c语言输出二叉树先序遍历结果的第k个结点
时间: 2023-12-14 15:03:32 浏览: 43
以下是输出二叉树先序遍历结果的第k个结点的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
void preOrder(struct TreeNode* root, int* count, int k, struct TreeNode** result) {
if (root == NULL) {
return;
}
(*count)++;
if (*count == k) {
*result = root;
return;
}
preOrder(root->left, count, k, result);
preOrder(root->right, count, k, result);
}
struct TreeNode* getKthNode(struct TreeNode* root, int k) {
if (root == NULL) {
return NULL;
}
int count = 0;
struct TreeNode* result = NULL;
preOrder(root, &count, k, &result);
return result;
}
int main() {
struct TreeNode* root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = 1;
root->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->left->val = 2;
root->left->left = NULL;
root->left->right = NULL;
root->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->val = 3;
root->right->left = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->left->val = 4;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->right->right->val = 5;
root->right->right->left = NULL;
root->right->right->right = NULL;
int k = 4;
struct TreeNode* result = getKthNode(root, k);
if (result != NULL) {
printf("The %dth node of the binary tree is %d.\n", k, result->val);
} else {
printf("The binary tree doesn't have %d nodes.\n", k);
}
return 0;
}
```
在这个例子中,我们首先定义了一个二叉树结构体`struct TreeNode`,其中包含一个整型变量`val`表示结点的值,以及两个指向左右子结点的指针`left`和`right`。我们接着定义了一个函数`preOrder`,用于实现先序遍历,并通过参数`count`记录已经遍历的结点个数,若已经遍历的结点个数等于目标结点的序号$k$,则将该结点的指针存入`result`中。最终,我们定义了一个函数`getKthNode`,用于调用`preOrder`函数获取二叉树先序遍历结果的第$k$个结点。最后,我们通过一个例子进行测试,输出二叉树先序遍历结果的第$k$个结点的值。
相关推荐
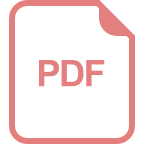
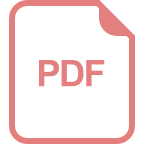
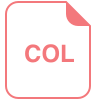
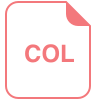
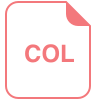
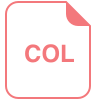
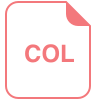









