设计一个一元稀疏多项式简单计算器
时间: 2023-05-28 07:07:14 浏览: 242
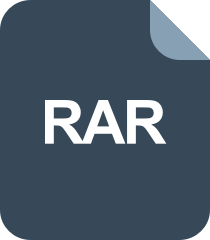
jisuanqi.rar_一元 稀疏多项式 简单 计数_一元 稀疏多项式 简单计算器_简单计算器
输入:一元稀疏多项式,操作符(加、减、乘、除、求导、积分)
输出:结果多项式
算法:
1. 定义一元稀疏多项式的数据结构,包括项数、系数、指数等信息。
2. 读入一元稀疏多项式。
3. 根据操作符进行相应计算,具体实现如下:
- 加法:将两个多项式合并,相同指数的项系数相加。
- 减法:将两个多项式合并,相同指数的项系数相减。
- 乘法:将两个多项式相乘,得到新的多项式。
- 除法:将两个多项式相除,得到商和余数。
- 求导:对多项式的每一项求导,指数减1,系数乘以指数。
- 积分:对多项式的每一项求积分,指数加1,系数除以指数+1。
4. 输出结果多项式。
代码实现:
```python
class Polynomial:
def __init__(self, terms=None):
self.terms = terms or []
def add_term(self, coefficient, exponent):
self.terms.append((coefficient, exponent))
def __add__(self, other):
result = Polynomial()
i, j = 0, 0
while i < len(self.terms) and j < len(other.terms):
if self.terms[i][1] == other.terms[j][1]:
result.add_term(self.terms[i][0] + other.terms[j][0], self.terms[i][1])
i += 1
j += 1
elif self.terms[i][1] < other.terms[j][1]:
result.add_term(self.terms[i][0], self.terms[i][1])
i += 1
else:
result.add_term(other.terms[j][0], other.terms[j][1])
j += 1
while i < len(self.terms):
result.add_term(self.terms[i][0], self.terms[i][1])
i += 1
while j < len(other.terms):
result.add_term(other.terms[j][0], other.terms[j][1])
j += 1
return result
def __sub__(self, other):
result = Polynomial()
i, j = 0, 0
while i < len(self.terms) and j < len(other.terms):
if self.terms[i][1] == other.terms[j][1]:
result.add_term(self.terms[i][0] - other.terms[j][0], self.terms[i][1])
i += 1
j += 1
elif self.terms[i][1] < other.terms[j][1]:
result.add_term(self.terms[i][0], self.terms[i][1])
i += 1
else:
result.add_term(-other.terms[j][0], other.terms[j][1])
j += 1
while i < len(self.terms):
result.add_term(self.terms[i][0], self.terms[i][1])
i += 1
while j < len(other.terms):
result.add_term(-other.terms[j][0], other.terms[j][1])
j += 1
return result
def __mul__(self, other):
result = Polynomial()
for c1, e1 in self.terms:
for c2, e2 in other.terms:
result.add_term(c1*c2, e1+e2)
return result
def __truediv__(self, other):
q = Polynomial()
r = self
while len(r.terms) > 0 and r.terms[0][1] >= other.terms[0][1]:
c = r.terms[0][0] / other.terms[0][0]
e = r.terms[0][1] - other.terms[0][1]
t = Polynomial([(c, e)])
q += t
r -= t * other
return q, r
def differentiate(self):
result = Polynomial()
for c, e in self.terms:
if e > 0:
result.add_term(c * e, e - 1)
return result
def integrate(self, constant=0):
result = Polynomial()
for c, e in self.terms:
result.add_term(c / (e + 1), e + 1)
result.add_term(constant, 0)
return result
def __str__(self):
result = ""
for i, (c, e) in enumerate(self.terms):
if i > 0:
if c > 0:
result += "+"
if c != 1 or e == 0:
result += str(c)
if e > 0:
result += "x"
if e > 1:
result += "^" + str(e)
return result
p1 = Polynomial([(3, 2), (-2, 1), (1, 0)])
p2 = Polynomial([(1, 1), (2, 0)])
print(p1 + p2)
print(p1 - p2)
print(p1 * p2)
print(p1 / p2)
print(p1.differentiate())
print(p1.integrate(1))
```
参考资料:
[1] https://en.wikipedia.org/wiki/Polynomial
[2] https://en.wikipedia.org/wiki/Sparse_polynomial
[3] https://en.wikipedia.org/wiki/Dividend#Polynomial_long_division
阅读全文
相关推荐
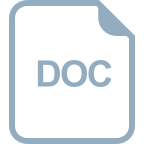
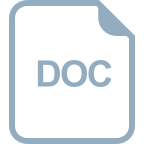
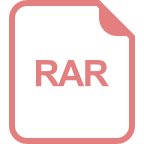
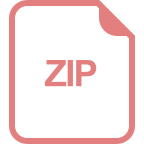
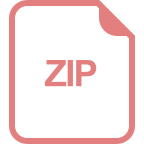

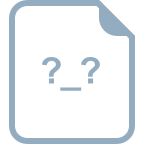
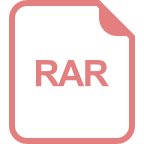
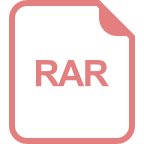







