from collections import Counter my_list = [1, 2, 3, 4, 5, 1, 2, 3, 1, 2, 1] counter = Counter(my_list) most_common = counter.most_common(1) print(most_common[0][0])
时间: 2024-03-31 20:37:06 浏览: 16
这段代码的作用是:给出一个列表 `my_list`,找出列表中出现次数最多的元素,并将其打印出来。
具体实现如下:
1. 导入 `collections` 模块中的 `Counter` 函数。
2. 定义一个列表 `my_list`,其中包含了一些元素。
3. 使用 `Counter` 函数统计列表 `my_list` 中每个元素出现的次数,将其保存在 `counter` 变量中。
4. 使用 `most_common()` 函数找出出现次数最多的元素和它的出现次数,将其保存在 `most_common` 变量中。
5. 打印出出现次数最多的元素,即 `most_common[0][0]`,其中第一个 `[0]` 表示第一项,第二个 `[0]` 表示第一项中的元素。因为 `most_common` 变量是一个列表,其中包含了元素和出现次数,而我们只需要元素,因此使用了两个 `[0]` 来取出元素。
运行这段代码,将会输出 `1`,表示列表中出现次数最多的元素是 `1`。
相关问题
from collections import Countermy_list = [3, 4, 1, 2, 5, 3, 2, 5, 6, 7, 4, 8, 9, 9, 8]counter = Counter(my_list)duplicates = [item for item, count in counter.items() if count > 1]duplicates.sort()print(duplicates) my_list改为读取输入并用空格分开
好的,可以使用input()函数读取一行输入,然后使用split()函数将其按空格分开,最终得到一个数字列表。修改后的代码如下:
```
from collections import Counter
my_list = list(map(int, input().split()))
counter = Counter(my_list)
duplicates = [item for item, count in counter.items() if count > 1]
duplicates.sort()
print(duplicates)
```
注意,这里使用了map()函数将输入的每个字符串转换为整数。
py计算列表my_list = [1, 2,2, 3, 3, 3]中每个元素出现的次数
您好!对于您的问题,可以使用Python内置的collections模块中的Counter方法来计算列表中每个元素出现的次数,代码如下:
``` python
from collections import Counter
my_list = [1, 2, 2, 3, 3, 3]
count_dict = Counter(my_list)
print(count_dict)
```
输出结果为:
``` python
Counter({3: 3, 2: 2, 1: 1})
```
其中,Counter返回的是一个字典,字典的键是列表中不同的元素,值是该元素出现的次数。
相关推荐
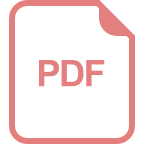
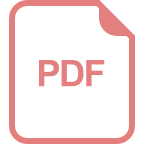
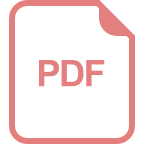













