verilog 调用函数
时间: 2024-12-04 09:15:08 浏览: 14
在Verilog硬件描述语言中,调用函数是通过`function`关键字来实现的。函数可以接受输入参数并返回结果,这对于模块间的交互非常有用。以下是使用Verilog编写函数的基本结构:
```verilog
// 定义一个名为add的函数,接受两个整数作为输入并返回它们的和
function [31:0] add(input integer a, input integer b);
output reg [31:0] result;
// 函数体内的操作
begin
result = a + b; // 对输入做加法操作
end
endfunction : add
// 在其他模块中调用该函数
module top_module (
input wire clk,
input wire rst,
output reg [31:0] sum
);
// 使用函数add
assign sum = add(10, 20); // 将10和20传递给add函数,并将结果赋值给sum
endmodule
```
相关问题
verilog 调用函数 寄存器输入
在Verilog语言中,函数调用是一种将函数的结果赋值给其他信号或者存储单元的操作。当涉及到寄存器输入时,函数通常用于处理数据的转换、计算或条件判断,并返回结果供后续操作。
例如,假设你有一个函数`process_data(input_data)`,这个函数接受一个输入寄存器的数据并对其进行某种处理。你可以像下面这样调用它:
```verilog
reg result_register; // 定义一个寄存器来保存函数结果
function logic processed_data;
input wire input_data;
// 函数体内的操作...
processed_data = some_logic_operation(input_data);
endfunction : process_data
always @(posedge clk) begin
if (condition) begin
processed_data = process_data(input_register); // 当满足某个条件时,调用函数并将结果赋给result_register
result_register <= processed_data; // 将函数结果写入寄存器
end
end
```
在这个例子中,`processed_data`是函数调用的结果,而`input_register`是一个寄存器,其内容作为函数的输入。函数返回的结果会被更新到`result_register`上,实现了对输入寄存器数据的处理。
system Verilog 调用C函数
SystemVerilog provides a DPI (Direct Programming Interface) mechanism that allows you to call C functions from your SystemVerilog code. This can be useful when you need to interface with external hardware or software components that are written in C.
Here are the steps to call a C function from SystemVerilog using DPI:
1. Define the C function in a header file. This header file should be included in both your C code and your SystemVerilog code.
```
// Example header file (example.h)
#ifndef EXAMPLE_H
#define EXAMPLE_H
int add(int a, int b);
#endif
```
2. Implement the C function in a C file. This file should be compiled into a shared library (DLL or .so) that can be loaded by your SystemVerilog simulator.
```
// Example C file (example.c)
#include "example.h"
int add(int a, int b) {
return a + b;
}
```
3. Load the shared library in your SystemVerilog code using the `import` keyword. This will allow you to call the C function from your SystemVerilog code.
```
// Example SystemVerilog code
import "DPI-C" function int add(int a, int b);
module top;
int result;
initial begin
result = add(1, 2); // Call the C function
$display("Result = %d", result);
end
endmodule
```
4. Compile and run your SystemVerilog code. Make sure that the shared library is in the library search path of your simulator. You may also need to set the `LD_LIBRARY_PATH` environment variable to specify the directory containing the shared library.
Note that DPI allows for passing arguments and returning values between C and SystemVerilog. You can also use the `extern` keyword in your C code to specify the linkage of your C function.
阅读全文
相关推荐
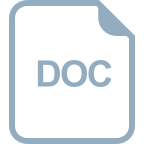
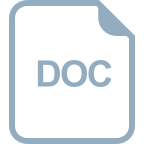
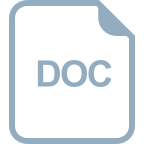

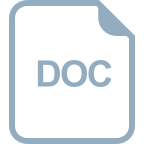
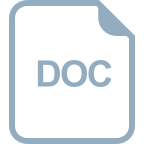










