如何修改代码,使得输出的每一个词的对应词频和密度分成两列显示 import pandas as pd import re from collections import Counter 读取词典txt文件 with open('词典.txt', 'r', encoding='utf-8') as f: list_dict = [line.strip() for line in f] 读取F列数据 df = pd.read_excel('实验体.xlsx') list_f = df['Answer'].tolist() 统计每行文本中的词频 dict_count_list = [] total_density_list = [] for text in list_f: # 匹配文本中的词列表 text = str(text) words = re.findall('|'.join(list_dict), text) # 统计每个词在该行文本中的出现次数和密度 dict_count = Counter(words) dict_count_list.append(dict_count) dict_count = {} for word in words: count = text.count(word) density = count / len(text) dict_count[word] = {'count': count, 'density': density} dict_count_list.append(dict_count) # 计算每行总词数的密度 total_density = sum([v['density'] for v in dict_count.values()]) total_density_list.append(total_density) 将每行文本的词频统计结果合并为一个DataFrame对象 df_count = pd.DataFrame(dict_count_list) df_count.index = df.index 输出为Excel文件 writer = pd.ExcelWriter('数实验体10.xlsx') df_count.to_excel(writer, sheet_name='Sheet1') writer._save()
时间: 2024-03-03 13:53:27 浏览: 124
你可以尝试修改代码如下:
```python
import pandas as pd
import re
from collections import Counter
# 读取词典txt文件
with open('词典.txt', 'r', encoding='utf-8') as f:
list_dict = [line.strip() for line in f]
# 读取F列数据
df = pd.read_excel('实验体.xlsx')
list_f = df['Answer'].tolist()
# 统计每行文本中的词频和密度
dict_count_list = []
total_density_list = []
for text in list_f:
# 匹配文本中的词列表
text = str(text)
words = re.findall('|'.join(list_dict), text)
# 统计每个词在该行文本中的出现次数和密度
dict_count = Counter(words)
dict_count = {k: {'count': v, 'density': v / len(text)} for k, v in dict_count.items()}
dict_count_list.append(dict_count)
# 计算每行总词数的密度
total_density = sum([v['density'] for v in dict_count.values()])
total_density_list.append(total_density)
# 将每行文本的词频统计结果合并为一个DataFrame对象
df_count = pd.concat([pd.DataFrame.from_dict(d, orient='index') for d in dict_count_list], axis=1)
df_count.index = df.index
# 输出为Excel文件并将每个词的词频和密度分成两列显示
with pd.ExcelWriter('数实验体10.xlsx') as writer:
df_count.to_excel(writer, sheet_name='Sheet1')
for col in df_count.columns:
if col.endswith('count'):
continue
count_col = col[:-7] + 'count'
density_col = col[:-7] + 'density'
df_count[[count_col, density_col]] = df_count[col].apply(pd.Series)
df_count = df_count.drop(col, axis=1)
df_count.to_excel(writer, sheet_name='Sheet1', startrow=0, startcol=len(df_count.columns))
```
修改后的代码会将每个词的词频和密度分成两列显示,并输出到Excel文件的第二列。
阅读全文
相关推荐
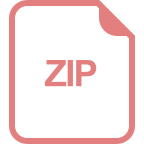
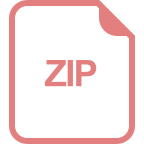















