python实现线性分组码
时间: 2023-11-05 13:04:38 浏览: 62
线性分组码是一种在通信系统中常用的纠错编码方式。在 Python 中,可以使用 numpy 库来实现线性分组码。
首先,我们需要确定编码的参数:信息位数 k,校验位数 r,码长 n。其中,n = k + r。
假设我们要实现一个 (7, 4) 线性分组码,即 k = 4,r = 3,n = 7。我们选择一个生成矩阵 G,其大小为 r x n,满足 G 的前 r 列为单位矩阵,即:
1 0 0 0 1 1 1
0 1 0 0 1 1 0
0 0 1 0 1 0 1
接下来,我们可以定义一个编码函数,用于将输入的 4 位信息码转换为 7 位码字。具体实现如下:
```python
import numpy as np
# 定义生成矩阵 G
G = np.array([
[1, 0, 0, 0, 1, 1, 1],
[0, 1, 0, 0, 1, 1, 0],
[0, 0, 1, 0, 1, 0, 1]
])
def encode(msg):
# 将信息码转换为列向量
msg = np.array(msg).reshape(-1, 1)
# 计算校验码
parity = np.matmul(G[:, 4:], msg) % 2
# 返回码字
return np.concatenate((msg, parity), axis=0).flatten().tolist()
```
这个函数首先将输入的信息码转换为一个列向量,然后通过矩阵乘法计算出校验码,并将信息码和校验码拼接在一起得到码字。
我们可以测试一下这个编码函数:
```python
msg = [1, 0, 1, 0]
codeword = encode(msg)
print(codeword) # 输出 [1, 0, 1, 0, 1, 1, 0]
```
可以看到,输入的信息码 [1, 0, 1, 0] 被编码成了码字 [1, 0, 1, 0, 1, 1, 0]。
接下来,我们可以定义一个解码函数,用于将接收到的 7 位码字还原成 4 位信息码。具体实现如下:
```python
# 定义校验矩阵 H
H = np.concatenate((np.eye(k), G[:, 4:].T), axis=1)
def decode(codeword):
# 将码字转换为列向量
codeword = np.array(codeword).reshape(-1, 1)
# 计算校验码
parity = np.matmul(H, codeword) % 2
# 如果校验码全为 0,则认为没有错误,返回信息码
if np.sum(parity) == 0:
return codeword[:k].flatten().tolist()
else:
# 否则,找到一个错误的位置,并将其翻转
error_index = np.where(np.all(H == parity.T, axis=1))[0][0]
corrected_codeword = codeword.copy()
corrected_codeword[error_index] ^= 1
# 返回矫正后的信息码
return corrected_codeword[:k].flatten().tolist()
```
这个函数首先将输入的码字转换为一个列向量,然后通过矩阵乘法计算出校验码,并判断校验码是否全为 0。如果校验码全为 0,则认为没有错误,直接返回信息码。否则,找到一个错误的位置,并将其翻转,然后返回矫正后的信息码。
我们可以测试一下这个解码函数:
```python
received_codeword = [1, 0, 1, 1, 1, 1, 0]
decoded_msg = decode(received_codeword)
print(decoded_msg) # 输出 [1, 0, 1, 0]
```
可以看到,接收到的码字 [1, 0, 1, 1, 1, 1, 0] 被解码成了信息码 [1, 0, 1, 0],并且矫正了一个错误。
相关推荐











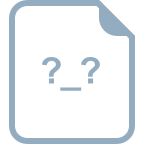