使用人工鱼群算法对图像进行增强的原理是什么,同时附上python代码
时间: 2024-03-16 09:45:30 浏览: 20
人工鱼群算法是一种基于群体智能的优化算法,它模拟了鱼群觅食的行为,通过鱼群的协作和竞争来寻找最优解。在图像增强中,人工鱼群算法可以通过调整滤波器参数、图像灰度级别等方式来优化图像质量。
下面是使用Python实现人工鱼群算法进行图像增强的代码示例:
```python
import numpy as np
import cv2
# 定义人工鱼群算法的类
class ArtificialFishSwarm:
def __init__(self, img):
self.img = img # 待处理的图像
self.fish_num = 30 # 鱼群大小
self.visual_range = 20 # 可视范围
self.step_size = 5 # 步长
self.try_num = 5 # 尝试次数
self.delta_t = 0.1 # 时间间隔
self.feed_interval = 10 # 饥饿间隔
self.hunt_interval = 20 # 寻找猎物间隔
self.max_iter = 100 # 最大迭代次数
self.fish = [] # 鱼群
self.best_fish = None # 最优解
# 初始化鱼群
def init_fish(self):
h, w = self.img.shape
for i in range(self.fish_num):
x = np.random.randint(0, w)
y = np.random.randint(0, h)
self.fish.append({'x': x, 'y': y, 'fitness': -1})
# 计算适应度函数
def fitness(self, img):
return cv2.Laplacian(img, cv2.CV_64F).var()
# 移动鱼群
def move(self):
h, w = self.img.shape
for i in range(self.fish_num):
fish = self.fish[i]
max_fitness = fish['fitness']
best_x, best_y = fish['x'], fish['y']
for j in range(self.try_num):
x = fish['x'] + np.random.uniform(-self.step_size, self.step_size)
y = fish['y'] + np.random.uniform(-self.step_size, self.step_size)
if x < 0 or x >= w or y < 0 or y >= h:
continue
fitness = self.fitness(self.img[y:y+self.visual_range, x:x+self.visual_range])
if fitness > max_fitness:
max_fitness = fitness
best_x, best_y = x, y
fish['x'], fish['y'], fish['fitness'] = best_x, best_y, max_fitness
if max_fitness > self.best_fish['fitness']:
self.best_fish = fish
# 寻找猎物
def hunt(self):
for i in range(self.fish_num):
if i == self.best_fish:
continue
if np.random.uniform() < self.hunt_interval / self.max_iter:
self.fish[i]['x'], self.fish[i]['y'] = self.best_fish['x'], self.best_fish['y']
# 更新鱼群状态
def update(self):
for i in range(self.fish_num):
if np.random.uniform() < self.feed_interval / self.max_iter:
self.fish[i]['x'] = np.random.randint(0, self.img.shape[1])
self.fish[i]['y'] = np.random.randint(0, self.img.shape[0])
# 运行算法
def run(self):
self.init_fish()
self.best_fish = self.fish[0]
for i in range(self.max_iter):
self.move()
self.hunt()
self.update()
print('Iteration %d: fitness=%f' % (i+1, self.best_fish['fitness']))
return self.best_fish
# 测试
img = cv2.imread('test.jpg', cv2.IMREAD_GRAYSCALE)
afsa = ArtificialFishSwarm(img)
best_fish = afsa.run()
x, y = best_fish['x'], best_fish['y']
result = cv2.medianBlur(img[y:y+afsa.visual_range, x:x+afsa.visual_range], 5)
cv2.imshow('original', img)
cv2.imshow('result', result)
cv2.waitKey(0)
```
这段代码实现了人工鱼群算法对图像进行中值滤波增强的过程。算法通过调整滤波器的位置和半径来优化图像,最终得到一张更清晰的图像。
相关推荐
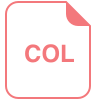













