C语言求最大公约数和最小公倍数,两种方法,一种用全局变量,一种不用全局变量
时间: 2024-10-11 10:10:14 浏览: 27
在C语言中,求两个整数的最大公约数(Greatest Common Divisor, GCD)和最小公倍数(Least Common Multiple, LCM)可以采用多种算法。以下是两种常见的非全局变量方法:
**方法一:欧几里得算法(辗转相除法) - 没有全局变量**
```c
#include <stdio.h>
// 函数声明,传入两个整数计算GCD
int gcd(int a, int b);
// 计算LCM基于GCD,没有全局变量
int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
// 实现欧几里得算法
int gcd(int a, int b) {
if (b == 0)
return a;
else
return gcd(b, a % b);
}
// 主函数示例
int main() {
int num1, num2;
printf("请输入两个正整数:");
scanf("%d %d", &num1, &num2);
int result = lcm(num1, num2);
printf("两数的最大公约数(GCD)是:%d\n", gcd(num1, num2));
printf("它们的最小公倍数(LCM)是:%d\n", result);
return 0;
}
```
这种方法的优点是不需要全局变量,函数之间数据传递简洁。
**方法二:欧几里得算法 + 临时变量 - 仅用于GCD,全局变量用于存储中间结果**
```c
#include <stdio.h>
#include <stdlib.h> // 使用malloc申请内存
// 定义全局变量存储中间结果
unsigned long long int temp;
// 函数声明
int gcd_iterative(int a, int b);
// 主函数
int main() {
int num1, num2;
printf("请输入两个正整数:");
scanf("%d %d", &num1, &num2);
int result = gcd_iterative(num1, num2);
printf("两数的最大公约数(GCD)是:%d\n", result);
free(temp); // 清理内存
return 0;
}
// 实现迭代版本的GCD
int gcd_iterative(int a, int b) {
if (b == 0) {
temp = a; // 全局变量保存最终结果
return a;
} else {
return gcd_iterative(b, a % b);
}
}
```
这种方法通过全局变量存储中间结果,使得算法在递归过程中可以复用这个值。但通常推荐避免过多使用全局变量,因为它可能会增加程序复杂性和维护难度。
阅读全文
相关推荐
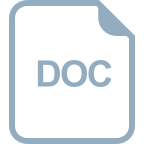
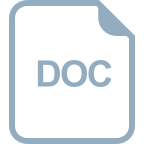
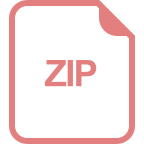









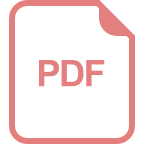
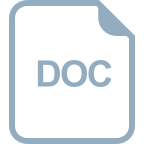
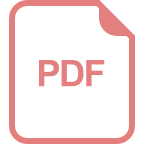
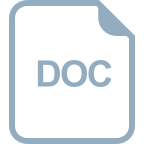
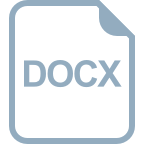
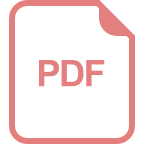
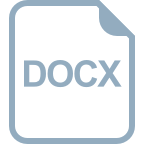