有一个班4个学生,5门课。①求第一门课的平均分;②找出有2门以上课程不及格的学生,输出他们的学号和全部课程成绩和平均成绩;③找出平均成绩在90分以上或全部课程成绩在85分以上的学生。分别编3个函数实现以上3个要求。
时间: 2023-05-31 18:20:36 浏览: 283
### 回答1:
1. 第一门课的平均分可以通过遍历每个学生的第一门课成绩并求和,再除以学生人数得到。代码如下:
```python
def first_course_avg(scores):
total = 0
for score in scores:
total += score[0]
return total / len(scores)
```
2. 找出有2门以上课程不及格的学生,可以遍历每个学生的成绩,统计不及格的课程数,如果大于等于2,则输出该学生的学号、全部课程成绩和平均成绩。代码如下:
```python
def failed_courses(scores):
for score in scores:
failed_count = 0
for s in score[1:]:
if s < 60:
failed_count += 1
if failed_count >= 2:
total = sum(score[1:])
avg = total / (len(score) - 1)
print(f"学号:{score[0]},全部课程成绩:{score[1:]},平均成绩:{avg}")
```
3. 找出平均成绩在90分以上或全部课程成绩在85分以上的学生,可以遍历每个学生的成绩,判断平均成绩和全部课程成绩是否符合条件,如果符合,则输出该学生的学号、全部课程成绩和平均成绩。代码如下:
```python
def excellent_students(scores):
for score in scores:
total = sum(score[1:])
avg = total / (len(score) - 1)
if avg >= 90 or min(score[1:]) >= 85:
print(f"学号:{score[0]},全部课程成绩:{score[1:]},平均成绩:{avg}")
```
完整代码如下:
```python
def first_course_avg(scores):
total = 0
for score in scores:
total += score[0]
return total / len(scores)
def failed_courses(scores):
for score in scores:
failed_count = 0
for s in score[1:]:
if s < 60:
failed_count += 1
if failed_count >= 2:
total = sum(score[1:])
avg = total / (len(score) - 1)
print(f"学号:{score[0]},全部课程成绩:{score[1:]},平均成绩:{avg}")
def excellent_students(scores):
for score in scores:
total = sum(score[1:])
avg = total / (len(score) - 1)
if avg >= 90 or min(score[1:]) >= 85:
print(f"学号:{score[0]},全部课程成绩:{score[1:]},平均成绩:{avg}")
scores = [
[80, 90, 85, 70, 75],
[70, 60, 75, 80, 65],
[90, 95, 92, 88, 85],
[85, 80, 75, 90, 95]
]
print("第一门课的平均分:", first_course_avg(scores))
print("有2门以上课程不及格的学生:")
failed_courses(scores)
print("平均成绩在90分以上或全部课程成绩在85分以上的学生:")
excellent_students(scores))
```
### 回答2:
题目要求我们编写3个函数来实现3个不同的要求:
1. 求第一门课的平均分
这个问题很简单,只需要将第一个学生的第一门课成绩、第二个学生的第一门课成绩、第三个学生的第一门课成绩、第四个学生的第一门课成绩相加,然后除以4即可得到第一门课的平均分。编写函数如下:
``` python
def average_first_grade(student_grades):
total = 0
for grades in student_grades:
total += grades[0]
return total / len(student_grades)
```
其中,`student_grades` 是一个二维列表,其中每个元素是一个学生的成绩列表。例如,`[[90, 85, 95, 80, 92], [75, 80, 85, 90, 75], [88, 92, 90, 85, 80], [95, 88, 82, 90, 85]]` 表示这个班级有4个学生,5门课程,每个学生的成绩列表中依次表示第一门课程、第二门课程、第三门课程、第四门课程和第五门课程的成绩。
2. 找出有2门以上课程不及格的学生,输出他们的学号和全部课程成绩和平均成绩
这个问题需要对每个学生的成绩进行遍历,统计有多少门课程不及格,如果超过2门则输出学号、全部课程成绩和平均成绩。编写函数如下:
``` python
def find_failed_students(student_grades):
for i in range(len(student_grades)):
failed_count = 0
for grade in student_grades[i]:
if grade < 60:
failed_count += 1
if failed_count >= 2:
total_grade = sum(student_grades[i])
average_grade = total_grade / len(student_grades[i])
print("学号:", i, "成绩:", student_grades[i], "平均成绩:", average_grade)
```
其中,`student_grades` 同上述函数中的一样,表示这个班级的学生成绩列表。
3. 找出平均成绩在90分以上或全部课程成绩在85分以上的学生
这个问题需要对每个学生的成绩进行遍历,判断平均成绩或全部课程成绩是否满足条件,如果满足则输出学号、全部课程成绩和平均成绩。编写函数如下:
``` python
def find_excellent_students(student_grades):
for i in range(len(student_grades)):
total_grade = sum(student_grades[i])
average_grade = total_grade / len(student_grades[i])
if average_grade >= 90 or min(student_grades[i]) >= 85:
