将array转化为torch
时间: 2023-08-15 19:05:08 浏览: 132
要将NumPy数组(array)转换为PyTorch张量(tensor),可以使用`torch.from_numpy()`函数。
以下是一个示例:
```python
import numpy as np
import torch
# 创建一个NumPy数组
array = np.array([[1, 2, 3], [4, 5, 6]])
# 将NumPy数组转换为PyTorch张量
tensor = torch.from_numpy(array)
# 打印结果
print(tensor)
```
在这个示例中,我们首先创建了一个NumPy数组`array`。然后,使用`torch.from_numpy()`函数将NumPy数组转换为PyTorch张量`tensor`。最后,我们打印出转换后的张量。
运行代码后,将会得到转换后的PyTorch张量。通过这种方式,你可以将NumPy数组转换为PyTorch张量,以便在PyTorch中进行深度学习和张量计算。
请注意,转换后的张量与原始数组共享相同的内存,因此对其中任意一个的修改会影响另一个。如果想要创建一个独立于原始数组的张量,可以使用`torch.tensor()`函数。
相关问题
image_data = image.pixel_array将image_data 转化为torch
要将`image_data`从PIL图像(像素数组)转换为PyTorch张量,可以按照以下步骤操作:
首先,确保`image_data`是PIL.Image对象的像素数组。然后,使用PyTorch的`torch.tensor()`函数将其转换为张量,考虑到图像通常以CHW(通道,高度,宽度)格式存储,而不是默认的HWC(高度,宽度,通道),所以还需要做一些预处理。
以下是相应的代码示例[^1]:
```python
# 假设image_data是PIL.Image对象的像素数组
img_tensor = torch.tensor(image_data, dtype=torch.float32) # 创建一个浮点32类型的张量
# 如果需要将HWC转换为CHW格式,按照源代码中的做法
if isinstance(image, PIL.Image.Image):
img_tensor = img_tensor.permute(2, 0, 1) # 将最后一个维度移动到前面
return img_tensor
```
这样就得到了一个适合PyTorch模型输入的张量。
将张量转化为torch张量方法
要将数据转换为PyTorch张量,你可以按照以下步骤进行:
1. **导入必要的库**[^2]:
```python
import torch
```
2. **数值数据转换**:
如果你已经有numpy数组或其他数值数据,可以使用`torch.from_numpy()`或`torch.tensor()`来转换:
```python
# 假设你有一个numpy数组
numpy_array = np.array([1, 2, 3, 4])
tensor_data = torch.from_numpy(numpy_array)
```
3. **字符串数据转换**:
字符串可以直接用`torch.tensor()`,但通常会先编码为数字表示:
```python
string = "Hello"
encoded_string = torch.tensor([ord(char) for char in string], dtype=torch.long)
```
4. **其他类型的数据转换**:
- 对于浮点数列表或元组,也可以直接传入`torch.tensor()`:
```python
float_list = [1.0, 2.0, 3.0]
tensor_floats = torch.tensor(float_list)
```
5. **创建随机张量**:
可以使用`torch.rand()`或`torch.randn()`生成随机张量:
```python
random_tensor = torch.rand(3, 3) # 创建3x3的随机浮点数张量
```
注意,在转换过程中,可以选择指定数据类型(如`dtype=torch.float32`),以及设备(CPU或GPU)。
阅读全文
相关推荐
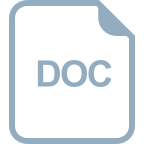
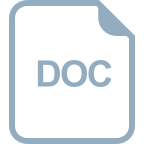
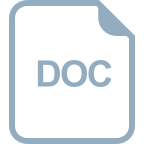













