dqn,pytorch
时间: 2023-08-18 18:15:08 浏览: 93
DQN (Deep Q-Network) 是一种强化学习算法,用于解决基于状态的马尔可夫决策过程(Markov Decision Process,MDP)问题。它结合了深度学习和Q-learning的思想,使用神经网络来近似估计Q值函数。PyTorch 是一个常用的深度学习框架,可以用于实现DQN算法。你可以使用PyTorch来构建神经网络模型,并使用DQN算法来训练模型以解决各种强化学习问题。
相关问题
DQN pytorch
DQN(Deep Q-Network)是一种基于深度学习的增强学习算法,用于解决强化学习中的决策问题。PyTorch是一个流行的深度学习框架,提供了构建深度神经网络的工具和库。在PyTorch中实现DQN涉及以下步骤:
1. 定义深度神经网络模型,通常使用卷积神经网络(CNN)。
2. 定义经验回放缓冲区,用于保存智能体与环境交互的经验。
3. 定义损失函数,通常使用均方误差(MSE)损失函数。
4. 定义优化器,通常使用随机梯度下降(SGD)或Adam优化器。
5. 定义智能体的行为策略,通常使用epsilon-greedy策略,其中epsilon表示探索概率。
6. 在每个时间步骤中,智能体根据当前状态选择一个动作,并与环境交互,获得下一个状态和奖励。
7. 将经验存储到经验回放缓冲区中。
8. 从经验回放缓冲区中抽取一小批经验,使用深度神经网络计算目标Q值和预测Q值,计算损失并进行反向传播。
9. 更新深度神经网络的参数。
10. 重复步骤6-9,直到智能体学会了最优策略或达到最大训练次数。
PyTorch提供了丰富的工具和库,使得实现DQN的过程相对简单。同时,PyTorch具有良好的可扩展性和灵活性,可以方便地扩展和调整DQN算法。
dqn pytorch代码
DQN(Deep Q-Network)是一种基于深度学习的强化学习算法,用于解决离散动作空间的问题。下面是一个简单的DQN PyTorch代码的介绍:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
# 定义DQN网络
class DQN(nn.Module):
def __init__(self, input_dim, output_dim):
super(DQN, self).__init__()
self.fc1 = nn.Linear(input_dim, 64)
self.fc2 = nn.Linear(64, 64)
self.fc3 = nn.Linear(64, output_dim)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = self.fc3(x)
return x
# 定义经验回放缓存
class ReplayBuffer():
def __init__(self, capacity):
self.capacity = capacity
self.buffer = []
self.position = 0
def push(self, state, action, reward, next_state, done):
if len(self.buffer) < self.capacity:
self.buffer.append(None)
self.buffer[self.position] = (state, action, reward, next_state, done)
self.position = (self.position + 1) % self.capacity
def sample(self, batch_size):
batch = np.random.choice(self.buffer, batch_size)
states, actions, rewards, next_states, dones = zip(*batch)
return np.array(states), np.array(actions), np.array(rewards), np.array(next_states), np.array(dones)
def __len__(self):
return len(self.buffer)
# 定义DQN Agent
class DQNAgent():
def __init__(self, input_dim, output_dim, lr, gamma, epsilon):
self.input_dim = input_dim
self.output_dim = output_dim
self.lr = lr
self.gamma = gamma
self.epsilon = epsilon
self.device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
self.model = DQN(input_dim, output_dim).to(self.device)
self.target_model = DQN(input_dim, output_dim).to(self.device)
self.target_model.load_state_dict(self.model.state_dict())
self.target_model.eval()
self.optimizer = optim.Adam(self.model.parameters(), lr=self.lr)
self.loss_fn = nn.MSELoss()
self.replay_buffer = ReplayBuffer(capacity=10000)
def select_action(self, state):
if np.random.rand() < self.epsilon:
return np.random.randint(self.output_dim)
state = torch.tensor(state, dtype=torch.float32).unsqueeze(0).to(self.device)
q_values = self.model(state)
return torch.argmax(q_values).item()
def train(self, batch_size):
if len(self.replay_buffer) < batch_size:
return
states, actions, rewards, next_states, dones = self.replay_buffer.sample(batch_size)
states = torch.tensor(states, dtype=torch.float32).to(self.device)
actions = torch.tensor(actions, dtype=torch.long).unsqueeze(1).to(self.device)
rewards = torch.tensor(rewards, dtype=torch.float32).unsqueeze(1).to(self.device)
next_states = torch.tensor(next_states, dtype=torch.float32).to(self.device)
dones = torch.tensor(dones, dtype=torch.float32).unsqueeze(1).to(self.device)
q_values = self.model(states).gather(1, actions)
next_q_values = self.target_model(next_states).max(1)[0].unsqueeze(1)
target_q_values = rewards + self.gamma * next_q_values * (1 - dones)
loss = self.loss_fn(q_values, target_q_values.detach())
self.optimizer.zero_grad()
loss.backward()
self.optimizer.step()
def update_target_model(self):
self.target_model.load_state_dict(self.model.state_dict())
def store_experience(self, state, action, reward, next_state, done):
self.replay_buffer.push(state, action, reward, next_state, done)
# 创建DQN Agent实例
input_dim = 4 # 输入维度
output_dim = 2 # 输出维度
lr = 0.001 # 学习率
gamma = 0.99 # 折扣因子
epsilon = 0.1 # 探索率
agent = DQNAgent(input_dim, output_dim, lr, gamma, epsilon)
# 训练DQN Agent
num_episodes = 1000
batch_size = 32
for episode in range(num_episodes):
state = env.reset()
done = False
total_reward = 0
while not done:
action = agent.select_action(state)
next_state, reward, done, _ = env.step(action)
agent.store_experience(state, action, reward, next_state, done)
agent.train(batch_size)
agent.update_target_model()
state = next_state
total_reward += reward
print(f"Episode: {episode+1}, Total Reward: {total_reward}")
# 使用训练好的DQN Agent进行预测
state = env.reset()
done = False
total_reward = 0
while not done:
action = agent.select_action(state)
next_state, reward, done, _ = env.step(action)
state = next_state
total_reward += reward
print(f"Total Reward: {total_reward}")
```
这段代码实现了一个简单的DQN Agent,包括DQN网络的定义、经验回放缓存的实现、Agent的训练和预测过程。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
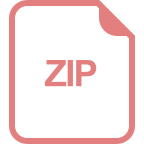
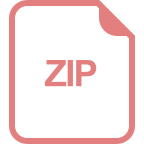
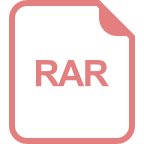
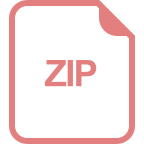




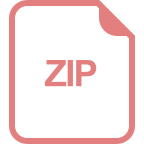
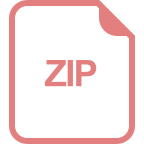




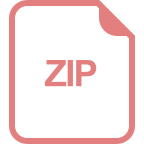