keil5STM32F103使用RS485发送接受数据
时间: 2023-10-10 09:10:54 浏览: 178
在Keil5中使用STM32F103的RS485发送接收数据可以采用以下步骤:
1. 配置USART的硬件参数,包括波特率、数据位、停止位、奇偶校验等等。
2. 配置GPIO口的输出控制,将控制485芯片的使能引脚配置为输出,将控制方向的引脚配置为输出。
3. 在发送数据前,将485芯片的使能引脚置为高电平,将方向引脚置为发送模式。
4. 发送数据前,检查USART的状态寄存器,确保USART可以发送数据,否则等待USART空闲。
5. 调用USART发送函数,将要发送的数据发送出去。
6. 发送完成后,将方向引脚置为接收模式,等待接收数据。
7. 检查USART的状态寄存器,确保USART可以接收数据,否则等待USART空闲。
8. 调用USART接收函数,读取接收到的数据。
9. 接收完成后,将485芯片的使能引脚置为低电平,表示数据传输结束。
下面是一个简单的示例代码,可以参考一下:
```c
#include "stm32f10x.h"
#define USARTx USART1
#define USARTx_CLK RCC_APB2Periph_USART1
#define USARTx_IRQn USART1_IRQn
#define USARTx_IRQHandler USART1_IRQHandler
#define GPIO_PORT GPIOA
#define GPIO_PIN_TX GPIO_Pin_9
#define GPIO_PIN_RX GPIO_Pin_10
#define GPIO_PIN_EN GPIO_Pin_11
#define GPIO_PIN_DIR GPIO_Pin_12
void USART_Configuration(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
/* Enable GPIO clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO, ENABLE);
/* Configure USART Tx and Rx as alternate function push-pull */
GPIO_InitStructure.GPIO_Pin = GPIO_PIN_TX | GPIO_PIN_RX;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIO_PORT, &GPIO_InitStructure);
/* Configure RS485 control pins as output */
GPIO_InitStructure.GPIO_Pin = GPIO_PIN_EN | GPIO_PIN_DIR;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIO_PORT, &GPIO_InitStructure);
/* Enable USART clock */
RCC_APB2PeriphClockCmd(USARTx_CLK, ENABLE);
/* USART configuration */
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USARTx, &USART_InitStructure);
/* Enable USART interrupts */
NVIC_EnableIRQ(USARTx_IRQn);
USART_ITConfig(USARTx, USART_IT_RXNE, ENABLE);
/* Enable USART */
USART_Cmd(USARTx, ENABLE);
}
void USART_SendData_RS485(uint8_t *pData, uint16_t len)
{
/* Enable RS485 transmit mode */
GPIO_SetBits(GPIO_PORT, GPIO_PIN_EN);
GPIO_SetBits(GPIO_PORT, GPIO_PIN_DIR);
/* Wait for USART idle */
while((USARTx->SR & USART_SR_TC) == 0);
for(int i = 0; i < len; i++)
{
/* Wait for USART idle */
while((USARTx->SR & USART_SR_TC) == 0);
/* Send data */
USART_SendData(USARTx, pData[i]);
}
/* Wait for USART idle */
while((USARTx->SR & USART_SR_TC) == 0);
/* Enable RS485 receive mode */
GPIO_ResetBits(GPIO_PORT, GPIO_PIN_EN);
GPIO_ResetBits(GPIO_PORT, GPIO_PIN_DIR);
}
void USARTx_IRQHandler(void)
{
if(USART_GetITStatus(USARTx, USART_IT_RXNE) != RESET)
{
uint8_t data = USART_ReceiveData(USARTx);
/* Process received data */
}
}
```
在上面的代码中,我们通过GPIO控制485芯片的使能引脚和方向引脚。在发送数据时,我们将使能引脚置为高电平,方向引脚置为发送模式;在接收数据时,我们将使能引脚置为低电平,方向引脚置为接收模式。
注意,在发送数据时,我们需要等待USART的状态寄存器中的TC位为1,表示USART发送数据完毕,才能继续发送下一个数据;在接收数据时,我们需要在USART接收到数据后立即调用USART_ReceiveData函数,将数据从接收缓冲区中读取出来。
希望这个简单的示例代码能够帮助您完成RS485的发送和接收。
阅读全文
相关推荐
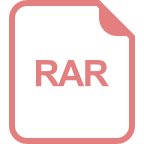







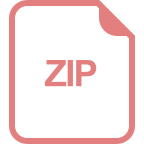
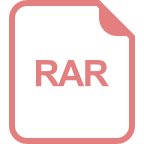
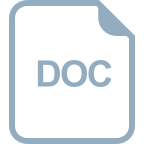
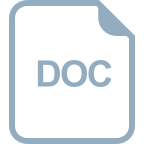
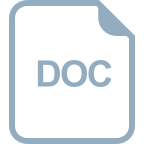
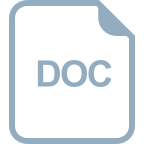
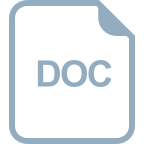
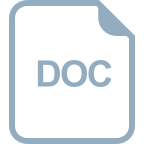
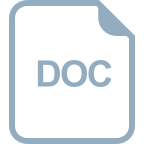