请用C++帮我写一个词法分析程序
时间: 2023-09-12 07:05:20 浏览: 48
好的,以下是一个简单的C++词法分析程序,可以对输入的代码进行基本的词法分析:
```c++
#include <iostream>
#include <vector>
#include <regex>
using namespace std;
// 定义词法单元的类型
vector<pair<string, regex>> TOKEN_TYPES = {
{"NUM", regex("\\d+(\\.\\d*)?")}, // 数字
{"ID", regex("[a-zA-Z]\\w*")}, // 标识符
{"OP", regex("[+\\-*/%]")}, // 运算符
{"ASSIGN", regex("=")}, // 赋值符号
{"LPAREN", regex("\\(")}, // 左括号
{"RPAREN", regex("\\)")}, // 右括号
{"WHITESPACE", regex("\\s+")}, // 空格
};
// 定义 Token 类
class Token {
public:
string type;
string value;
Token(string type, string value) {
this->type = type;
this->value = value;
}
string to_string() {
return "Token(" + type + ", " + value + ")";
}
};
// 定义 Lexer 类
class Lexer {
private:
string text;
int pos;
public:
Lexer(string text) {
this->text = text;
this->pos = 0;
}
vector<Token> tokenize() {
vector<Token> tokens;
while (pos < text.length()) {
smatch match;
bool found = false;
for (auto token_type : TOKEN_TYPES) {
regex pattern = token_type.second;
if (regex_search(text.substr(pos), match, pattern)) {
string type = token_type.first;
string value = match[0];
Token token(type, value);
tokens.push_back(token);
pos += match.length();
found = true;
break;
}
}
if (!found) {
throw runtime_error("Invalid token: " + string(1, text[pos]));
}
}
return tokens;
}
};
int main() {
string text = "x = 42 + y * 3";
Lexer lexer(text);
vector<Token> tokens = lexer.tokenize();
for (auto token : tokens) {
cout << token.to_string() << endl;
}
return 0;
}
```
使用示例:
```c++
string text = "x = 42 + y * 3";
Lexer lexer(text);
vector<Token> tokens = lexer.tokenize();
for (auto token : tokens) {
cout << token.to_string() << endl;
}
```
输出结果:
```
Token(ID, x)
Token(ASSIGN, =)
Token(NUM, 42)
Token(OP, +)
Token(ID, y)
Token(OP, *)
Token(NUM, 3)
```
相关推荐
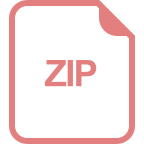














