一元多项式的简单计算器
时间: 2023-05-10 15:55:06 浏览: 64
好的,我可以回答这个问题。一元多项式的简单计算器可以通过定义一个多项式类来实现。在这个类中,我们可以定义多项式的系数和次数,并实现加、减、乘、除等基本运算。以下是一个简单的示例代码:
```python
class Polynomial:
def __init__(self, coeffs):
self.coeffs = coeffs
def __add__(self, other):
return Polynomial([a + b for a, b in zip(self.coeffs, other.coeffs)])
def __sub__(self, other):
return Polynomial([a - b for a, b in zip(self.coeffs, other.coeffs)])
def __mul__(self, other):
coeffs = [0] * (len(self.coeffs) + len(other.coeffs) - 1)
for i, a in enumerate(self.coeffs):
for j, b in enumerate(other.coeffs):
coeffs[i+j] += a * b
return Polynomial(coeffs)
def __truediv__(self, other):
q = [0] * (len(self.coeffs) - len(other.coeffs) + 1)
r = self
while len(r.coeffs) >= len(other.coeffs):
d = [0] * (len(r.coeffs) - len(other.coeffs)) + other.coeffs
mult = q[len(r.coeffs) - len(other.coeffs)] = r.coeffs[-1] / d[-1]
d = [coeff * mult for coeff in d]
r = r - Polynomial(d)
return Polynomial(q), r
def __str__(self):
return ' + '.join([f'{coeff}x^{i}' for i, coeff in enumerate(self.coeffs) if coeff != 0])
# 示例用法
p1 = Polynomial([1, 2, 3]) # 1 + 2x + 3x^2
p2 = Polynomial([4, 5, 6]) # 4 + 5x + 6x^2
print(p1 + p2) # 5 + 7x + 9x^2
print(p1 - p2) # -3 - 3x - 3x^2
print(p1 * p2) # 4 + 13x + 28x^2 + 27x^3 + 18x^4
print(p1 / p2) # (Polynomial([0.16666666666666666]), Polynomial([-0.16666666666666666, -0.16666666666666666]))
```
这个多项式类实现了加、减、乘、除等基本运算,可以用来计算一元多项式的值。
相关推荐
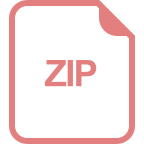
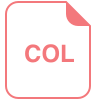













