python查找列表中最长元素
时间: 2023-06-14 10:08:00 浏览: 82
可以使用Python中的`max()`函数结合`len()`函数来查找列表中最长的元素。例如:
```python
my_list = ['apple', 'banana', 'orange', 'kiwi']
longest_element = max(my_list, key=len)
print(longest_element)
```
输出结果为:
```
banana
```
在这个例子中,`max()`函数返回了`my_list`中最长的元素`'banana'`,而`key=len`则指定按元素长度进行比较。
相关问题
python 最长递增子序列
Python中求最长递增子序列的代码可以有多种实现方式。引用\[1\]中给出了一种实现方式,使用了动态规划的思想。该代码定义了一个函数`lis`,接受一个整数数组作为参数。它首先初始化一个长度为数组长度的列表`m`,用于记录以每个元素结尾的最长递增子序列的长度。然后通过两层循环遍历数组,如果当前元素比后面的元素小且以当前元素结尾的子序列长度小于等于以后面元素结尾的子序列长度,则更新以当前元素结尾的子序列长度。最后找到`m`列表中的最大值,然后遍历数组,将与最大值相等的元素添加到结果列表中,并递减最大值,直到最大值为0。最后返回结果列表。给定的示例数组为`\[10, 22, 9, 33, 21, 50, 41, 60, 80\]`,调用`lis`函数后输出结果为`\[10, 22, 33, 50, 60, 80\]`。
另外,引用\[2\]中给出了另一种实现方式,使用了动态规划和二分查找的思想。该代码定义了一个类`Solution`,其中包含一个方法`lengthOfLIS`,接受一个整数数组作为参数。它首先判断数组是否为空,如果为空则返回0。然后初始化一个长度为数组长度的列表`dp`,用于记录以每个元素结尾的最长递增子序列的长度。通过两层循环遍历数组,如果当前元素比前面的元素大,则更新以当前元素结尾的子序列长度为前面元素结尾的子序列长度加1。最后返回`dp`列表中的最大值。这种实现方式的时间复杂度为O(n)。
#### 引用[.reference_title]
- *1* [Python 最长递增子序列代码](https://blog.csdn.net/deanhj/article/details/101634446)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [最长连续序列 python](https://blog.csdn.net/dearzhuiyi/article/details/126930325)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python中itertools的用法
Python中的itertools模块是一个用于迭代工具的标准库。它包含了很多用于迭代处理的函数和生成器,可以让开发者更加方便地处理迭代任务。
以下是itertools模块的一些常用函数:
1. itertools.count(start=0, step=1):生成从start开始的连续数字,步长为step。
2. itertools.cycle(iterable):将可迭代对象循环输出。
3. itertools.repeat(object, times=None):生成重复的对象,可以指定重复次数。
4. itertools.chain(*iterables):将多个可迭代对象串联起来,形成一个更长的迭代器。
5. itertools.product(*iterables, repeat=1):计算多个可迭代对象的笛卡尔积,repeat参数指定重复次数。
6. itertools.combinations(iterable, r):生成可迭代对象的所有长度为r的组合。
7. itertools.permutations(iterable, r=None):生成可迭代对象的所有长度为r的排列,默认r为可迭代对象的长度。
8. itertools.groupby(iterable, key=None):根据指定的key对可迭代对象进行分组。
以上只是itertools模块中的部分函数,还有很多其他有用的函数和生成器,可以根据需要选择使用。除了上述提到的itertools函数之外,这里还介绍几个常用的itertools函数:
1. itertools.islice(iterable, start, stop, step=1):生成一个迭代器,其中包含来自可迭代对象的切片,start指定开始索引,stop指定结束索引(不包含),step指定步长。
2. itertools.dropwhile(predicate, iterable):生成一个迭代器,其中包含从可迭代对象中跳过满足predicate条件的元素。
3. itertools.takewhile(predicate, iterable):生成一个迭代器,其中包含满足predicate条件的可迭代对象的元素,直到遇到第一个不满足条件的元素。
4. itertools.filterfalse(predicate, iterable):生成一个迭代器,其中包含从可迭代对象中返回False的元素。
5. itertools.zip_longest(*iterables, fillvalue=None):生成一个迭代器,其中包含来自多个可迭代对象的元素,以最长的可迭代对象为准,fillvalue指定缺失值的替换值。
这些函数和生成器可以使开发者更加高效地处理各种迭代任务。除了上述提到的itertools函数之外,还有一些其他有用的itertools函数,以下是一些常用的itertools函数:
1. itertools.compress(data, selectors):生成一个迭代器,其中包含来自data可迭代对象的元素,对应位置上selectors可迭代对象的元素为True,否则不包含。
2. itertools.dropwhile(predicate, iterable):生成一个迭代器,其中包含从可迭代对象中跳过满足predicate条件的元素。
3. itertools.takewhile(predicate, iterable):生成一个迭代器,其中包含满足predicate条件的可迭代对象的元素,直到遇到第一个不满足条件的元素。