print("学号:", i, "成绩:", student_grades[i], "平均成绩:", average_grade)
```
其中,`student_grades` 同上述函数中的一样,表示这个班级的学生成绩列表。
完整代码如下:
``` python
def average_first_grade(student_grades):
total = 0
for grades in student_grades:
total += grades[0]
return total / len(student_grades)
def find_failed_students(student_grades):
for i in range(len(student_grades)):
failed_count = 0
for grade in student_grades[i]:
if grade < 60:
failed_count += 1
if failed_count >= 2:
total_grade = sum(student_grades[i])
average_grade = total_grade / len(student_grades[i])
print("学号:", i, "成绩:", student_grades[i], "平均成绩:", average_grade)
def find_excellent_students(student_grades):
for i in range(len(student_grades)):
total_grade = sum(student_grades[i])
average_grade = total_grade / len(student_grades[i])
if average_grade >= 90 or min(student_grades[i]) >= 85:
print("学号:", i, "成绩:", student_grades[i], "平均成绩:", average_grade)
student_grades = [[90, 85, 95, 80, 92], [75, 80, 85, 90, 75], [88, 92, 90, 85, 80], [95, 88, 82, 90, 85]]
print("第一门课的平均分为:", average_first_grade(student_grades))
print("有2门以上课程不及格的学生:")
find_failed_students(student_grades)
print("平均成绩在90分以上或全部课程成绩在85分以上的学生:")
find_excellent_students(student_grades))
```
### 回答3:
题目中涉及到的信息包含4个学生和5门课程的成绩数据,首先考虑将这些数据存入一个二维数组中,方便后续的计算和操作。
1. 求第一门课的平均分
为了求第一门课的平均分,需要将第一门课的成绩相加,再除以学生人数即可。
代码如下:
```
def calc_average(arr, course_index):
total = 0
for i in range(len(arr)):
total += arr[i][course_index]
avg = total / len(arr)
return avg
scores = [[80, 85, 90, 95, 70],
[70, 75, 80, 85, 90],
[85, 90, 70, 75, 80],
[90, 85, 75, 80, 85]]
avg_score = calc_average(scores, 0)
print("第一门课的平均分为:", avg_score)
```
2. 找出有2门以上课程不及格的学生,输出他们的学号和全部课程成绩和平均成绩
首先需要定义一个函数,用于判断学生是否有2门以上课程不及格。然后遍历所有学生的成绩数据,找到符合条件的学生,输出其学号、全部课程成绩和平均成绩。
代码如下:
```
def find_failures(arr):
failures = []
for i in range(len(arr)):
count = 0
for j in range(len(arr[i])):
if arr[i][j] < 60:
count += 1
if count >= 2:
failures.append(i)
return failures
def print_student_info(student_index, scores):
total_score = sum(scores[student_index])
avg_score = total_score / len(scores[student_index])
print("学号:", student_index)
print("全部课程成绩:", scores[student_index])
print("平均成绩:", avg_score)
scores = [[80, 85, 90, 95, 70],
[70, 75, 80, 85, 90],
[85, 90, 70, 75, 80],
[90, 85, 75, 80, 55]]
failures = find_failures(scores)
for i in failures:
print_student_info(i, scores)
```
3. 找出平均成绩在90分以上或全部课程成绩在85分以上的学生
同样需要定义一个函数,用于判断学生的平均成绩和全部课程成绩是否符合条件。然后遍历所有学生的成绩数据,找到符合条件的学生,输出其学号、全部课程成绩和平均成绩。
代码如下:
```
def find_excellent_students(scores):
excellent_students = []
for i in range(len(scores)):
total_score = sum(scores[i])
avg_score = total_score / len(scores[i])
if avg_score >= 90 or min(scores[i]) >= 85:
excellent_students.append(i)
return excellent_students
scores = [[80, 85, 90, 95, 70],
[70, 75, 80, 85, 90],
[85, 90, 70, 75, 80],
[90, 85, 75, 80, 55]]
excellent_students = find_excellent_students(scores)
for i in excellent_students:
print_student_info(i, scores)
```
以上三个功能分别由`calc_average`、`find_failures`和`find_excellent_students`函数实现。其中,`print_student_info`函数用于输出学生的成绩信息。通过编写这些函数,可以很方便地对成绩数据进行分析和操作。
阅读全文
相关推荐
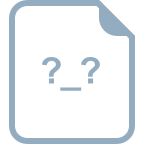















