编写二叉树的递归遍历算法,给定二叉树的扩展先序遍历序列,输出二叉树的先序遍历,中序遍历和后序遍历的结点序列和叶子结点和和结点个数的c语言代码
时间: 2024-05-14 08:19:17 浏览: 13
以下是对应的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 创建一棵二叉树
TreeNode* createTree(int* pos) {
char* s = "AB##C##";
char ch = s[(*pos)++];
if (ch == '#') {
return NULL;
}
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = ch;
root->left = createTree(pos);
root->right = createTree(pos);
return root;
}
// 先序遍历
void preOrder(TreeNode* root) {
if (root == NULL) {
return;
}
printf("%c ", root->val);
preOrder(root->left);
preOrder(root->right);
}
// 中序遍历
void inOrder(TreeNode* root) {
if (root == NULL) {
return;
}
inOrder(root->left);
printf("%c ", root->val);
inOrder(root->right);
}
// 后序遍历
void postOrder(TreeNode* root) {
if (root == NULL) {
return;
}
postOrder(root->left);
postOrder(root->right);
printf("%c ", root->val);
}
// 计算叶子结点个数
int countLeaves(TreeNode* root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
return countLeaves(root->left) + countLeaves(root->right);
}
// 计算结点个数
int countNodes(TreeNode* root) {
if (root == NULL) {
return 0;
}
return countNodes(root->left) + countNodes(root->right) + 1;
}
int main() {
int pos = 0;
TreeNode* root = createTree(&pos);
printf("先序遍历:");
preOrder(root);
printf("\n");
printf("中序遍历:");
inOrder(root);
printf("\n");
printf("后序遍历:");
postOrder(root);
printf("\n");
printf("叶子结点个数:%d\n", countLeaves(root));
printf("结点个数:%d\n", countNodes(root));
return 0;
}
```
相关推荐
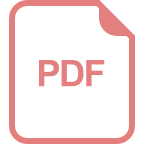
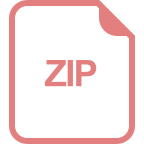
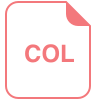
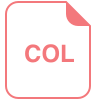
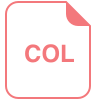
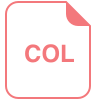
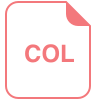









