同时实现问题1可以吗
时间: 2024-10-20 14:17:15 浏览: 10
当然可以。以下是对问题1的具体要求和建议:
### 问题1:实现Conway’s Game of Life
**任务描述:**
编写一个C++程序来演示Conway’s Game of Life。程序应允许用户确定网格大小、初始活细胞的数量以及游戏进行的步数。初始活细胞的位置在网格中随机分布。使用ASCII字符显示每一步的模拟结果,并提供保存和加载功能以便暂停和重新开始模拟。
**具体要求:**
1. **输入参数:**
- 用户输入网格大小(例如10x10)。
- 用户输入初始活细胞的数量。
- 用户输入游戏进行的步数。
2. **输出格式:**
- 使用“.”表示网格的边界。
- 使用空格“ ”表示死细胞。
- 使用“O”表示活细胞。
- 每一步的模拟结果显示为一个ASCII网格。
3. **示例输出:**
```
. . . . . . . . . .
. . . . . .O.O. . .
. . . . . . . . . .
. . . . .O. . . . .
. . . . . . . . . .
. . . . . . . . . .
. . .O.O. . . . . .
. . .O. . . . . . .
. . . . . . . . . .
. . . . . . . . . .
```
4. **保存和加载功能:**
- 允许将当前状态保存到文件。
- 允许从文件加载先前的状态并继续模拟。
### 实现建议:
1. **数据结构:**
- 使用二维数组或向量来存储网格状态。
- 可以使用`std::vector<std::vector<bool>>`来表示网格,其中`true`表示活细胞,`false`表示死细胞。
2. **函数设计:**
- `initializeGrid(int rows, int cols, int numAlive)`:初始化网格,随机分配活细胞。
- `printGrid(const std::vector<std::vector<bool>>& grid)`:打印当前网格状态。
- `updateGrid(std::vector<std::vector<bool>>& grid)`:根据Conway的游戏规则更新网格状态。
- `saveState(const std::vector<std::vector<bool>>& grid, const std::string& filename)`:将当前网格状态保存到文件。
- `loadState(std::vector<std::vector<bool>>& grid, const std::string& filename)`:从文件加载网格状态。
3. **主程序逻辑:**
- 获取用户输入的网格大小、初始活细胞数量和步数。
- 初始化网格并打印初始状态。
- 进行指定步数的模拟,每一步后打印当前状态。
- 提供菜单选项让用户选择保存当前状态或加载先前状态。
### 示例代码框架:
```cpp
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
// Initialize the grid with random alive cells
void initializeGrid(int rows, int cols, int numAlive, std::vector<std::vector<bool>>& grid) {
// Seed the random number generator
srand(time(0));
// Set all cells to dead initially
grid.resize(rows, std::vector<bool>(cols, false));
// Randomly place alive cells
while (numAlive > 0) {
int row = rand() % rows;
int col = rand() % cols;
if (!grid[row][col]) {
grid[row][col] = true;
--numAlive;
}
}
}
// Print the current state of the grid
void printGrid(const std::vector<std::vector<bool>>& grid) {
int rows = grid.size();
int cols = grid[0].size();
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
std::cout << (grid[i][j] ? "O" : " ") << " ";
}
std::cout << std::endl;
}
}
// Update the grid according to the rules of Conway's Game of Life
void updateGrid(std::vector<std::vector<bool>>& grid) {
int rows = grid.size();
int cols = grid[0].size();
std::vector<std::vector<bool>> newGrid(rows, std::vector<bool>(cols, false));
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
int liveNeighbors = 0;
for (int di = -1; di <= 1; ++di) {
for (int dj = -1; dj <= 1; ++dj) {
if (di == 0 && dj == 0) continue;
int ni = i + di;
int nj = j + dj;
if (ni >= 0 && ni < rows && nj >= 0 && nj < cols && grid[ni][nj]) {
++liveNeighbors;
}
}
}
if (grid[i][j] && (liveNeighbors == 2 || liveNeighbors == 3)) {
newGrid[i][j] = true;
} else if (!grid[i][j] && liveNeighbors == 3) {
newGrid[i][j] = true;
}
}
}
grid.swap(newGrid);
}
int main() {
int rows, cols, numAlive, steps;
std::cout << "Enter the number of rows: ";
std::cin >> rows;
std::cout << "Enter the number of columns: ";
std::cin >> cols;
std::cout << "Enter the number of initial alive cells: ";
std::cin >> numAlive;
std::cout << "Enter the number of steps: ";
std::cin >> steps;
std::vector<std::vector<bool>> grid;
initializeGrid(rows, cols, numAlive, grid);
std::cout << "Initial Grid:\n";
printGrid(grid);
for (int step = 1; step <= steps; ++step) {
updateGrid(grid);
std::cout << "Step " << step << ":\n";
printGrid(grid);
}
return 0;
}
```
这个框架提供了基本的功能,你可以在此基础上添加保存和加载功能以及其他高级特性。希望这对你有所帮助!
阅读全文
相关推荐
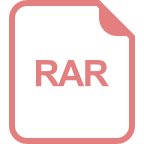
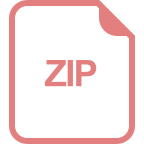
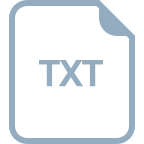
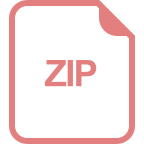
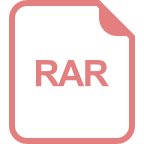
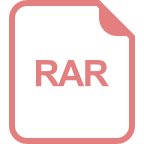
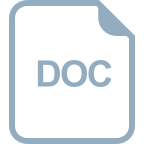
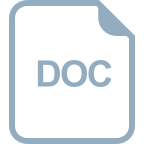
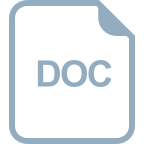
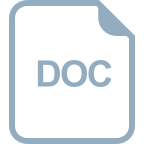
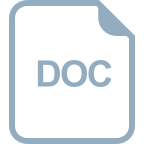
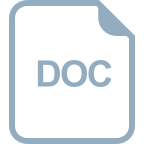
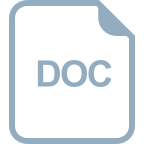
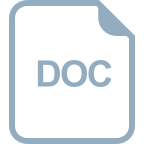
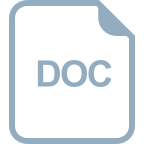
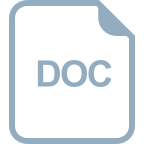
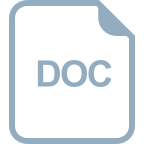
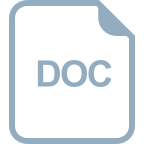