用Java实现扫雷游戏
时间: 2023-12-19 16:05:29 浏览: 81
好的,下面是一个简单的Java实现扫雷游戏的代码,供参考:
```
import java.util.*;
public class Minesweeper {
private int[][] board;
private boolean[][] revealed;
private boolean[][] flagged;
private int numMines;
private int numRows;
private int numCols;
private int numRevealed;
public Minesweeper(int numRows, int numCols, int numMines) {
this.numRows = numRows;
this.numCols = numCols;
this.numMines = numMines;
this.numRevealed = 0;
this.board = new int[numRows][numCols];
this.revealed = new boolean[numRows][numCols];
this.flagged = new boolean[numRows][numCols];
initializeBoard();
}
private void initializeBoard() {
Random rand = new Random();
int minesPlaced = 0;
while (minesPlaced < numMines) {
int row = rand.nextInt(numRows);
int col = rand.nextInt(numCols);
if (board[row][col] == 0) {
board[row][col] = -1;
minesPlaced++;
}
}
for (int row = 0; row < numRows; row++) {
for (int col = 0; col < numCols; col++) {
if (board[row][col] == -1) {
updateAdjacentCells(row, col);
}
}
}
}
private void updateAdjacentCells(int row, int col) {
for (int i = row - 1; i <= row + 1; i++) {
for (int j = col - 1; j <= col + 1; j++) {
if (i >= 0 && i < numRows && j >= 0 && j < numCols && board[i][j] != -1) {
board[i][j]++;
}
}
}
}
public void printBoard() {
System.out.println("\n");
for (int row = 0; row < numRows; row++) {
for (int col = 0; col < numCols; col++) {
if (revealed[row][col]) {
if (board[row][col] == -1) {
System.out.print("* ");
} else {
System.out.print(board[row][col] + " ");
}
} else if (flagged[row][col]) {
System.out.print("F ");
} else {
System.out.print("- ");
}
}
System.out.println();
}
}
public boolean isGameWon() {
return numRevealed == numRows * numCols - numMines;
}
public boolean isGameLost() {
for (int row = 0; row < numRows; row++) {
for (int col = 0; col < numCols; col++) {
if (revealed[row][col] && board[row][col] == -1) {
return true;
}
}
}
return false;
}
public boolean isCellRevealed(int row, int col) {
return revealed[row][col];
}
public boolean isCellFlagged(int row, int col) {
return flagged[row][col];
}
public boolean isCellMine(int row, int col) {
return board[row][col] == -1;
}
public void revealCell(int row, int col) {
if (!revealed[row][col] && !flagged[row][col]) {
revealed[row][col] = true;
numRevealed++;
if (board[row][col] == 0) {
revealAdjacentCells(row, col);
}
}
}
private void revealAdjacentCells(int row, int col) {
for (int i = row - 1; i <= row + 1; i++) {
for (int j = col - 1; j <= col + 1; j++) {
if (i >= 0 && i < numRows && j >= 0 && j < numCols && !revealed[i][j]) {
revealCell(i, j);
}
}
}
}
public void flagCell(int row, int col) {
if (!revealed[row][col]) {
flagged[row][col] = !flagged[row][col];
}
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter number of rows: ");
int numRows = sc.nextInt();
System.out.print("Enter number of columns: ");
int numCols = sc.nextInt();
System.out.print("Enter number of mines: ");
int numMines = sc.nextInt();
Minesweeper game = new Minesweeper(numRows, numCols, numMines);
while (!game.isGameWon() && !game.isGameLost()) {
game.printBoard();
System.out.print("Enter row to reveal: ");
int row = sc.nextInt();
System.out.print("Enter column to reveal: ");
int col = sc.nextInt();
if (game.isCellMine(row, col)) {
System.out.println("Game over!");
game.revealCell(row, col);
} else {
game.revealCell(row, col);
if (game.isGameWon()) {
System.out.println("You win!");
}
}
System.out.print("Do you want to flag a cell? (Y/N) ");
String response = sc.next();
if (response.equalsIgnoreCase("Y")) {
System.out.print("Enter row to flag: ");
row = sc.nextInt();
System.out.print("Enter column to flag: ");
col = sc.nextInt();
game.flagCell(row, col);
}
}
game.printBoard();
}
}
```
这个实现中,我们使用了一个二维数组来表示扫雷游戏的棋盘,其中包含了每个格子周围的地雷数量。我们还使用了两个布尔类型的二维数组来表示每个格子是否已经被揭示或标记。在程序的主循环中,玩家可以选择一个格子来揭示它,或者标记一个格子为地雷。如果揭示的格子是地雷,游戏将结束。否则,游戏将继续进行,直到玩家揭示所有非地雷格子或揭示了一个地雷。
阅读全文
相关推荐
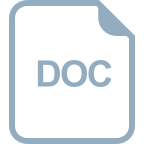
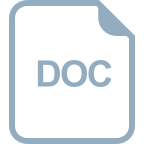
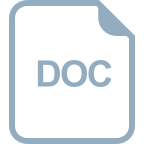
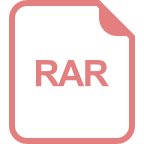
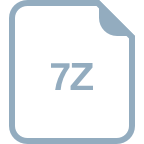

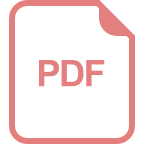
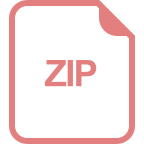
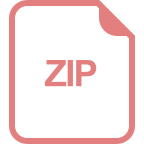


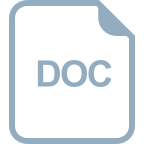
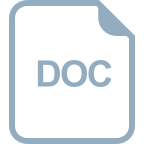
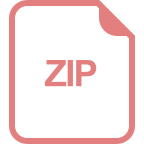
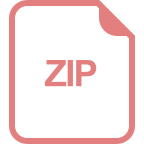
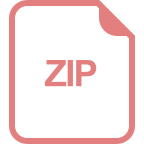
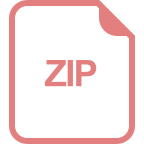