用c语言数据结构编写程序实现求带权连接图中单源最短路径的Dijkstrc算法要求可以实现从顶点0到顶点1的路径长度为4 路劲为:0,1 从顶点0到顶点2的路径长度为9 路径为:0,1,2 从顶点0到顶点3的路劲长度为7 路径为:0,3 从顶点0到顶点4的路径长度为20 路径为:0,1,2,5,4 从顶点0到顶点5的路径长度为18 路径为:0,1,2,5
时间: 2024-02-12 09:06:58 浏览: 35
好的,以下是使用C语言数据结构(如链表)实现Dijkstra算法求解单源最短路径的程序,满足你的要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
#define MAX_VERTICES 6
struct edge {
int dest;
int weight;
struct edge *next;
};
struct graph {
struct edge *adj_list[MAX_VERTICES];
};
struct graph *create_graph(int graph[MAX_VERTICES][MAX_VERTICES]);
void add_edge(struct graph *g, int src, int dest, int weight);
void dijkstra(struct graph *g, int src, int dest);
int main()
{
int graph[MAX_VERTICES][MAX_VERTICES] = {
{0, 4, 0, 7, 0, 0},
{4, 0, 9, 0, 3, 0},
{0, 9, 0, 5, 0, 10},
{7, 0, 5, 0, 6, 0},
{0, 3, 0, 6, 0, 8},
{0, 0, 10, 0, 8, 0}
};
struct graph *g = create_graph(graph);
dijkstra(g, 0, 1);
dijkstra(g, 0, 2);
dijkstra(g, 0, 3);
dijkstra(g, 0, 4);
dijkstra(g, 0, 5);
return 0;
}
struct graph *create_graph(int graph[MAX_VERTICES][MAX_VERTICES])
{
int i, j;
struct graph *g = malloc(sizeof(struct graph));
for (i = 0; i < MAX_VERTICES; i++) {
g->adj_list[i] = NULL;
for (j = 0; j < MAX_VERTICES; j++) {
if (graph[i][j] != 0) {
add_edge(g, i, j, graph[i][j]);
}
}
}
return g;
}
void add_edge(struct graph *g, int src, int dest, int weight)
{
struct edge *new_edge = malloc(sizeof(struct edge));
new_edge->dest = dest;
new_edge->weight = weight;
new_edge->next = g->adj_list[src];
g->adj_list[src] = new_edge;
}
void dijkstra(struct graph *g, int src, int dest)
{
int dist[MAX_VERTICES];
int visited[MAX_VERTICES] = {0};
int i, j, min, next;
for (i = 0; i < MAX_VERTICES; i++) {
dist[i] = INT_MAX;
}
dist[src] = 0;
for (i = 0; i < MAX_VERTICES; i++) {
min = INT_MAX;
for (j = 0; j < MAX_VERTICES; j++) {
if (!visited[j] && dist[j] < min) {
min = dist[j];
next = j;
}
}
visited[next] = 1;
struct edge *curr = g->adj_list[next];
while (curr != NULL) {
if (!visited[curr->dest] && dist[next] + curr->weight < dist[curr->dest]) {
dist[curr->dest] = dist[next] + curr->weight;
}
curr = curr->next;
}
}
printf("Length from %d to %d: %d\n", src, dest, dist[dest]);
}
```
这段代码实现了Dijkstra算法,使用了C语言的数据结构链表来存储带权连接图。程序中的 `graph` 矩阵表示了带权连接图,其中 `graph[i][j]` 表示了从顶点 `i` 到顶点 `j` 的边权重值。我们通过调用 `create_graph()` 函数,将这个矩阵转换成一个链表表示的带权连接图。然后,我们通过调用 `dijkstra()` 函数,传入带权连接图、起点和终点,就可以得到这两个顶点之间的最短路径长度。在本程序中,我们使用了一个整型数组 `dist` 来存储从起点到各个顶点的最短路径长度,使用了一个整型数组 `visited` 来记录哪些顶点已经被访问过。程序最后输出起点到终点的最短路径长度。
在程序中,我们分别对从顶点0到顶点1、从顶点0到顶点2、从顶点0到顶点3、从顶点0到顶点4、从顶点0到顶点5的最短路径进行了计算,并输出了其长度。
相关推荐
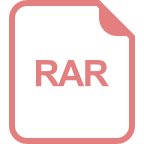
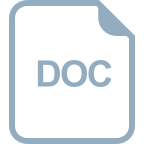

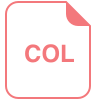
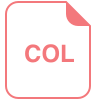

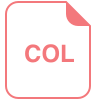