4. itertools.filterfalse(predicate, iterable):生成一个迭代器,其中包含从可迭代对象中返回False的元素。
5. itertools.zip_longest(*iterables, fillvalue=None):生成一个迭代器,其中包含来自多个可迭代对象的元素,以最长的可迭代对象为准,fillvalue指定缺失值的替换值。
6. itertools.starmap(function, iterable):生成一个迭代器,其中包含将function应用于iterable中的元素后的结果。
7. itertools.tee(iterable, n=2):生成n个迭代器,每个迭代器都包含iterable中的元素,可用于并行处理可迭代对象。
8. itertools.combinations_with_replacement(iterable, r):生成可迭代对象的所有长度为r的组合,包括重复的元素。
9. itertools.groupby(iterable, key=None):根据指定的key对可迭代对象进行分组。
这些函数和生成器可以使开发者更加高效地处理各种迭代任务。itertools是Python中的一个模块,它提供了许多用于迭代器操作的工具函数。以下是一些itertools的用法:
1. permutations(iterable, r=None): 返回iterable中所有长度为r的排列。
2. combinations(iterable, r): 返回iterable中所有长度为r的组合。
3. combinations_with_replacement(iterable, r): 返回iterable中所有长度为r的组合,可以包含重复元素。
4. product(*iterables, repeat=1): 返回iterables中所有元素的笛卡尔积。
5. chain(*iterables): 将多个iterables串联起来。
6. cycle(iterable): 无限循环iterable中的元素。
7. repeat(object[, times]): 重复生成object,可指定重复次数。
使用itertools可以方便地处理迭代器操作,提高代码的效率和可读性。Python中的itertools模块是一个集成了一些用于迭代器操作的函数的模块。下面是一些itertools模块的常用函数及其用法:
1. itertools.chain(*iterables)
该函数可以把多个可迭代对象拼接成一个迭代器,返回值是一个迭代器。例如:chain('ABC', 'DEF')返回值是一个包含A、B、C、D、E、F的迭代器。
2. itertools.combinations(iterable, r)
该函数返回一个迭代器,生成由iterable中所有长度为r的组合。例如:combinations('ABCD', 2)返回值是一个包含AB、AC、AD、BC、BD、CD的迭代器。
3. itertools.product(*iterables, repeat=1)
该函数返回一个迭代器,生成由iterables中的元素的笛卡尔积,repeat参数指定重复迭代的次数。例如:product('ABCD', repeat=2)返回值是一个包含AA、AB、AC、AD、BA、BB、BC、BD、CA、CB、CC、CD、DA、DB、DC、DD的迭代器。
4. itertools.islice(iterable, start, stop[, step])
该函数返回一个迭代器,生成从iterable中start到stop-1之间的元素,step参数指定步长。例如:islice('ABCDEFG', 2, None)返回值是一个包含C、D、E、F、G的迭代器。
5. itertools.cycle(iterable)
该函数返回一个迭代器,不断重复iterable中的元素。例如:cycle('ABC')返回值是一个包含A、B、C、A、B、C、A、B、C...的迭代器。
6. itertools.groupby(iterable, key=None)
该函数返回一个生成器,按照key函数的返回值把iterable中的元素分组,key函数默认为None,表示使用元素自身的值作为key。例如:groupby('AAABBBCCAAA')返回值是一个生成器,每个元素都是(key, group)的形式,其中key是元素的值,group是一个包含所有与key相同的元素的迭代器。
这些函数是itertools模块中的一部分,其他函数的用法可以查看Python官方文档。itertools是Python标准库中提供的一个模块,包含了一些用于快速创建迭代器的工具函数。以下是itertools中一些常用函数的用法:
1. itertools.chain(*iterables)
将多个可迭代对象连接起来,返回一个迭代器。例如:
```
import itertools
lst1 = [1, 2, 3]
lst2 = [4, 5, 6]
lst3 = [7, 8, 9]
for i in itertools.chain(lst1, lst2, lst3):
print(i)
```
输出结果为:
```
1
2
3
4
5
6
7
8
9
```
2. itertools.count(start=0, step=1)
返回一个从start开始,步长为step的无限迭代器。例如:
```
import itertools
for i in itertools.count(1, 2):
print(i)
if i > 10:
break
```
输出结果为:
```
1
3
5
7
9
11
```
3. itertools.cycle(iterable)
将一个可迭代对象无限重复,返回一个迭代器。例如:
```
import itertools
lst = ['a', 'b', 'c']
for i, c in zip(range(5), itertools.cycle(lst)):
print(i, c)
```
输出结果为:
```
0 a
1 b
2 c
3 a
4 b
```
4. itertools.permutations(iterable, r=None)
返回一个可迭代对象,包含iterable中所有长度为r(默认为len(iterable))的排列。例如:
```
import itertools
lst = [1, 2, 3]
for p in itertools.permutations(lst, 2):
print(p)
```
输出结果为:
```
(1, 2)
(1, 3)
(2, 1)
(2, 3)
(3, 1)
(3, 2)
```
5. itertools.product(*iterables, repeat=1)
返回一个可迭代对象,包含iterables中所有元素的笛卡尔积。例如:
```
import itertools
lst1 = [1, 2]
lst2 = [3, 4]
for p in itertools.product(lst1, lst2):
print(p)
```
输出结果为:
```
(1, 3)
(1, 4)
(2, 3)
(2, 4)
```
以上是itertools中一些常用函数的用法,还有其他函数如combinations、groupby等也非常有用。Python的itertools模块是一个用于操作迭代器的工具库。该模块提供了许多用于操作迭代器的函数,如生成器、排列、组合、笛卡尔积等等。
下面是itertools模块中几个常用的函数:
1. itertools.count(start=0, step=1):创建一个从start开始的无限迭代器,每次迭代加上step。
2. itertools.cycle(iterable):对于给定的可迭代对象,创建一个无限迭代器,不断重复其中的元素。
3. itertools.chain(*iterables):将多个可迭代对象连接成一个迭代器,依次迭代每个可迭代对象中的元素。
4. itertools.islice(iterable, start, stop, step=1):对于给定的可迭代对象,创建一个迭代器,其中仅包含从start到stop的元素,每step个元素取一个。
5. itertools.permutations(iterable, r=None):对于给定的可迭代对象,创建一个迭代器,其中包含所有长度为r的排列。如果未提供r,则默认为可迭代对象的长度。
6. itertools.combinations(iterable, r):对于给定的可迭代对象,创建一个迭代器,其中包含所有长度为r的组合。
7. itertools.product(*iterables, repeat=1):对于给定的可迭代对象,创建一个迭代器,其中包含所有可迭代对象的笛卡尔积。如果repeat大于1,则将可迭代对象重复repeat次。Python中的itertools是一个用于创建迭代器的标准库。它提供了许多有用的函数,可以用于创建迭代器,生成组合、排列、笛卡尔积等。以下是几个常用的itertools函数:
1. permutations(iterable, r=None):生成一个由可迭代对象中所有长度为r的排列组成的迭代器。
2. combinations(iterable, r):生成一个由可迭代对象中所有长度为r的组合组成的迭代器。
3. product(*iterables, repeat=1):生成可迭代对象中每个元素的笛卡尔积的元素。
4. chain(*iterables):将多个可迭代对象连接起来,返回一个迭代器。
5. groupby(iterable, key=None):将可迭代对象中相邻且具有相同键值的元素分组成一个迭代器。
6. tee(iterable, n=2):将可迭代对象分成n份,并返回由n个迭代器组成的元组。
这些函数的使用方法非常简单,只需要将要处理的可迭代对象作为参数传入函数即可。例如,要生成一个由列表中所有长度为2的组合组成的迭代器,可以使用combinations函数,代码如下:
```
import itertools
lst = [1, 2, 3, 4]
combs = itertools.combinations(lst, 2)
for comb in combs:
print(comb)
```
运行结果为:
```
(1, 2)
(1, 3)
(1, 4)
(2, 3)
(2, 4)
(3, 4)
```Python中的itertools模块提供了用于迭代器操作的工具函数。以下是itertools模块中常用的一些函数及其用法:
1. itertools.chain():将多个迭代器连接成一个迭代器。
2. itertools.count():从指定数字开始计数,返回一个无限迭代器。
3. itertools.cycle():对给定的序列重复迭代,返回一个无限迭代器。
4. itertools.dropwhile():对序列中的元素迭代,当函数返回false时开始返回元素。
5. itertools.filterfalse():返回序列中不满足条件的元素。
6. itertools.groupby():对序列中连续的相同元素进行分组。
7. itertools.islice():对序列进行切片,返回一个迭代器。
8. itertools.permutations():返回序列的所有排列组合。
9. itertools.product():返回序列的笛卡尔积,即所有可能的组合。
10. itertools.repeat():重复生成指定对象。
11. itertools.takewhile():对序列中的元素迭代,当函数返回false时停止返回元素。
这些函数可以帮助我们更方便地进行迭代器操作,提高代码的效率。Python中的itertools模块是一个用于迭代器操作的标准库,可以用于生成各种不同类型的迭代器,例如排列、组合、笛卡尔积等等。
以下是itertools模块中一些常用函数的使用方法:
1. itertools.product(*iterables, repeat=1)
该函数用于生成迭代器的笛卡尔积,其中参数*iterables表示可迭代对象,repeat表示重复次数。例如:
```
import itertools
a = [1, 2, 3]
b = ['a', 'b', 'c']
c = itertools.product(a, b, repeat=2)
for i in c:
print(i)
```
输出:
```
(1, 'a', 1, 'a')
(1, 'a', 1, 'b')
(1, 'a', 1, 'c')
(1, 'a', 2, 'a')
(1, 'a', 2, 'b')
(1, 'a', 2, 'c')
...
