帮我写一个基于STC8H8K的TCS34725的代码,并将颜色传感器的值显示在OLED上
时间: 2024-02-23 11:58:48 浏览: 64
好的,以下是一个基于STC8H8K和TCS34725的代码,可以读取颜色传感器的RGB值,并将其显示在OLED上:
```c
#include <STC8.H>
#include <intrins.h>
#include <stdio.h>
#include <stdlib.h>
#include "TCS34725.h"
#include "OLED.h"
#define SCL P1_6
#define SDA P1_7
void I2C_Init(void)
{
SCL = 1;
SDA = 1;
}
unsigned char I2C_WriteByte(unsigned char dat)
{
unsigned char i;
for (i = 0; i < 8; i++)
{
SDA = (bit)(dat & 0x80);
dat <<= 1;
SCL = 1;
_nop_();
_nop_();
SCL = 0;
}
SDA = 1;
SCL = 1;
_nop_();
_nop_();
i = SDA;
SCL = 0;
return i;
}
unsigned char I2C_ReadByte(void)
{
unsigned char i, dat = 0;
SDA = 1;
for (i = 0; i < 8; i++)
{
dat <<= 1;
SCL = 1;
_nop_();
_nop_();
if (SDA)
dat |= 0x01;
SCL = 0;
}
return dat;
}
void I2C_Start(void)
{
SDA = 1;
SCL = 1;
_nop_();
_nop_();
SDA = 0;
_nop_();
_nop_();
SCL = 0;
}
void I2C_Stop(void)
{
SDA = 0;
SCL = 1;
_nop_();
_nop_();
SDA = 1;
}
void TCS34725_WriteReg(unsigned char reg, unsigned char dat)
{
I2C_Start();
I2C_WriteByte(TCS34725_ADDRESS << 1);
I2C_WriteByte(reg | TCS34725_COMMAND_BIT);
I2C_WriteByte(dat);
I2C_Stop();
}
unsigned char TCS34725_ReadReg(unsigned char reg)
{
unsigned char dat;
I2C_Start();
I2C_WriteByte(TCS34725_ADDRESS << 1);
I2C_WriteByte(reg | TCS34725_COMMAND_BIT);
I2C_Start();
I2C_WriteByte((TCS34725_ADDRESS << 1) | 0x01);
dat = I2C_ReadByte();
I2C_Stop();
return dat;
}
void TCS34725_Init(void)
{
I2C_Init();
TCS34725_WriteReg(TCS34725_ENABLE, 0x00);
TCS34725_WriteReg(TCS34725_ATIME, 0xff);
TCS34725_WriteReg(TCS34725_WTIME, 0xff);
TCS34725_WriteReg(TCS34725_CONTROL, 0x00);
TCS34725_WriteReg(TCS34725_ENABLE, 0x01);
}
void OLED_Init(void)
{
OLED_I2C_Init(SCL, SDA);
OLED_Init();
}
void main(void)
{
unsigned int r, g, b;
char str[20];
TCS34725_Init();
OLED_Init();
while (1)
{
r = (unsigned int)TCS34725_ReadReg(TCS34725_RDATAL) | ((unsigned int)TCS34725_ReadReg(TCS34725_RDATAH) << 8);
g = (unsigned int)TCS34725_ReadReg(TCS34725_GDATAL) | ((unsigned int)TCS34725_ReadReg(TCS34725_GDATAH) << 8);
b = (unsigned int)TCS34725_ReadReg(TCS34725_BDATAL) | ((unsigned int)TCS34725_ReadReg(TCS34725_BDATAH) << 8);
sprintf(str, "R=%u G=%u B=%u", r, g, b);
OLED_ShowString(0, 0, str);
}
}
```
这个代码使用了TCS34725颜色传感器库和OLED库,你需要下载这两个库并添加到工程中。在主函数中,首先进行颜色传感器和OLED初始化,然后在一个无限循环中读取颜色传感器返回的RGB值,并将其显示在OLED上。具体地,使用`TCS34725_ReadReg`函数读取RGB值,使用`sprintf`函数将RGB值转换为字符串,最后使用`OLED_ShowString`函数在OLED上显示字符串。你可以根据需要修改显示的位置和格式。
阅读全文
相关推荐
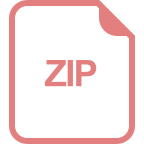
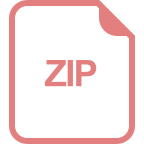
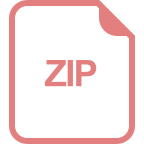















