java的FTP工具类
时间: 2023-12-23 13:28:04 浏览: 29
以下是一个Java的FTP工具类的示例代码:
```java
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
import java.io.*;
public class FTPUtil {
private FTPClient ftpClient;
public FTPUtil() {
ftpClient = new FTPClient();
}
public boolean connect(String server, int port, String user, String password) {
try {
ftpClient.connect(server, port);
int replyCode = ftpClient.getReplyCode();
if (!FTPReply.isPositiveCompletion(replyCode)) {
return false;
}
boolean success = ftpClient.login(user, password);
if (!success) {
return false;
}
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
ftpClient.enterLocalPassiveMode();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
public boolean uploadFile(String localFilePath, String remoteFilePath) {
try (InputStream inputStream = new FileInputStream(localFilePath)) {
return ftpClient.storeFile(remoteFilePath, inputStream);
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
public boolean downloadFile(String remoteFilePath, String localFilePath) {
try (OutputStream outputStream = new FileOutputStream(localFilePath)) {
return ftpClient.retrieveFile(remoteFilePath, outputStream);
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
public boolean disconnect() {
try {
ftpClient.logout();
ftpClient.disconnect();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
}
```
使用该工具类,你可以连接到FTP服务器,上传文件和下载文件。你需要引入Apache Commons Net库,该库提供了FTP客户端的实现。
相关推荐
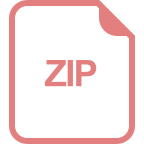