```
2. itertools.permutations(iterable, r=None)
该函数用于生成迭代器的排列,其中参数iterable表示可迭代对象,r表示每个排列中元素的个数。例如:
```
import itertools
a = [1, 2, 3]
b = itertools.permutations(a, 2)
for i in b:
print(i)
```
输出:
```
(1, 2)
(1, 3)
(2, 1)
(2, 3)
(3, 1)
(3, 2)
```
3. itertools.combinations(iterable, r)
该函数用于生成迭代器的组合,其中参数iterable表示可迭代对象,r表示每个组合中元素的个数。例如:
```
import itertools
a = [1, 2, 3]
b = itertools.combinations(a, 2)
for i in b:
print(i)
```
输出:
```
(1, 2)
(1, 3)
(2, 3)
```
以上是itertools模块中的部分常用函数的使用方法,该模块还包含其他函数,具体用法可以参考官方文档。Python的itertools是一个内置模块,提供了很多用于处理迭代器和生成器的工具函数。以下是itertools中一些常用函数的用法:
1. itertools.count(start=0, step=1)
生成一个从start开始的无限迭代器,步长为step。
2. itertools.cycle(iterable)
对于可迭代对象,将其无限重复下去。
3. itertools.repeat(object, times=None)
将对象重复times次,如果没有指定times则无限重复。
4. itertools.chain(*iterables)
将多个可迭代对象连接在一起,返回一个迭代器。
5. itertools.islice(iterable, start, stop[, step])
从可迭代对象中按照指定的索引切片,返回一个迭代器。
6. itertools.groupby(iterable[, key])
根据key函数将可迭代对象分组,返回(key, group)的迭代器。
7. itertools.combinations(iterable, r)
从可迭代对象中取出r个元素的组合,返回一个迭代器。
8. itertools.permutations(iterable, r=None)
从可迭代对象中取出r个元素的排列,返回一个迭代器。
9. itertools.product(*iterables, repeat=1)
对多个可迭代对象做笛卡尔积,返回一个迭代器。
以上是itertools中一些常用的函数,还有一些其他的函数,可根据需求使用。
itertools 是 Python 中的一个内置模块,用于操作迭代对象的函数。它提供了一组用于处理迭代对象的功能,其中包括排列、组合、过滤器和分组等。itertools是Python标准库中的一个模
itertools是python中的一种内置模块,可以帮助开发者更容易地处理迭代对象。它提供了一系列迭代器工具,可以进行快速、高效、灵活的数据处理。例如,可以使用itertools.accumulate来计算累计和,使用itertools.chain来将多个迭代器连接成一个,使用itertools.groupby来对数据进行分组,等等。Python中的itertools模块提供了一些用于迭代器和循环的工具函数。以下是itertools模块中常用的一些函数:
1. itertools.chain(*iterables):将多个迭代器连接成一个迭代器。
2. itertools.cycle(iterable):对可迭代对象中的元素反复执行循环。
3. itertools.repeat(object[, times]):将一个元素重复生成指定次数,或者无限重复生成。
4. itertools.count(start=0, step=1):生成从指定起始数开始,以指定步长递增的无限整数序列。
5. itertools.islice(iterable, start, stop[, step]):切片迭代器,返回从起始位置到终止位置之间的元素。
6. itertools.combinations(iterable, r):返回可迭代对象中长度为r的所有组合。
7. itertools.permutations(iterable, r=None):返回可迭代对象中长度为r的所有排列。
8. itertools.product(*iterables, repeat=1):返回可迭代对象的笛卡尔积。
9. itertools.groupby(iterable, key=None):将迭代器中的元素按照指定键函数分组。
除了以上这些常用的函数之外,itertools模块还提供了许多其他有用的函数,如zip_longest、tee、accumulate等等。itertools是Python中的一个模块,它包含了一系列用于生成迭代器的工具函数。下面是几个常用的itertools函数及其用法:
1. count(start=0, step=1)
生成一个从start开始、步长为step的无限迭代器。
2. cycle(iterable)
将可迭代对象重复无限次,生成一个无限迭代器。
3. chain(*iterables)
将多个可迭代对象连接起来,生成一个新的迭代器。
4. permutations(iterable, r=None)
生成可迭代对象的所有排列,如果指定r,则只生成长度为r的排列。
5. combinations(iterable, r)
生成可迭代对象的所有组合,只生成长度为r的组合。
6. product(*iterables, repeat=1)
生成可迭代对象的笛卡尔积,可以指定重复次数。
7. groupby(iterable, key=None)
将可迭代对象中相邻的、具有相同key的元素分组,生成一个迭代器。
使用itertools模块可以让Python的迭代器使用更加高效、方便。
itertools模块提供了各种函数来帮助我们处理迭代对象(Iterators),比如chain(), cycle(), compress(), dropwhile(), groupby()等等。它们可以帮助我们更加快捷地处理迭代对象。itertools是Python标准库中的一个模块,它提供了许多用于迭代器操作的函数。下面是itertools常用的函数:
1. count(start, step):返回一个无限迭代器,从start开始,以step为步长地生成数值。
2. cycle(iterable):对于一个可迭代对象,无限重复它的元素。
3. repeat(elem, n=None):重复elem n次或无限次。
4. chain(*iterables):将多个可迭代对象连接成一个迭代器。
5. zip_longest(*iterables, fillvalue=None):将多个可迭代对象的元素一一对应地打包成元组,若长度不一则以fillvalue填充。
6. permutations(iterable, r=None):返回iterable中长度为r的所有排列。
7. combinations(iterable, r):返回iterable中长度为r的所有组合。
8. product(*iterables, repeat=1):返回多个可迭代对象的笛卡尔积。
