通过openpyxl模块像表格中写入东西
时间: 2023-08-10 09:51:52 浏览: 143
好的,你可以通过以下步骤使用openpyxl模块向Excel表格中写入内容:
1. 导入openpyxl模块:`import openpyxl`
2. 打开Excel文件:`wb = openpyxl.load_workbook('文件名.xlsx')`
3. 选择要操作的表格:`sheet = wb['表格名']`
4. 写入内容,比如在A1单元格中写入'Hello World!':`sheet['A1'] = 'Hello World!'`
5. 保存文件:`wb.save('文件名.xlsx')`
注意,如果表格中不存在要操作的单元格,可以使用`sheet.cell(row, column)`方法来创建新的单元格并写入内容。例如,在第2行第3列的单元格中写入'Python':`sheet.cell(row=2, column=3, value='Python')`。另外,你也可以使用for循环来批量写入数据。
相关问题
openpyxl怎么批量从字典中写入各个子表格
在使用Python库openpyxl操作Excel文件时,如果你想从一个字典批量写入到工作簿的不同子表(即工作表),可以按照以下步骤进行:
1. 首先,导入openpyxl模块以及可能需要的pandas库(如果你的字典是从pandas DataFrame转换过来的)。
```python
import pandas as pd
from openpyxl import load_workbook, Workbook
```
2. 加载现有的工作簿,或者创建一个新的Workbook对象。
```python
# 加载现有工作簿
workbook = load_workbook('your_file.xlsx')
# 或者新建一个工作簿
workbook = Workbook()
```
3. 获取你想要写的子工作表。你可以通过索引来访问,例如`workbook['Sheet1']`。
4. 将字典转换为DataFrame,因为openpyxl直接处理DataFrame更为方便。
```python
if 'data_dict' in locals(): # 假设你有一个名为data_dict的字典
df = pd.DataFrame.from_dict(data_dict)
```
5. 然后遍历字典,将每个子字典的数据写入相应的子表。
```python
for sheet_name, data in data_dict.items():
ws = workbook[sheet_name] # 获取当前工作表
df.to_excel(ws, index=False) # 写入数据,index=False表示不写入行索引
```
6. 最后保存修改。
```python
workbook.save('your_file.xlsx') # 如果是加载的文件,替换为'data_dict.xlsx'
```
注意:这里假设`data_dict`的键是工作表名,值是对应的子字典,如果不是这种情况,你需要调整代码逻辑以匹配实际的字典结构。
openpyxl给写入的表格增加边框
在Python中,`openpyxl`是一个用于读写Excel 2010 xlsx/xlsm/xltx/xltm文件的库。如果你想要给写入的表格添加边框,首先你需要导入`openpyxl.styles`模块,然后可以创建`BorderStyle`和`Side`对象来设置边框样式。以下是一个简单的例子:
```python
from openpyxl import Workbook
from openpyxl.styles import Border, Side
# 创建一个新的工作簿
wb = Workbook()
# 选择你要操作的工作表
sheet = wb.active
# 添加边框样式
thin_border = Border(left=Side(style='thin', color='FF0000'), # 红色细线
right=Side(style='thin', color='00FF00'), # 绿色细线
top=Side(style='thin', color='0000FF'), # 蓝色细线
bottom=Side(style='thin', color='FFFF00')) # 黄色细线
# 应用边框到单元格
cell = sheet['A1']
cell.border = thin_border
# 写入数据
sheet['A1'] = 'Hello, World!'
wb.save('example.xlsx')
```
在这个示例中,我们为单元格A1设置了四条细边框,分别代表左、右、上、下边。
阅读全文
相关推荐
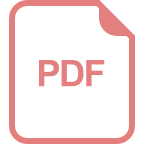
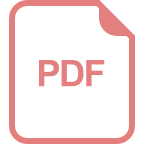
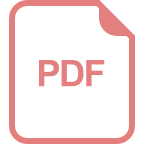













