用JAVA代码编写 定义数组存储3部汽车对象。 汽车的属性:品牌,价格,颜色。 创建三个汽车对象,数据通过键盘录入而来,并把数据存入到数组当中。
时间: 2024-09-29 09:10:04 浏览: 39
在Java中,你可以使用ArrayList来动态地存储不同类型的对象,比如汽车。首先,你需要创建一个Car类作为汽车的对象,包含品牌(brand)、价格(price)和颜色(color)属性。然后你可以定义一个方法来获取用户输入并创建新的Car对象。以下是一个简单的示例:
```java
import java.util.ArrayList;
import java.util.Scanner;
class Car {
String brand;
double price;
String color;
// 构造函数
public Car(String brand, double price, String color) {
this.brand = brand;
this.price = price;
this.color = color;
}
@Override
public String toString() {
return "Brand: " + brand + ", Price: " + price + ", Color: " + color;
}
}
public class Main {
public static void main(String[] args) {
ArrayList<Car> carArray = new ArrayList<>();
Scanner scanner = new Scanner(System.in);
System.out.println("Enter details for the first car:");
System.out.print("Brand: ");
String brand1 = scanner.nextLine();
System.out.print("Price: ");
double price1 = scanner.nextDouble();
System.out.print("Color: ");
String color1 = scanner.nextLine();
Car car1 = new Car(brand1, price1, color1);
carArray.add(car1);
// 可以继续添加其他两部车,这里只举一例
System.out.println("Enter details for the second car:");
System.out.print("Brand: ");
String brand2 = scanner.nextLine();
System.out.print("Price: ");
double price2 = scanner.nextDouble();
System.out.print("Color: ");
String color2 = scanner.nextLine();
Car car2 = new Car(brand2, price2, color2);
carArray.add(car2);
scanner.close();
}
}
```
在这个例子中,我们从用户那里获取输入,然后创建对应的Car对象并将其添加到ArrayList中。运行程序时,用户会依次被提示输入每辆汽车的品牌、价格和颜色。
阅读全文
相关推荐
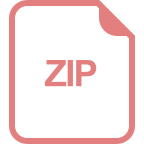
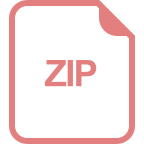
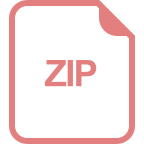
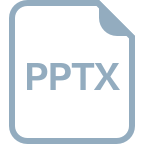
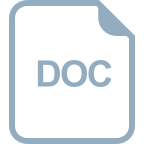
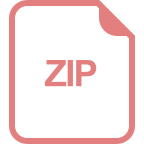
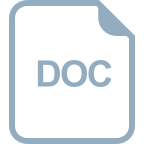
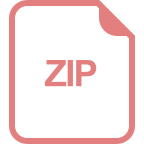
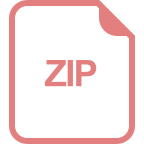
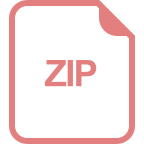
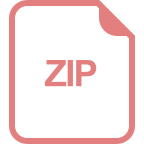
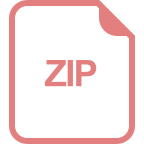
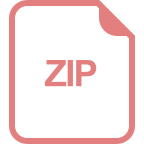
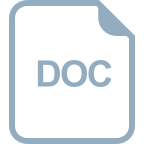
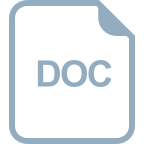
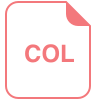
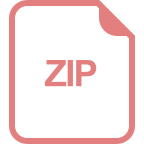
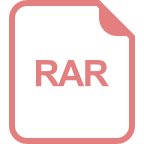