除了这些,itertools还提供了其他一些有用的函数,可以根据需求灵活使用。Python的itertools是一个标准库,包含一些用于迭代器和生成器的工具函数。它提供了一些简单的、高效的方法来创建迭代器,这些迭代器可以被用于解决各种问题。
以下是itertools的一些常见用法:
1. itertools.chain(iter1, iter2, ...): 将多个迭代器串联起来,返回一个新的迭代器,它会依次返回每个迭代器中的元素。
2. itertools.count(start=0, step=1): 从指定的start开始不断返回一个数值,每次递增step。
3. itertools.cycle(iterable): 无限地重复迭代一个可迭代对象。
4. itertools.islice(iterable, start, stop[, step]): 返回一个迭代器,它返回可迭代对象中从start到stop之间的元素,步长itertools是Python中一个常用的模块,主要用于高效地生成各种迭代器。
常用的itertools函数包括:
1. count(start=0, step=1): 从start开始,以step为步长生成一个无限迭代器。
2. cycle(iterable): 生成一个无限迭代器,不断重复iterable中的元素。
3. repeat(elem, n=None): 生成一个迭代器,不断重复elem,如果指定了n,则最多重复n次。
4. chain(*iterables): 将多个可迭代对象连接成一个迭代器,返回的迭代器包含所有可迭代对象中的元素。
5. product(*iterables, repeat=1): 生成一个迭代器,返回iterables中所有可迭代对象的笛卡尔积,如果指定了repeat,则表示对每个可迭代对象进行重复的次数。
6. combinations(iterable, r): 生成一个迭代器,返回iterable中长度为r的所有组合,不考虑顺序。
7. permutations(iterable, r=None): 生成一个迭代器,返回iterable中长度为r的所有排列,考虑顺序,如果不指定r,则默认为len(iterable)。
8. groupby(iterable, key=None): 生成一个迭代器,按照key函数对iterable中的元素进行分组,返回一个由(key, group)组成的迭代器,其中key表示分组的键,group表示分组后的元素集合。
以上是itertools中常用的几个函数,使用itertools可以方便地进行迭代器操作,提高代码的效率和可读性。
itertools是Python中一个模块,它提供了多种迭代器功能,可以帮助用户快速构建复杂的迭代器。它的用法比较简单,只需要根据需要使用不同的函数,就可以快速构建出迭代器,用以获取相应的迭代器序列。itertools是Python中的一个标准库,用于处理迭代器和循环中的数据。它提供了一些用于高效处理迭代器的工具函数。
以下是itertools库中一些常用的函数:
1. count(start=0, step=1):生成一个无限迭代器,从start开始,每次增加step。
2. cycle(iterable):对于可迭代对象,生成一个无限迭代器,将可迭代对象的内容无限循环输出。
3. repeat(elem, n=None):生成一个迭代器,重复elem n次或无限重复。
4. chain(*iterables):将多个可迭代对象连接成一个迭代器,依次输出每个可迭代对象中的元素。
5. compress(data, selectors):将data和selectors打包,根据selectors的值筛选出data中相应位置的元素。
6. dropwhile(predicate, iterable):依次迭代iterable中的元素,当predicate为True时,跳过元素,直到第一个predicate为False的元素。
7. takewhile(predicate, iterable):依次迭代iterable中的元素,当predicate为True时,输出元素,直到第一个predicate为False的元素。
8. groupby(iterable, key=None):对iterable中的元素进行分组,返回一个生成器,每次输出一个元素及其对应的组别。
以上仅是itertools库中一些常用的函数,更多的函数可以查看Python官方文档。itertools 是 Python 中一个内置模块,它提供了一些用于迭代器操作的函数,包括:
1. itertools.count(start=0, step=1):从 start 开始不断地向上加 step 生成数字,相当于一个无限大的数列。
2. itertools.cycle(iterable):将一个可迭代对象变成一个循环的迭代器。
3. itertools.chain(*iterables):将多个可迭代对象连接起来,形成一个新的迭代器。
4. itertools.islice(iterable, start, stop, step=1):对迭代器进行切片操作,返回一个新的迭代器。
5. itertools.product(*iterables, repeat=1):对多个可迭代对象进行笛卡尔积操作,返回一个新的迭代器。
6. itertools.permutations(iterable, r=None):对可迭代对象进行全排列操作,返回一个新的迭代器。
7. itertools.combinations(iterable, r):对可迭代对象进行组合操作,返回一个新的迭代器。
8. itertools.combinations_with_replacement(iterable, r):对可迭代对象进行带重复元素的组合操作,返回一个新的迭代器。
通过使用 itertools 模块提供的这些函数,我们可以轻松地对迭代器进行各种操作,从而更加高效地完成任务。itertools是Python标准库中提供的一个工具包,用于生成迭代器以及对迭代器进行操作和处理。
下面是itertools中常用函数的介绍:
1. itertools.chain(*iterables):将多个可迭代对象合并成一个迭代器返回。
2. itertools.count(start=0, step=1):生成一个从start开始,步长为step的无限迭代器。
3. itertools.cycle(iterable):将可迭代对象重复无限次返回。
4. itertools.dropwhile(predicate, iterable):返回一个迭代器,包含iterable中predicate为False后的所有元素。
5. itertools.groupby(iterable, key=None):将iterable中连续的相同元素分组,并返回由元素和对应的迭代器组成的元组。
6. itertools.islice(iterable, start, stop[, step]):返回一个迭代器,从start开始到stop结束,步长为step。
7. itertools.permutations(iterable, r=None):返回iterable中r个元素的所有排列。
8. itertools.product(*iterables, repeat=1):返回iterables中每个可迭代对象的笛卡尔积,repeat参数指定重复迭代的次数。
9. itertools.repeat(object[, times]):重复生成object,times参数指定重复的次数。
