c语言,按先序建立二叉树,然后输出先序遍历结果的第n个结点值,代码思路
时间: 2024-05-05 14:20:07 浏览: 11
首先,我们需要定义二叉树的结构体,每个节点包含左右子树指针以及它自己的值。
```C
typedef struct node {
int value;
struct node *left;
struct node *right;
} Node;
```
然后,我们需要编写一个递归的函数来建立二叉树。
```C
Node *create_tree() {
char c;
Node *node;
scanf("%c", &c);
if (c == ' ') {
node = NULL;
} else {
node = (Node *)malloc(sizeof(Node));
node->value = c - '0'; // 假设输入的节点值都是数字字符
node->left = create_tree();
node->right = create_tree();
}
return node;
}
```
接下来,我们可以编写一个函数来输出先序遍历结果的第n个节点值。
```C
void pre_order(Node *node, int n, int *count, int *result) {
if (node == NULL || *count >= n) {
return;
}
(*count)++;
if (*count == n) {
*result = node->value;
return;
}
pre_order(node->left, n, count, result);
pre_order(node->right, n, count, result);
}
```
最后,我们需要在主函数中调用这些函数,并输入二叉树的先序遍历结果和n的值。
```C
int main() {
Node *root = create_tree();
int n;
scanf("%d", &n);
int count = 0;
int result;
pre_order(root, n, &count, &result);
printf("%d\n", result);
return 0;
}
```
这样,我们就完成了这个问题的代码实现。
相关推荐
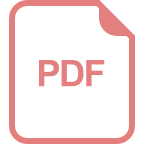
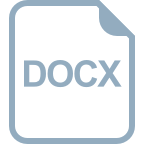
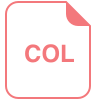
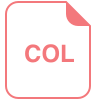
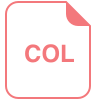
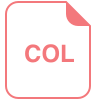










