用c++语言设计一个一元稀疏多项式简单计算器。
时间: 2024-02-20 13:59:04 浏览: 229
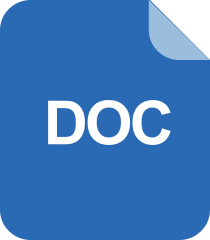
一元稀疏多项式计算器
下面是一个简单的一元稀疏多项式计算器的C++实现:
```cpp
#include <iostream>
#include <map>
using namespace std;
// 多项式项
struct Term {
int coeff; // 系数
int exp; // 指数
};
// 多项式
class Polynomial {
private:
map<int, int> terms; // 用map存储多项式的项,键为指数,值为系数
public:
// 构造函数
Polynomial() {}
// 添加一个项
void add_term(int coeff, int exp) {
if (coeff == 0) return; // 系数为0直接返回
if (terms.find(exp) != terms.end()) { // 指数已经存在
terms[exp] += coeff; // 系数相加
if (terms[exp] == 0) // 系数为0则删除该项
terms.erase(exp);
} else { // 指数不存在
terms[exp] = coeff;
}
}
// 求导
void differentiate() {
for (auto it = terms.begin(); it != terms.end(); ++it) {
int exp = it->first;
int coeff = it->second;
if (exp == 0) { // 常数项
it->second = 0;
} else { // 非常数项
it->second = coeff * exp;
it->first = exp - 1;
}
}
}
// 求值
int evaluate(int x) {
int result = 0;
for (auto it = terms.begin(); it != terms.end(); ++it) {
int exp = it->first;
int coeff = it->second;
result += coeff * pow(x, exp);
}
return result;
}
// 输出多项式
void print() {
bool first = true;
for (auto it = terms.rbegin(); it != terms.rend(); ++it) {
int exp = it->first;
int coeff = it->second;
if (coeff > 0) {
if (!first) cout << "+";
} else {
cout << "-";
}
first = false;
if (abs(coeff) != 1 || exp == 0) cout << abs(coeff);
if (exp > 0) cout << "x";
if (exp > 1) cout << "^" << exp;
}
cout << endl;
}
};
// 主函数
int main() {
Polynomial p;
// 读入多项式
int coeff, exp;
while (cin >> coeff >> exp) {
p.add_term(coeff, exp);
}
// 输出多项式
p.print();
// 求导并输出
p.differentiate();
cout << "The derivative is: ";
p.print();
// 求值并输出
int x;
cout << "Enter the value of x: ";
cin >> x;
int result = p.evaluate(x);
cout << "The value of the polynomial at x = " << x << " is: " << result << endl;
return 0;
}
```
这个计算器支持读入一元稀疏多项式,求导,求值等操作。在实现中,使用了map来存储多项式的项,键为指数,值为系数。添加项时,如果指数已经存在,则将新系数加到原系数上;如果指数不存在,则直接插入新项。求导时,对于每一项,系数变为原系数乘以指数,指数减1;对于常数项,将系数设为0。求值时,遍历所有项,将每一项的系数乘以x的指数次方,然后累加到结果中。输出多项式时,从高到低遍历所有项,根据系数的正负和指数的大小输出不同的符号和系数的绝对值,指数为0时不输出x,指数为1时不输出指数的符号。
阅读全文
相关推荐
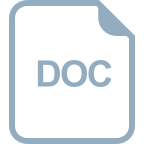
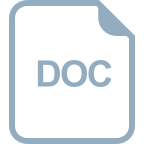
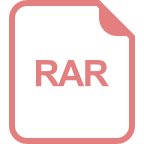
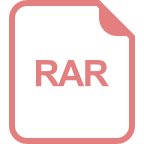
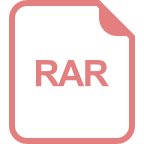
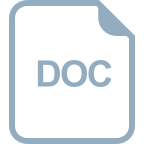

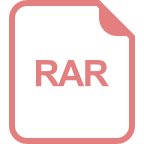
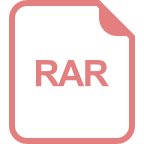
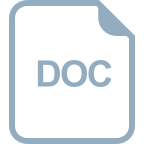
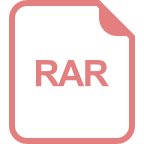
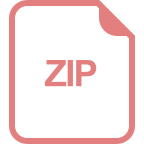
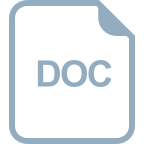
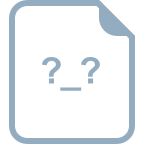



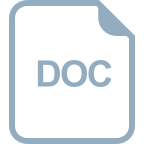