解析ldf文件 代码c++
时间: 2023-12-12 09:02:35 浏览: 61
以下是使用C++解析LDF文件的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <vector>
#include <map>
#include "rapidxml.hpp"
using namespace std;
using namespace rapidxml;
// 定义LDF节点结构体
struct LDFNode {
string name; // 节点名称
int nadc; // 节点ADC值
};
// 定义LDF数据帧结构体
struct LDFFrame {
int id; // 数据帧ID
string name; // 数据帧名称
int length; // 数据帧长度
vector<string> signals; // 数据帧包含的信号列表
};
// 解析LDF文件
void parseLDF(const string& filename, map<string, LDFNode>& nodes, vector<LDFFrame>& frames) {
ifstream file(filename);
stringstream buffer;
buffer << file.rdbuf();
file.close();
xml_document<> doc;
doc.parse<0>(&buffer[0]);
// 解析节点信息
xml_node<>* node = doc.first_node("LDF")->first_node("LIN_Nodes")->first_node("LIN_Node");
while (node) {
LDFNode ldfNode;
ldfNode.name = node->first_attribute("Node_Name")->value();
ldfNode.nadc = atoi(node->first_attribute("NAD")->value());
nodes[ldfNode.name] = ldfNode;
node = node->next_sibling("LIN_Node");
}
// 解析数据帧信息
node = doc.first_node("LDF")->first_node("LIN_Frame");
while (node) {
LDFFrame ldfFrame;
ldfFrame.id = strtol(node->first_attribute("Frame_ID")->value(), NULL, 16);
ldfFrame.name = node->first_attribute("Frame_Name")->value();
ldfFrame.length = atoi(node->first_attribute("Frame_Length")->value());
// 解析数据帧包含的信号信息
xml_node<>* signal = node->first_node("LIN_Signal");
while (signal) {
ldfFrame.signals.push_back(signal->first_attribute("Signal_Name")->value());
signal = signal->next_sibling("LIN_Signal");
}
frames.push_back(ldfFrame);
node = node->next_sibling("LIN_Frame");
}
}
int main() {
map<string, LDFNode> nodes;
vector<LDFFrame> frames;
parseLDF("example.ldf", nodes, frames);
// 输出解析结果
for (auto& node : nodes) {
cout << "Node name: " << node.second.name << ", NADC: " << node.second.nadc << endl;
}
for (auto& frame : frames) {
cout << "Frame ID: " << frame.id << ", Frame name: " << frame.name << ", Frame length: " << frame.length << endl;
cout << "Signals: ";
for (auto& signal : frame.signals) {
cout << signal << " ";
}
cout << endl;
}
return 0;
}
```
该代码使用了第三方XML解析库rapidxml,其中`parseLDF`函数实现了对LDF文件的解析,解析结果保存在`nodes`和`frames`变量中。解析结果可以根据需要进行进一步处理和使用。
相关推荐
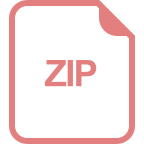