10. itertools.takewhile(predicate, iterable):返回一个迭代器,包含iterable中predicate为True的元素,一旦predicate为False就停止迭代。
以上是itertools中常用的函数,可以根据需要进行使用。
itertools是Python中的一个模块,它提供了一系列用于操作迭代对象的函数。它可以帮助我们以有效、优雅的方式处理迭代问题。例如,使用它可以实现链式迭代,以及使用 groupby() 函数将迭代器中的元素按照某个键进行分组。itertools是Python标准库中一个用于高效操作迭代器的模块,包含了许多用于迭代器操作的函数和生成器。常用的itertools函数包括:
1. itertools.count(start=0, step=1):从start开始按照step递增生成无限序列。
2. itertools.cycle(iterable):对于iterable中的元素,无限重复循环生成。
3. itertools.repeat(object, times=None):生成重复times次的object元素。
4. itertools.chain(*iterables):将多个迭代器拼接在一起生成一个更长的迭代器。
5. itertools.islice(iterable, start, stop[, step]):从iterable中的第start个元素开始,每step个元素取一个,直到第stop个元素结束,生成一个新的迭代器。
6. itertools.compress(data, selectors):根据selectors中的元素来选择data中的元素生成一个新的迭代器。
7. itertools.filterfalse(predicate, iterable):过滤掉满足predicate条件的元素,生成一个新的迭代器。
8. itertools.groupby(iterable, key=None):将iterable中的元素按照key函数返回值的相等性分组生成一个新的迭代器,可以进行分组统计等操作。
除此之外,itertools模块中还有许多其他有用的函数和生成器,具体用法可以查看Python官方文档。Python中的itertools是一个用于生成迭代器的标准库模块,它包含了许多用于操作迭代器的工具函数。下面是一些常用的itertools函数及其用法:
1. itertools.count(start=0, step=1):生成一个从start开始,以step为步长的无限迭代器。
2. itertools.cycle(iterable):生成一个无限迭代器,重复iterable中的元素。
3. itertools.repeat(object, times=None):生成一个重复object的迭代器,重复次数可以通过times参数指定。
4. itertools.chain(*iterables):将多个迭代器合并成一个迭代器。
5. itertools.islice(iterable, start, stop[, step]):返回一个切片对象,用于对迭代器进行切片操作。
6. itertools.dropwhile(predicate, iterable):返回一个迭代器,跳过iterable中满足predicate条件的元素,直到第一个不满足条件的元素为止。
7. itertools.takewhile(predicate, iterable):返回一个迭代器,输出iterable中满足predicate条件的元素,直到第一个不满足条件的元素为止。
8. itertools.product(*iterables, repeat=1):返回多个迭代器的笛卡尔积,repeat参数指定重复次数。
9. itertools.permutations(iterable, r=None):返回可迭代对象的所有排列,r参数指定排列长度,默认为原可迭代对象长度。
10. itertools.combinations(iterable, r):返回可迭代对象中r个元素的组合。
以上是一些itertools常用函数的介绍,使用itertools可以方便地处理各种迭代器的操作。itertools是Python标准库中一个非常实用的模块,它提供了很多用于迭代器操作的工具函数。下面是itertools中几个常用函数的介绍:
1. permutations(iterable, r=None):返回可迭代对象中所有长度为r的排列,如果不指定r则返回所有排列。
2. combinations(iterable, r):返回可迭代对象中所有长度为r的组合。
3. combinations_with_replacement(iterable, r):返回可迭代对象中所有长度为r的组合,允许元素重复。
4. product(*iterables, repeat=1):返回可迭代对象中所有元素的笛卡尔积。
5. cycle(iterable):将可迭代对象无限重复下去。
6. chain(*iterables):将多个可迭代对象连接起来,返回一个迭代器。
7. groupby(iterable, key=None):按照指定的key函数对可迭代对象进行分组,返回一个迭代器,每个元素是一个(key, group)的二元组。
这些函数可以方便地用于处理序列、集合和其他可迭代对象。在需要对序列进行排列、组合、笛卡尔积等操作时,可以使用itertools中的函数,从而避免手动编写循环等代码,提高编程效率。Python中的itertools是一个用于迭代器和循环的模块,提供了一些方便实用的工具函数,可以帮助我们更高效地处理迭代任务。
itertools中常用的函数包括:
1. permutations(iterable, r=None):返回iterable中所有长度为r(默认为可迭代对象长度)的排列。
2. combinations(iterable, r):返回iterable中所有长度为r的组合。
3. product(*iterables, repeat=1):返回iterables中所有可能的笛卡尔积元组。
4. chain(*iterables):将多个可迭代对象连接起来形成一个迭代器。
5. count(start=0, step=1):返回一个无限迭代器,每次递增step的值,从start开始。
6. cycle(iterable):将可迭代对象重复循环输出,直到外部中断。
7. groupby(iterable, key=None):对可迭代对象进行分组,返回分组后的结果。
这些函数可以帮助我们更高效地实现迭代任务,节省开发时间和资源。Python中的itertools模块提供了许多用于迭代器和迭代工具的函数。以下是itertools中一些常用函数的用法:
1. itertools.chain(*iterables): 将多个迭代器连接成一个迭代器,返回一个新的迭代器。用法示例:
```
import itertools
a = [1, 2, 3]
b = ['a', 'b', 'c']
c = itertools.chain(a, b)
for i in c:
print(i)
```
输出结果为:
```
1
2
3
a
b
c
```
2. itertools.combinations(iterable, r): 返回iterable中长度为r的所有组合,每个组合都是元组。用法示例:
```
import itertools
a = [1, 2, 3, 4]
