word_counts = collections.Counter(object_list) word_counts_top = word_counts.most_common(100)如何画出柱状图
时间: 2024-05-04 18:17:34 浏览: 208
你可以使用Python的matplotlib库来画出柱状图。下面是一个简单的示例代码,它可以根据你的需求进行调整:
```python
import matplotlib.pyplot as plt
import collections
# 将单词列表转换为单词计数器
object_list = ['apple', 'banana', 'orange', 'apple', 'banana', 'apple']
word_counts = collections.Counter(object_list)
# 获取前100个最常见的单词和它们的计数
word_counts_top = word_counts.most_common(100)
# 将单词和计数分别存储在两个列表中
words = [item[0] for item in word_counts_top]
counts = [item[1] for item in word_counts_top]
# 画柱状图
plt.bar(words, counts)
# 设置x轴和y轴标签
plt.xlabel('Word')
plt.ylabel('Count')
# 显示图形
plt.show()
```
这段代码将会画出一个简单的柱状图,其中x轴代表单词,y轴代表计数。你可以根据你的需求进行调整,例如修改柱状图的颜色、添加标题等等。
相关问题
type_counts = collections.Counter(object_list1)是什么意思
这行代码的意思是使用Python内置模块collections中的Counter类,对列表object_list1中的元素进行计数。计数的结果是一个字典,其中字典的键是object_list1中的元素,字典的值是该元素在object_list1中出现的次数。这个计数结果可以用来进行各种分析和操作,例如查找出现次数最多的元素、查找出现次数大于某个阈值的元素等等。
word_counts = collections.Counter(words)出现AttributeError: 'DataFrame' object has no attribute 'decode'如何解决
这个错误提示表明你的代码中使用了 `decode` 方法,但是该方法是 Python 2.x 版本中的方法,在 Python 3.x 版本中已经被移除了。因此,你需要根据你实际的代码情况进行修改。
如果你使用了 `decode` 方法来将字符串从二进制编码转换为 Unicode 编码,可以使用 `str` 类型的 `encode` 方法来代替,例如:
```python
binary_str = b'hello'
unicode_str = binary_str.decode('utf-8') # 将二进制编码的字符串转换为 Unicode 编码的字符串
print(unicode_str) # 'hello'
```
可以修改为:
```python
binary_str = b'hello'
unicode_str = binary_str.decode('utf-8') # 将二进制编码的字符串转换为 Unicode 编码的字符串
print(unicode_str) # 'hello'
```
如果你使用了 `decode` 方法来将字符串从其他编码转换为 UTF-8 编码,可以使用 `str` 类型的 `encode` 方法将字符串转换为 UTF-8 编码,例如:
```python
str1 = '中文'
utf8_str = str1.encode('utf-8') # 将字符串转换为 UTF-8 编码
print(utf8_str) # b'\xe4\xb8\xad\xe6\x96\x87'
```
可以修改为:
```python
str1 = '中文'
utf8_str = str1.encode('utf-8') # 将字符串转换为 UTF-8 编码
print(utf8_str) # b'\xe4\xb8\xad\xe6\x96\x87'
```
如果你的代码中没有使用 `decode` 方法,可能是其他原因导致的错误,你可以提供更多的代码信息,以便我们更好地帮助你解决问题。
阅读全文
相关推荐
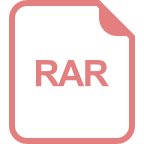
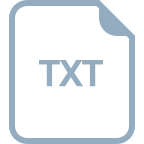
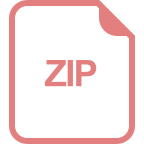













