springboot 获取contextpath
时间: 2023-05-08 17:58:47 浏览: 205
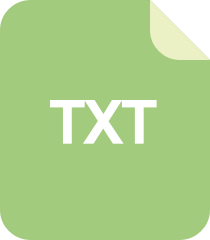
springboot 通过 ApplicationContextAware、ApplicationContext获取spring管理的bean-附件资源

在SpringBoot应用程序中,通过获取servletContext对象,可以轻松地获取contextPath。以下是在SpringBoot中获取contextPath的步骤:
1、在SpringBoot应用程序中,使用注解@Autowire注入ServletContext对象。
2、通过调用HttpServletRequest的getContextPath()方法,可以获取contextPath。
3、如果需要将contextPath值用于其他目的,可以将其存储在SpringBoot应用程序的任何范围内,例如web.xml或application.yml文件。
总之,通过以上步骤,我们可以轻松地在SpringBoot中获取contextPath,并进行任何必要的处理。由于SpringBoot提供了便捷的开发工具和优秀的API,因此在应用程序中获取contextPath是一项简单的任务。
阅读全文
相关推荐
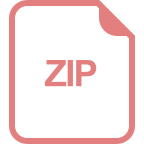



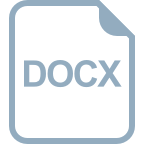
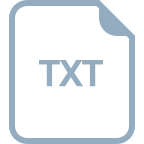
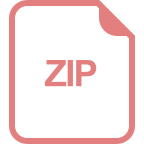









