java,ftp工具类
时间: 2023-10-17 17:20:39 浏览: 35
下面是一个简单的 Java FTP 工具类,可以用于上传、下载和删除文件:
```
import java.io.*;
import org.apache.commons.net.ftp.*;
public class FTPClientUtil {
private FTPClient ftpClient;
public FTPClientUtil() {
ftpClient = new FTPClient();
}
public void connect(String server, int port, String username, String password) throws IOException {
ftpClient.connect(server, port);
ftpClient.login(username, password);
}
public void disconnect() throws IOException {
ftpClient.logout();
ftpClient.disconnect();
}
public void uploadFile(String localFilePath, String remoteFilePath) throws IOException {
File localFile = new File(localFilePath);
InputStream inputStream = new FileInputStream(localFile);
ftpClient.storeFile(remoteFilePath, inputStream);
inputStream.close();
}
public void downloadFile(String localFilePath, String remoteFilePath) throws IOException {
OutputStream outputStream = new FileOutputStream(localFilePath);
ftpClient.retrieveFile(remoteFilePath, outputStream);
outputStream.close();
}
public void deleteFile(String remoteFilePath) throws IOException {
ftpClient.deleteFile(remoteFilePath);
}
}
```
使用示例:
```
FTPClientUtil ftp = new FTPClientUtil();
ftp.connect("ftp.example.com", 21, "username", "password");
ftp.uploadFile("/path/to/local/file.txt", "/path/to/remote/file.txt");
ftp.downloadFile("/path/to/local/file.txt", "/path/to/remote/file.txt");
ftp.deleteFile("/path/to/remote/file.txt");
ftp.disconnect();
```
需要注意的是,这个工具类依赖于 Apache Commons Net 库,需要先添加这个库的依赖。
相关推荐
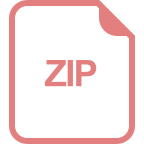














