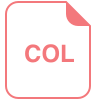
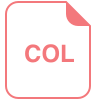
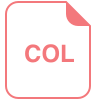
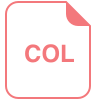
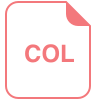
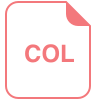
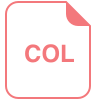
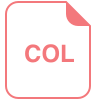
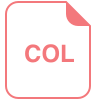
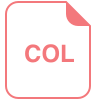
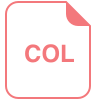
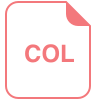
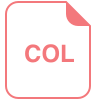