findViewById详细解释
时间: 2023-12-15 21:06:35 浏览: 41
findViewById() 是 Android 开发中常用的一个方法,用于在布局文件中查找指定的视图控件。该方法的作用是根据控件的 ID 查找到对应的 View 对象,并返回该对象的引用。它通常在 Activity 或 Fragment 中使用。
方法签名为:`public View findViewById(int id)`
其中,id 表示要查找的控件的 ID,它是一个整型值,可以在布局文件中通过 `android:id` 属性指定。
使用该方法的步骤如下:
1. 在布局文件中给控件指定一个 id,例如:
```xml
<Button
android:id="@+id/btn_ok"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="OK" />
```
2. 在代码中使用 findViewById() 方法查找该控件:
```java
Button btnOk = findViewById(R.id.btn_ok);
```
3. 然后就可以使用 btnOk 对象来操作该控件了,例如设置点击事件等操作。
需要注意的是,如果在布局文件中没有指定 id 或者指定的 id 在当前布局中不存在,那么调用 findViewById() 方法就会返回 null,因此在使用时需要进行 null 判断。
相关问题
可以详细解释一下上面的例子吗
当一个 Activity 发送广播时,其他组件(如 Service)可以通过注册广播接收器来接收该广播,并执行相应的操作。下面是一个示例,演示了如何在 Activity 和 Service 之间进行通信。
首先,在发送广播的 Activity 中,我们需要定义一个广播接收器,并在发送广播时注册该接收器。在这个示例中,我们假设用户在 EditText 中输入一个整数 n,并点击按钮发送广播。
```java
public class MainActivity extends AppCompatActivity {
private EditText numberEditText;
private Button sendButton;
private BroadcastReceiver broadcastReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
int result = intent.getIntExtra("factorial", 0);
textView.setText("Factorial: " + result);
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
numberEditText = findViewById(R.id.numberEditText);
sendButton = findViewById(R.id.sendButton);
sendButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int number = Integer.parseInt(numberEditText.getText().toString());
Intent intent = new Intent("com.example.FACTORIAL_ACTION");
intent.putExtra("number", number);
sendBroadcast(intent);
}
});
}
@Override
protected void onResume() {
super.onResume();
IntentFilter filter = new IntentFilter("com.example.FACTORIAL_ACTION");
registerReceiver(broadcastReceiver, filter);
}
@Override
protected void onPause() {
super.onPause();
unregisterReceiver(broadcastReceiver);
}
}
```
在上述代码中,我们定义了一个 BroadcastReceiver,当接收到广播时,它会从 Intent 中提取计算阶乘结果的值,并将其显示在 TextView 上。
接下来,在接收广播的 Service 中,我们需要注册一个广播接收器,并在接收到广播时执行相应的操作。在这个示例中,我们计算接收到的数字的阶乘,并将结果发送回 Activity。
```java
public class FactorialService extends Service {
private BroadcastReceiver broadcastReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
int number = intent.getIntExtra("number", 0);
int result = calculateFactorial(number);
Intent resultIntent = new Intent("com.example.FACTORIAL_ACTION");
resultIntent.putExtra("factorial", result);
sendBroadcast(resultIntent);
}
};
@Override
public void onCreate() {
super.onCreate();
IntentFilter filter = new IntentFilter("com.example.FACTORIAL_ACTION");
registerReceiver(broadcastReceiver, filter);
}
@Override
public void onDestroy() {
super.onDestroy();
unregisterReceiver(broadcastReceiver);
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
private int calculateFactorial(int n) {
int factorial = 1;
for (int i = 2; i <= n; i++) {
factorial *= i;
}
return factorial;
}
}
```
在上述代码中,我们定义了一个 BroadcastReceiver,当接收到广播时,它会从 Intent 中提取输入的数字,并计算其阶乘。然后,它将结果放入新的 Intent 中,并发送广播回 Activity。
为了使 Service 能够接收广播,我们需要在 AndroidManifest.xml 文件中声明 Service:
```xml
<service android:name=".FactorialService" />
```
