用c语言实现并给出相关注释,输入一个树的中序遍历序列 该树后序遍历序列,中间用空格分开。输入序列中仅含有小写字母,且没有重复的字母, 输出一个树的前序遍历序列
时间: 2023-12-30 11:05:30 浏览: 26
以下是用 C 语言实现的代码及注释:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
// 定义二叉树节点结构体
typedef struct node {
char val;
struct node* left;
struct node* right;
} Node;
// 根据中序遍历序列和后序遍历序列重建二叉树
Node* buildTree(char* inorder, char* postorder, int len) {
if (len <= 0) {
return NULL;
}
// 后序遍历序列的最后一个元素是根节点
char rootVal = postorder[len-1];
Node* root = (Node*)malloc(sizeof(Node));
root->val = rootVal;
root->left = NULL;
root->right = NULL;
if (len == 1) {
return root;
}
// 在中序遍历序列中找到根节点位置
int idx = 0;
while (inorder[idx] != rootVal) {
idx++;
}
// 递归构建左子树和右子树
int leftLen = idx;
int rightLen = len - idx - 1;
root->left = buildTree(inorder, postorder, leftLen);
root->right = buildTree(inorder+idx+1, postorder+leftLen, rightLen);
return root;
}
// 前序遍历二叉树,输出序列
void preorder(Node* root) {
if (root == NULL) {
return;
}
printf("%c ", root->val);
preorder(root->left);
preorder(root->right);
}
int main() {
char inorder[100], postorder[100];
scanf("%s%s", inorder, postorder);
int len = strlen(inorder);
Node* root = buildTree(inorder, postorder, len);
preorder(root);
printf("\n");
return 0;
}
注:此代码仅供参考,可能存在某些细节问题,仅供学习交流使用。
相关推荐
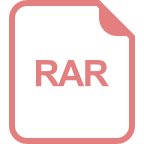
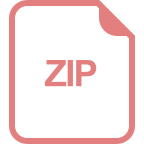
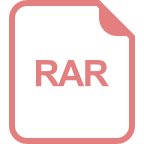















