np张量转化为torch张量
时间: 2024-06-16 14:06:16 浏览: 117
要将NumPy张量转换为PyTorch张量,可以使用`torch.from_numpy()`函数。这个函数接受一个NumPy数组作为输入,并返回一个对应的PyTorch张量。以下是一个示例代码:
```python
import numpy as np
import torch
# 创建一个NumPy数组
np_tensor = np.array([[1, 2, 3], [4, 5, 6]])
# 将NumPy数组转换为PyTorch张量
torch_tensor = torch.from_numpy(np_tensor)
print(torch_tensor)
```
输出结果为:
```
tensor([[1, 2, 3],
[4, 5, 6]])
```
需要注意的是,通过`torch.from_numpy()`函数创建的PyTorch张量与原始的NumPy数组共享内存,因此对其中一个进行修改会影响到另一个。如果需要在转换后的PyTorch张量上进行操作,建议使用`.clone()`方法创建一个独立的副本。
相关问题
将张量转化为torch张量方法
要将数据转换为PyTorch张量,你可以按照以下步骤进行:
1. **导入必要的库**[^2]:
```python
import torch
```
2. **数值数据转换**:
如果你已经有numpy数组或其他数值数据,可以使用`torch.from_numpy()`或`torch.tensor()`来转换:
```python
# 假设你有一个numpy数组
numpy_array = np.array([1, 2, 3, 4])
tensor_data = torch.from_numpy(numpy_array)
```
3. **字符串数据转换**:
字符串可以直接用`torch.tensor()`,但通常会先编码为数字表示:
```python
string = "Hello"
encoded_string = torch.tensor([ord(char) for char in string], dtype=torch.long)
```
4. **其他类型的数据转换**:
- 对于浮点数列表或元组,也可以直接传入`torch.tensor()`:
```python
float_list = [1.0, 2.0, 3.0]
tensor_floats = torch.tensor(float_list)
```
5. **创建随机张量**:
可以使用`torch.rand()`或`torch.randn()`生成随机张量:
```python
random_tensor = torch.rand(3, 3) # 创建3x3的随机浮点数张量
```
注意,在转换过程中,可以选择指定数据类型(如`dtype=torch.float32`),以及设备(CPU或GPU)。
tensorflow张量转换成torch张量
在Python中,要将TensorFlow张量转换为PyTorch张量,可以按照以下步骤操作[^1]:
1. 使用`tf.constant`创建一个TensorFlow张量:
```python
import tensorflow as tf
tf_tensor = tf.constant([1, 2, 3])
```
2. 使用`tf.Session().eval()`获取TensorFlow张量的numpy数组:
```python
with tf.compat.v1.Session().as_default():
np_array = tf_tensor.eval()
```
3. 利用`torch.from_numpy()`将numpy数组转换为PyTorch张量:
```python
import torch
torch_tensor = torch.from_numpy(np_array)
```
这样就完成了从TensorFlow到PyTorch的转换。
反之,如果有一个PyTorch张量,转换为TensorFlow的过程也相似:
```python
torch_tensor = torch.ones(100)
np_tensor = torch_tensor.numpy()
tf_tensor = tf.convert_to_tensor(np_tensor)
```
这里先使用`numpy()`函数将PyTorch张量转换为numpy数组,再通过`tf.convert_to_tensor()`转换为TensorFlow张量。
阅读全文
相关推荐
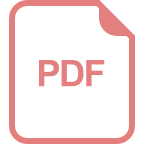
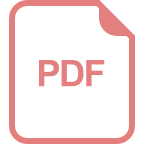
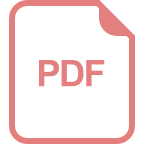













