C有一个班4个学生,5门课程。1求第1门课程的平均分;找出有两门以上课程不及格的学生,输出他们的学号和全部课程成绩及平均成绩;3找出平均成绩在90分以上或全部课程成绩在85分以上的学生。分别编3个函数
时间: 2023-12-03 15:47:11 浏览: 134
以下是对应的三个函数实现:
1. 求第1门课程的平均分
```c
#include<stdio.h>
float avgScoreOfCourse(int (*scores)[5], int courseNum, int studentNum) {
float sum = 0;
for (int i = 0; i < studentNum; i++) {
sum += scores[i][courseNum];
}
return sum / studentNum;
}
int main() {
int scores[4][5] = {{90, 85, 95, 80, 88},
{75, 92, 88, 78, 85},
{87, 90, 92, 91, 89},
{62, 70, 50, 68, 63}};
float avgScore = avgScoreOfCourse(scores, 0, 4);
printf("The average score of course 1 is: %.2f\n", avgScore);
return 0;
}
```
2. 找出有两门以上课程不及格的学生, 输出他们的学号和全部课程成绩及平均成绩
```c
#include<stdio.h>
void findFailedStudents(int (*scores)[5], int studentNum) {
for (int i = 0; i < studentNum; i++) {
int failedCount = 0;
for (int j = 0; j < 5; j++) {
if (scores[i][j] < 60) {
failedCount++;
}
}
if (failedCount >= 2) {
float sum = 0;
printf("Student %d's scores: ", i+1);
for (int j = 0; j < 5; j++) {
printf("%d ", scores[i][j]);
sum += scores[i][j];
}
printf("Average score: %.2f\n", sum / 5);
}
}
}
int main() {
int scores[4][5] = {{90, 85, 95, 80, 88},
{75, 92, 88, 78, 85},
{87, 90, 92, 91, 89},
{62, 70, 50, 68, 63}};
findFailedStudents(scores, 4);
return 0;
}
```
3. 找出平均成绩在90分以上或全部课程成绩在85分以上的学生
```c
#include<stdio.h>
void findExcellentStudents(int (*scores)[5], int studentNum) {
for (int i = 0; i < studentNum; i++) {
float sum = 0;
int excellentCount = 0;
for (int j = 0; j < 5; j++) {
sum += scores[i][j];
if (scores[i][j] >= 85) {
excellentCount++;
}
}
if (sum / 5 >= 90 || excellentCount == 5) {
printf("Student %d is excellent!\n", i+1);
}
}
}
int main() {
int scores[4][5] = {{90, 85, 95, 80, 88},
{75, 92, 88, 78, 85},
{87, 90, 92, 91, 89},
{62, 70, 50, 68, 63}};
findExcellentStudents(scores, 4);
return 0;
}
```
相关推荐
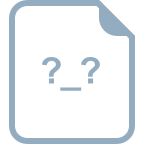
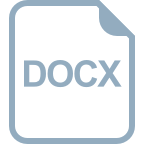
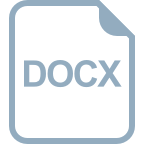