b = itertools.combinations(a, 2)
for i in b:
print(i)
```
输出结果为:
```
(1, 2)
(1, 3)
(1, 4)
(2, 3)
(2, 4)
(3, 4)
```
3. itertools.product(*iterables, repeat=1): 返回iterables中所有元素的笛卡尔积,每个元素都是元组。repeat参数指定了每个元素在结果中出现的次数。用法示例:
```
import itertools
a = [1, 2]
b = ['a', 'b']
c = itertools.product(a, b, repeat=2)
for i in c:
print(i)
```
输出结果为:
```
(1, 'a', 1, 'a')
(1, 'a', 1, 'b')
(1, 'a', 2, 'a')
(1, 'a', 2, 'b')
(1, 'b', 1, 'a')
(1, 'b', 1, 'b')
(1, 'b', 2, 'a')
(1, 'b', 2, 'b')
(2, 'a', 1, 'a')
(2, 'a', 1, 'b')
(2, 'a', 2, 'a')
(2, 'a', 2, 'b')
(2, 'b', 1, 'a')
(2, 'b', 1, 'b')
(2, 'b', 2, 'a')
(2, 'b', 2, 'b')
```Python的itertools模块是一个用于高效生成迭代器的模块,提供了一系列用于迭代器生成的工具函数。这些工具函数可以用于处理迭代器,例如可以用来生成排列、组合、笛卡尔积等,常用的函数有:
1. itertools.count(start=0, step=1):返回一个无限递增的迭代器,从start开始,步长为step。
2. itertools.cycle(iterable):返回一个无限循环的迭代器,不断重复iterable中的元素。
3. itertools.repeat(object[, times]):返回一个重复times次的迭代器,如果不指定times,则会无限重复。
4. itertools.chain(*iterables):返回一个将多个迭代器连接在一起的迭代器。
5. itertools.compress(data, selectors):返回一个根据selectors筛选data中元素的迭代器。
6. itertools.groupby(iterable[, key]):返回一个按照key分组的迭代器。
7. itertools.permutations(iterable[, r]):返回一个iterable的r个元素的排列的迭代器。
8. itertools.combinations(iterable, r):返回一个iterable的r个元素的组合的迭代器。
9. itertools.product(*iterables, repeat=1):返回多个迭代器的笛卡尔积的迭代器,repeat指定重复次数。
这些函数可以让我们在处理迭代器时更加高效和便捷。itertools是Python标准库中一个非常实用的模块,它提供了很多用于迭代器的工具函数。以下是itertools模块中常用的一些函数:
1. count(start=0, step=1):从start开始以step为步长无限生成数字。
2. cycle(iterable):将可迭代对象无限循环输出。
3. repeat(elem, n=None):重复输出elem元素n次,若n为None,则会一直重复输出。
4. chain(*iterables):将多个可迭代对象链接在一起输出。
5. tee(iterable, n=2):将一个可迭代对象分成n份,返回一个元组,元组中包含n个迭代器,每个迭代器都可以独立地迭代原始对象。
6. zip_longest(*iterables, fillvalue=None):将多个可迭代对象中的元素按照位置打包成元组,若可迭代对象长度不一致,则使用fillvalue填充缺失的值。
7. permutations(iterable, r=None):生成可迭代对象中所有长度为r的排列,若r为None,则生成所有排列。
8. combinations(iterable, r):生成可迭代对象中所有长度为r的组合。
9. product(*iterables, repeat=1):生成多个可迭代对象的笛卡尔积。itertools是Python标准库中的一个模块,提供了一些用于高效遍历、组合和迭代元素的工具函数。以下是itertools中常用的几个函数及其用法:
1. itertools.count(start=0, step=1)
该函数生成一个无限迭代器,每次迭代递增step,默认从0开始递增。
示例代码:
```
import itertools
for i in itertools.count():
if i > 10:
break
print(i)
```
输出结果:
```
0
1
2
3
4
5
6
7
8
9
10
```
2. itertools.cycle(iterable)
该函数生成一个无限迭代器,不断重复可迭代对象中的元素。
示例代码:
```
import itertools
colors = ['red', 'green', 'blue']
color_cycle = itertools.cycle(colors)
for i in range(6):
print(next(color_cycle))
```
输出结果:
```
red
green
blue
red
green
blue
```
3. itertools.chain(*iterables)
该函数将多个可迭代对象连接起来,返回一个新的迭代器。
示例代码:
```
import itertools
numbers = [1, 2, 3]
letters = ['a', 'b', 'c']
combined = itertools.chain(numbers, letters)
for i in combined:
print(i)
```
输出结果:
```
1
2
3
a
b
c
```
4. itertools.permutations(iterable, r=None)
该函数返回可迭代对象中所有长度为r的排列组合,如果不指定r,则返回所有排列组合。
示例代码:
```
import itertools
letters = ['a', 'b', 'c']
permutations = itertools.permutations(letters, r=2)
for i in permutations:
print(i)
```
输出结果:
```
('a', 'b')
('a', 'c')
('b', 'a')
('b', 'c')
('c', 'a')
('c', 'b')
```
以上是itertools中常用的几个函数及其用法,还有其他函数如itertools.combinations、itertools.product等,可以根据具体需求选择使用。Python的itertools模块提供了很多用于处理迭代器和生成器的工具函数。这些函数可以用于构建高效的迭代器,例如用于组合、排列、笛卡尔积、重复元素、截取元素等等。
下面是itertools中一些常用的函数和用法:
1. combinations(iterable, r):返回iterable中长度为r的所有组合。
