题目描述 假设二叉树中每个结点值为单个字符(不超过100个),求二叉树的先序、中序、后序序列。 输入格式 一个字符串(括号表示法表示二叉树) 输出格式 三行,每行一个字符串(分别表示二叉树的先序、中序、后序序列)用C语言做
时间: 2023-12-03 14:43:56 浏览: 107
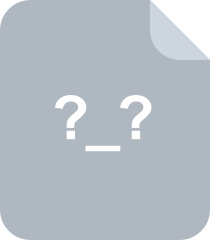
二叉树的前序,中序,后序排列C语言程序
以下是用 C 语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_N 10005
typedef struct TreeNode {
char val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
void preorder(TreeNode* root, char* res, int* idx) {
if (!root) return;
res[(*idx)++] = root->val;
preorder(root->left, res, idx);
preorder(root->right, res, idx);
}
void inorder(TreeNode* root, char* res, int* idx) {
if (!root) return;
inorder(root->left, res, idx);
res[(*idx)++] = root->val;
inorder(root->right, res, idx);
}
void postorder(TreeNode* root, char* res, int* idx) {
if (!root) return;
postorder(root->left, res, idx);
postorder(root->right, res, idx);
res[(*idx)++] = root->val;
}
int find(char* s, int l, int r) {
int cnt = 0;
for (int i = l; i <= r; i++) {
if (s[i] == '(') cnt++;
else if (s[i] == ')') cnt--;
if (cnt == 0 && (s[i] == ',' || s[i] == ')')) return i;
}
return -1;
}
TreeNode* build(char* s, int l, int r) {
if (l > r) return NULL;
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = s[l];
root->left = root->right = NULL;
if (l == r) return root;
int i = find(s, l+2, r-1);
if (i == -1) return root;
root->left = build(s, l+2, i-1);
root->right = build(s, i+1, r-1);
return root;
}
int main() {
char s[MAX_N];
scanf("%s", s);
TreeNode* root = build(s, 0, strlen(s)-1);
char pre[MAX_N] = {0}, in[MAX_N] = {0}, post[MAX_N] = {0};
int idx = 0;
preorder(root, pre, &idx);
idx = 0;
inorder(root, in, &idx);
idx = 0;
postorder(root, post, &idx);
printf("%s\n%s\n%s\n", pre, in, post);
return 0;
}
```
注释:
- `preorder()`、`inorder()` 和 `postorder()` 分别实现二叉树的先序、中序和后序遍历,并将遍历结果存储在 `res` 数组中。
- `find()` 函数用于查找括号表示法中某个结点的右括号位置,即该结点的子树在字符串中的范围。具体实现是维护一个计数器 `cnt`,遇到左括号则 `cnt++`,遇到右括号则 `cnt--`,当 `cnt` 减为 0 且当前字符为逗号或右括号时,返回右括号位置。
- `build()` 函数递归构建二叉树。首先根据左括号位置 `l` 取出根结点的值,然后调用 `find()` 函数找到根结点左子树和右子树的范围,递归构建左子树和右子树,返回根结点。
- `main()` 函数读入字符串,调用 `build()` 函数构建二叉树,再分别调用 `preorder()`、`inorder()` 和 `postorder()` 函数得到遍历结果,最后输出即可。
阅读全文
相关推荐











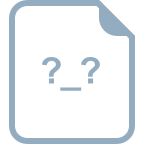