response.body为byte数组怎么处理
时间: 2024-03-08 22:48:44 浏览: 11
如果你想要将byte数组转换为字符串,可以使用以下方法:
```
String str = new String(response.body, StandardCharsets.UTF_8);
```
如果你想要将byte数组写入文件,可以使用以下方法:
```
try (OutputStream outputStream = new FileOutputStream("file.txt")) {
outputStream.write(response.body);
} catch (IOException e) {
e.printStackTrace();
}
```
如果你想要将byte数组转换为InputStream,可以使用以下方法:
```
InputStream inputStream = new ByteArrayInputStream(response.body);
```
相关问题
C#post请求中 传入数组类型的body 使用Newtonsoft.Json
可以使用下面的代码来实现C#中的POST请求传递数组类型的body,使用Newtonsoft.Json进行序列化和反序列化:
```csharp
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.IO;
using System.Net;
using System.Text;
public class Program
{
static void Main(string[] args)
{
// 定义POST请求的URL地址
string url = "http://example.com/api/test";
// 定义POST请求的Body参数,这里使用List<string>类型的数组
List<string> data = new List<string>()
{
"value1",
"value2",
"value3"
};
// 将List<string>类型的数组转换为JSON格式的字符串
string jsonData = JsonConvert.SerializeObject(data);
// 设置POST请求的Header参数
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "POST";
request.ContentType = "application/json";
// 将JSON格式的字符串转换为Byte数组
byte[] byteData = Encoding.UTF8.GetBytes(jsonData);
// 设置POST请求的Body参数
request.ContentLength = byteData.Length;
Stream requestStream = request.GetRequestStream();
requestStream.Write(byteData, 0, byteData.Length);
requestStream.Close();
// 发送POST请求并接收响应
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
Stream responseStream = response.GetResponseStream();
StreamReader reader = new StreamReader(responseStream);
string responseText = reader.ReadToEnd();
Console.WriteLine(responseText);
reader.Close();
responseStream.Close();
response.Close();
}
}
```
在上面的代码中,我们定义了一个List<string>类型的数组,然后使用Newtonsoft.Json将其转换为JSON格式的字符串。接着,我们设置了POST请求的Header参数,包括请求的方法、请求的Content-Type和请求的Body参数。最后,我们发送POST请求并接收响应,将响应输出到控制台上。
如何将将文件以字节数组(byte[])的方式写入到请求的 body 中发送请求示例代码
以下是一个使用Python和Requests库将文件以字节数组(byte[])的方式写入请求的body并发送请求的示例代码:
```python
import requests
# 1. 读取文件并转换为字节数组
file_path = 'path/to/your/file.txt'
with open(file_path, 'rb') as file:
file_bytes = file.read()
# 2. 发送请求
url = 'https://example.com/upload'
response = requests.post(url, data=file_bytes)
# 3. 处理响应...
print(response.text)
```
在上面的示例中,我们首先使用`open()`函数以二进制模式打开文件,并使用`read()`方法读取文件内容,将其存储为字节数组`file_bytes`。
然后,我们使用`requests.post()`方法发送POST请求,将文件字节数组作为请求的data参数传递给它。这将把文件内容作为请求体发送到指定的URL。
最后,我们打印出响应的内容`response.text`,您可以根据需要进行进一步处理。
请注意,上述代码仅为示例,具体的实现方式可能会因编程语言、框架和具体的应用场景而有所不同。请根据您的需求和环境进行相应的调整。
相关推荐
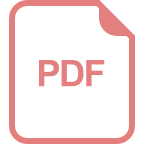
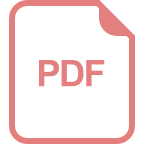
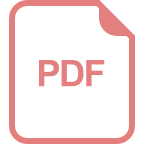