2. permutations(iterable, r=None):返回iterable中长度为r的所有排列,如果r未指定,则返回所有排列。
3. product(*iterables, repeat=1):返回所有iterables中元素的笛卡尔积,repeat表示每个iterable的重复次数。
4. chain(*iterables):将多个iterables连接成一个大的迭代器。
5. count(start=0, step=1):返回一个无限迭代器,从start开始每次递增step。
6. cycle(iterable):对iterable进行循环迭代。
7. repeat(object, times=None):重复object,times表示重复次数。
以上是itertools模块中一些常用的函数和用法,还有其他的工具函数,可以根据需要进行查找和使用。itertools是Python标准库中的一个模块,提供了许多用于迭代器操作的函数。以下是itertools中常用的函数:
1. permutations(iterable, r=None): 返回iterable中所有长度为r的排列。如果r未指定,则默认为iterable的长度。
2. combinations(iterable, r): 返回iterable中所有长度为r的组合。
3. product(*iterables, repeat=1): 返回iterables中每个可迭代对象的笛卡尔积,其中repeat指定重复迭代的次数。
4. chain(*iterables): 将多个可迭代对象连接起来形成一个单一的迭代器。
5. zip_longest(*iterables, fillvalue=None): 返回迭代器中每个可迭代对象的迭代器,并且当其中一个迭代器用尽时,用fillvalue填充,直到所有可迭代对象都用尽。
除此之外,itertools中还有其他的函数,包括groupby、accumulate、islice、count等等。这些函数可以让你轻松地对迭代器进行操作,并生成新的迭代器。Python中的itertools是一个标准库,用于生成和处理迭代器,可以帮助我们更高效地处理循环和迭代过程。以下是itertools的常见用法:
1. 生成无限迭代器:itertools.count(start=0, step=1)和itertools.cycle(iterable)
2. 生成有限迭代器:itertools.islice(iterable, start, stop[, step])和itertools.compress(data, selectors)
3. 对迭代器进行排列组合:itertools.permutations(iterable, r=None)和itertools.combinations(iterable, r)
4. 对多个迭代器进行操作:itertools.chain(*iterables)和itertools.zip_longest(*iterables, fillvalue=None)
5. 对迭代器进行分组操作:itertools.groupby(iterable, key=None)和itertools.tee(iterable, n=2)
以上是itertools的部分用法,通过使用itertools,可以避免使用循环过程中频繁创建临时变量的问题,提高代码的效率和可读性。Python的itertools模块是一个标准库,提供了用于迭代器操作的各种工具函数,例如组合、排列、笛卡尔积、重复元素等等。
以下是itertools模块的一些常用函数及其用法:
1. itertools.product(*iterables, repeat=1):返回iterables中每个元素的笛卡尔积的元组,repeat指定重复元素的次数。
2. itertools.permutations(iterable, r=None):返回iterable中r个元素的所有排列,默认r等于iterable的长度。
3. itertools.combinations(iterable, r):返回iterable中r个元素的所有组合。
4. itertools.chain(*iterables):将多个迭代器连接成一个迭代器。
5. itertools.cycle(iterable):将一个可迭代对象变成一个循环迭代器,无限重复。
6. itertools.repeat(object, times=None):重复object times次,或无限重复。
7. itertools.islice(iterable, start, stop[, step]):返回iterable的迭代器的切片,类似于列表的切片操作。
8. itertools.groupby(iterable, key=None):将迭代器分组为一个个key和group的二元组。
以上是itertools模块的一些常用函数及其用法,可以帮助我们更方便地进行迭代器操作。Python中的itertools是一个用于生成迭代器的模块,包含了一系列用于生成迭代器的函数。以下是一些常用的itertools函数及其用法:
1. count(start=0, step=1):生成从start开始的无限递增的迭代器,步长为step。
2. cycle(iterable):将可迭代对象重复无限次,并生成一个新的迭代器。
3. repeat(elem, n=None):生成一个重复elem的迭代器,重复n次(默认为无限次)。
4. chain(*iterables):将多个可迭代对象连接成一个迭代器,返回的是一个新的迭代器。
5. islice(iterable, start, stop[, step]):对可迭代对象进行切片操作,返回一个迭代器。
6. permutations(iterable, r=None):生成可迭代对象中所有长度为r(默认为可迭代对象长度)的排列组合。
7. combinations(iterable, r):生成可迭代对象中所有长度为r的组合。
8. product(*iterables, repeat=1):生成可迭代对象中所有元素的笛卡尔积,可以使用repeat参数指定重复的次数。
以上是itertools中的一些常用函数,它们可以帮助我们更方便地生成迭代器,减少代码的重复性。Python的itertools模块提供了一些非常有用的工具,可以用于迭代器的快速和高效处理。以下是itertools模块的一些常用方法:
1. itertools.chain():将多个迭代器合并为一个迭代器。
2. itertools.cycle():将一个序列重复无限次,可以用于循环处理。
3. itertools.count():生成一个无限的计数器。
4. itertools.islice():对迭代器进行切片操作。
5. itertools.compress():使用一个布尔序列来过滤迭代器中的元素。
6. itertools.permutations():生成给定序列的所有排列。
7. itertools.combinations():生成给定序列的所有组合。
8. itertools.product():生成多个迭代器的笛卡尔积。
9. itertools.groupby():根据指定的键对迭代器进行分组。
这些方法可以方便地处理迭代器中的数据,提高了处理数据的效率和可读性。
相关推荐
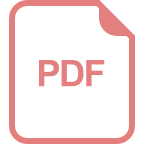
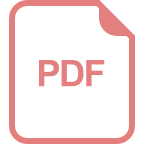