这样,当 Activity 发送广播时,Service 就能够接收到该广播,并执行相应的操作。接收到结果后,Activity 的广播接收器会更新 TextView 上显示的内容,即计算出的阶乘结果。
请注意,为了避免内存泄漏,我们在 Activity 的 onResume() 方法中注册广播接收器,并在 onPause() 方法中取消注册。这样可以确保在 Activity 不可见时,不会继续接收广播。
android studio开发简易计算器全部代码详细解释
好的,下面我将为您提供一份简易计算器的Android Studio开发代码,同时对代码进行详细解释。
首先,我们需要在Android Studio中创建一个新的项目,然后在布局文件中添加UI组件,如下所示:
```xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<EditText
android:id="@+id/et_num1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:hint="输入数字1"
android:inputType="number"
app:layout_constraintEnd_toStartOf="@id/tv_symbol"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/tv_symbol"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="+"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="@id/et_num1"
app:layout_constraintEnd_toStartOf="@id/et_num2"
app:layout_constraintStart_toEndOf="@id/et_num1"
app:layout_constraintTop_toTopOf="@id/et_num1" />
<EditText
android:id="@+id/et_num2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:hint="输入数字2"
android:inputType="number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.5"
app:layout_constraintStart_toEndOf="@id/tv_symbol"
app:layout_constraintTop_toTopOf="@id/et_num1" />
<Button
android:id="@+id/btn_calculate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="计算"
app:layout_constraintEnd_toEndOf="@id/et_num2"
app:layout_constraintStart_toStartOf="@id/et_num1"
app:layout_constraintTop_toBottomOf="@id/et_num1" />
<TextView
android:id="@+id/tv_result"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="计算结果"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="@id/et_num2"
app:layout_constraintStart_toStartOf="@id/et_num1"
app:layout_constraintTop_toBottomOf="@id/btn_calculate" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
上述布局文件中包含四个EditText组件分别用于输入两个数字,一个TextView组件用于显示计算符号,一个Button组件用于执行计算操作,一个TextView组件用于显示计算结果。
在MainActivity.java文件中,我们需要进行如下操作:
1. 绑定UI组件:使用findViewById方法将UI组件与Java代码中的变量进行绑定。
```java
EditText etNum1 = findViewById(R.id.et_num1);
EditText etNum2 = findViewById(R.id.et_num2);
TextView tvSymbol = findViewById(R.id.tv_symbol);
Button btnCalculate = findViewById(R.id.btn_calculate);
TextView tvResult = findViewById(R.id.tv_result);
```
2. 注册事件监听器:使用setOnClickListener方法为按钮组件注册事件监听器,当用户点击按钮时,执行计算操作。
```java
btnCalculate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 执行计算操作
}
});
```
3. 执行计算操作:获取用户输入的数字并执行相应的计算操作,将计算结果显示在结果TextView组件中。
```java
String strNum1 = etNum1.getText().toString();
String strNum2 = etNum2.getText().toString();
int num1 = Integer.parseInt(strNum1);
int num2 = Integer.parseInt(strNum2);
int result = 0;
switch (tvSymbol.getText().toString()) {
case "+":
result = num1 + num2;
break;
case "-":
result = num1 - num2;
break;
case "*":
result = num1 * num2;
break;
case "/":
result = num1 / num2;
break;
}
tvResult.setText(String.valueOf(result));
```
上述代码中,首先获取用户输入的数字并将其转换为整型,然后根据计算符号执行相应的计算操作,将计算结果转换为字符串并显示在结果TextView组件中。
至此,一个简单的计算器应用程序就完成了。通过这个例子,您可以了解到如何创建UI组件、注册事件监听器以及执行简单的计算操作。
相关推荐
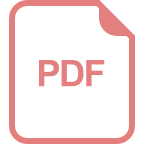
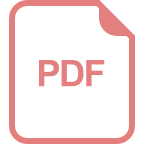












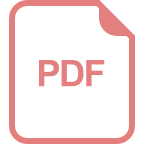