帮我生成一个非支配排序遗传算法python代码
时间: 2023-10-14 19:06:07 浏览: 73
好的,以下是一个简单的非支配排序遗传算法的Python代码示例:
```python
import random
# 遗传算法参数
POPULATION_SIZE = 50 # 种群大小
P_CROSSOVER = 0.9 # 交叉概率
P_MUTATION = 0.1 # 变异概率
MAX_GENERATIONS = 50 # 最大迭代次数
# 非支配排序遗传算法函数
def non_dominated_sorting(genomes):
# 初始化支配解列表和支配该解的个数
domination_counts = [0] * len(genomes)
dominated_solutions = [[] for _ in range(len(genomes))]
# 初始化等级
ranks = [0] * len(genomes)
# 计算每个解对其他解的支配关系
for i in range(len(genomes)):
for j in range(len(genomes)):
if i == j:
continue
if all(genomes[i][k] <= genomes[j][k] for k in range(len(genomes[i]))):
dominated_solutions[i].append(j)
domination_counts[j] += 1
# 如果没有其他解支配该解,则该解为第一等级
if domination_counts[i] == 0:
ranks[i] = 1
# 计算其他等级
current_rank = 1
while any(rank == 0 for rank in ranks):
current_rank += 1
for i in range(len(genomes)):
if ranks[i] == 0 and domination_counts[i] == 0:
ranks[i] = current_rank
for j in dominated_solutions[i]:
domination_counts[j] -= 1
return ranks
# 交叉函数
def crossover(parent1, parent2):
# 选择一个随机交叉点
crossover_point = random.randint(1, len(parent1) - 1)
# 交叉
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 变异函数
def mutate(genome):
# 选择一个随机变异点
mutation_point = random.randint(0, len(genome) - 1)
# 变异
new_value = random.uniform(0, 1)
mutated_genome = genome[:mutation_point] + [new_value] + genome[mutation_point+1:]
return mutated_genome
# 创建初始种群
population = [[random.uniform(0, 1) for _ in range(2)] for _ in range(POPULATION_SIZE)]
# 迭代
for generation in range(MAX_GENERATIONS):
# 非支配排序
ranks = non_dominated_sorting(population)
# 计算每个解的拥挤度
crowding_distances = [0] * len(population)
for rank in range(1, max(ranks)+1):
rank_solutions = [i for i in range(len(population)) if ranks[i] == rank]
for i in range(len(rank_solutions[0])):
rank_solutions.sort(key=lambda x: population[x][i])
crowding_distances[rank_solutions[0]] = float("inf")
crowding_distances[rank_solutions[-1]] = float("inf")
for j in range(1, len(rank_solutions)-1):
crowding_distances[rank_solutions[j]] += population[rank_solutions[j+1]][i] - population[rank_solutions[j-1]][i]
# 新种群
new_population = []
# 交叉
while len(new_population) < POPULATION_SIZE:
parent1 = random.choice(population)
parent2 = random.choice(population)
if random.random() < P_CROSSOVER:
child1, child2 = crossover(parent1, parent2)
new_population.append(child1)
new_population.append(child2)
# 变异
for i in range(len(new_population)):
if random.random() < P_MUTATION:
new_population[i] = mutate(new_population[i])
# 合并种群
population += new_population
# 非支配排序
ranks = non_dominated_sorting(population)
# 选择下一代
next_population = []
for rank in range(1, max(ranks)+1):
rank_solutions = [i for i in range(len(population)) if ranks[i] == rank]
rank_solutions.sort(key=lambda x: crowding_distances[x], reverse=True)
next_population += [population[rank_solutions[i]] for i in range(len(rank_solutions)) if len(next_population) + i < POPULATION_SIZE]
population = next_population
# 最终种群
print(population)
```
注:此代码示例仅用于演示非支配排序遗传算法的基本思想,具体实现应根据实际情况进行调整和优化。
阅读全文
相关推荐
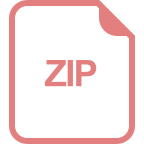

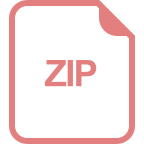
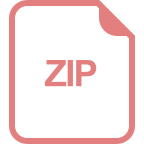
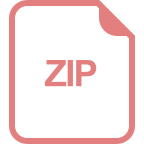
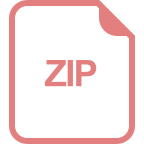

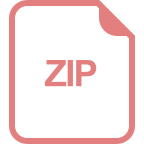
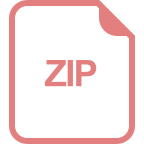
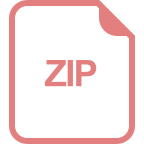
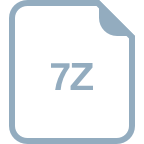
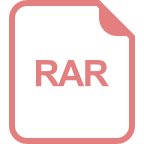
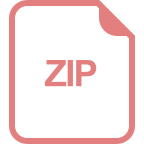
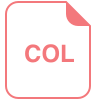
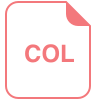

