1. 求第一门课程的平均分; 2. 找出有两门以上课程不及格的学生,输出他们的学号和全部课程成绩及平均成绩; 3. 找出平均成绩在90分以上或全部课程成绩在85分以上的学生。分别编三个函数实现以上三个要求。用c语言实验 运用结构体类型
时间: 2023-10-26 15:06:25 浏览: 144
对于您的问题,我将分别进行回答:
1. 求第一门课程的平均分
这是一个求某门课程平均分的问题,假设您已经用结构体类型定义了一些学生的信息(包括学号、姓名和课程成绩)。
可以使用以下代码来实现求第一门课程的平均分:
```c
float avg_score(struct student stu[], int n) {
float sum = 0.0;
for(int i = 0; i < n; i++) {
sum += stu[i].score[0];
}
return sum / n;
}
```
其中,`struct student` 代表学生的结构体类型,`stu[]` 是包含学生信息的数组,`n` 是数组的长度。`stu[i].score[0]` 表示学生数组中第 i 个学生的第一门课程成绩,这里假设第一门课程的成绩在数组中的序号为 0。
2. 找出有两门以上课程不及格的学生,输出他们的学号和全部课程成绩及平均成绩
这是一个找出不及格学生的问题,可以使用以下代码实现:
```c
void find_failed_students(struct student stu[], int n) {
int count; // 记录不及格科目的数量
float avg; // 记录平均成绩
for(int i = 0; i < n; i++) {
count = 0;
for(int j = 0; j < 5; j++) { // 假设学生有五门课程
if(stu[i].score[j] < 60) {
count++;
}
}
if(count >= 2) {
printf("学号:%d,姓名:%s,全部课程成绩:", stu[i].id, stu[i].name);
for(int j = 0; j < 5; j++) { // 假设学生有五门课程
printf("%.1f, ", stu[i].score[j]);
}
avg = (stu[i].score[0] + stu[i].score[1] + stu[i].score[2] + stu[i].score[3] + stu[i].score[4]) / 5.0;
printf("平均成绩:%.2f\n", avg);
}
}
}
```
其中,`struct student` 代表学生的结构体类型,`stu[]` 是包含学生信息的数组,`n` 是数组的长度。`stu[i].score[j]` 表示学生数组中第 i 个学生的第 j 门课程成绩,这里假设学生有五门课程。如果某个学生有两门或两门以上不及格的科目,那么就输出该学生的信息(学号、姓名、全部课程成绩和平均成绩)。
3. 找出平均成绩在90分以上或全部课程成绩在85分以上的学生
这是一个找出高成绩学生的问题,可以使用以下代码实现:
```c
void find_high_score_students(struct student stu[], int n) {
float avg; // 记录平均成绩
printf("平均成绩在90分以上的学生:\n");
for(int i = 0; i < n; i++) {
avg = (stu[i].score[0] + stu[i].score[1] + stu[i].score[2] + stu[i].score[3] + stu[i].score[4]) / 5.0;
if(avg >= 90.0) {
printf("学号:%d,姓名:%s,平均成绩:%.2f\n", stu[i].id, stu[i].name, avg);
}
}
printf("全部课程成绩在85分以上的学生:\n");
for(int i = 0; i < n; i++) {
if(stu[i].score[0] >= 85 && stu[i].score[1] >= 85 && stu[i].score[2] >= 85 && stu[i].score[3] >= 85 && stu[i].score[4] >= 85) {
printf("学号:%d,姓名:%s,全部课程成绩:", stu[i].id, stu[i].name);
for(int j = 0; j < 5; j++) { // 假设学生有五门课程
printf("%.1f, ", stu[i].score[j]);
}
printf("平均成绩:%.2f\n", avg);
}
}
}
```
其中,`struct student` 代表学生的结构体类型,`stu[]` 是包含学生信息的数组,`n` 是数组的长度。`stu[i].score[j]` 表示学生数组中第 i 个学生的第 j 门课程成绩,这里假设学生有五门课程。如果某个学生的平均成绩在90分以上或者所有课程成绩都在85分以上,那么就输出该学生的信息(学号、姓名、全部课程成绩和平均成绩)。
阅读全文
相关推荐

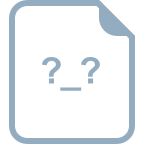













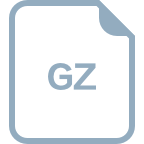