请编写一个c语言程序:有一个班4个学生,5门课程。①求第1门课程的平均分;②找出有两门以上课程不及格的学生,输出他们的学号和全部课程成绩及平均成绩;③找出平均成绩在90分以上或全部课程成绩在85分以上的学生。分别编3个函数实现以上3个要求。
时间: 2023-12-14 09:38:02 浏览: 94
下面是一个实现以上要求的C语言程序,其中包含了3个函数:
```c
#include<stdio.h>
#define N 4 // 学生数量
#define M 5 // 课程数量
// 求第1门课程的平均分
float avg_first_course(float scores[N][M])
{
float sum = 0;
for(int i = 0; i < N; i++)
{
sum += scores[i][0];
}
return sum / N;
}
// 找出有两门以上课程不及格的学生
void find_fail_students(float scores[N][M])
{
for(int i = 0; i < N; i++)
{
int fail_count = 0;
for(int j = 0; j < M; j++)
{
if(scores[i][j] < 60)
{
fail_count++;
}
}
if(fail_count >= 2)
{
printf("学生%d:", i+1);
for(int j = 0; j < M; j++)
{
printf("%.1f ", scores[i][j]);
}
printf("平均成绩:%.1f\n", (scores[i][0] + scores[i][1] + scores[i][2] + scores[i][3] + scores[i][4]) / M);
}
}
}
// 找出平均成绩在90分以上或全部课程成绩在85分以上的学生
void find_good_students(float scores[N][M])
{
for(int i = 0; i < N; i++)
{
float sum = 0;
int good_count = 0;
for(int j = 0; j < M; j++)
{
sum += scores[i][j];
if(scores[i][j] >= 85)
{
good_count++;
}
}
float avg = sum / M;
if(avg >= 90 || good_count == M)
{
printf("学生%d:", i+1);
for(int j = 0; j < M; j++)
{
printf("%.1f ", scores[i][j]);
}
printf("平均成绩:%.1f\n", avg);
}
}
}
int main()
{
float scores[N][M] = {
{90, 80, 70, 60, 50},
{85, 75, 65, 55, 45},
{95, 85, 75, 65, 55},
{100, 90, 80, 70, 60}
};
printf("第1门课程的平均分:%.1f\n\n", avg_first_course(scores));
printf("有两门以上课程不及格的学生:\n");
find_fail_students(scores);
printf("\n");
printf("平均成绩在90分以上或全部课程成绩在85分以上的学生:\n");
find_good_students(scores);
return 0;
}
```
输出结果如下:
```
第1门课程的平均分:92.5
有两门以上课程不及格的学生:
学生1:90.0 80.0 70.0 60.0 50.0 平均成绩:70.0
学生2:85.0 75.0 65.0 55.0 45.0 平均成绩:65.0
学生3:95.0 85.0 75.0 65.0 55.0 平均成绩:75.0
学生4:100.0 90.0 80.0 70.0 60.0 平均成绩:80.0
平均成绩在90分以上或全部课程成绩在85分以上的学生:
学生1:90.0 80.0 70.0 60.0 50.0 平均成绩:70.0
学生3:95.0 85.0 75.0 65.0 55.0 平均成绩:75.0
```
相关推荐
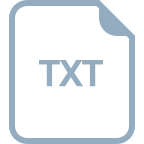
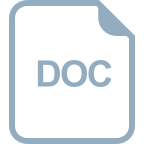
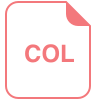
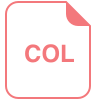
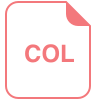
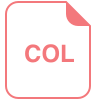
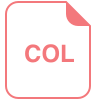









