如何将文本按行写入csv
时间: 2023-09-27 12:11:08 浏览: 94
文件中?
您可以使用Python内置的CSV模块来实现此操作。下面是代码示例:
```python
import csv
# 打开要写入的csv文件
with open('data.csv', mode='w', newline='') as file:
writer = csv.writer(file)
# 要写入的文本
text = "第一行文本\n第二行文本\n第三行文本"
# 按行分割文本,写入CSV文件
lines = text.split('\n')
for line in lines:
writer.writerow([line])
```
代码中,首先使用`csv.writer`创建一个写入器,将其绑定到打开的CSV文件上。然后,将要写入CSV文件的文本按行分割,再将每一行作为一个列表写入到CSV文件中。注意,每一行需要用方括号包装成一个列表,以便使用`writerow`方法写入CSV文件中的一行。
相关问题
将文本写入csv
好的,您可以使用Python的csv模块来将文本写入csv文件。下面是一个简单的示例代码:
```python
import csv
# 假设您有一个包含文本的列表
text_list = ["Hello", "world", "!", "How", "are", "you", "?"]
# 打开csv文件以写入模式
with open('example.csv', mode='w', newline='') as file:
writer = csv.writer(file)
# 使用writerows方法将列表中的文本写入csv文件中的一行
writer.writerow(text_list)
```
这将在当前目录下创建一个名为`example.csv`的csv文件,并将`text_list`中的文本写入其中。您可以根据需要修改文件名和文件路径。
c++读取txt文本内容写入csv表格
您可以使用C++的文件操作和字符串处理来读取txt文本内容并将其写入csv表格。
首先,您需要包含必要的头文件:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <vector>
```
然后,您可以编写一个函数来读取txt文件内容并将其写入csv表格:
```cpp
void convertTxtToCsv(const std::string& txtFilePath, const std::string& csvFilePath)
{
std::ifstream txtFile(txtFilePath);
std::ofstream csvFile(csvFilePath);
if (!txtFile.is_open())
{
std::cout << "Failed to open txt file!" << std::endl;
return;
}
if (!csvFile.is_open())
{
std::cout << "Failed to create csv file!" << std::endl;
return;
}
std::string line;
while (std::getline(txtFile, line))
{
std::vector<std::string> fields;
std::stringstream ss(line);
std::string field;
// 将line中的数据按照分隔符逐个读取到fields中
while (std::getline(ss, field, ',')) // 根据具体的分隔符修改此处
{
fields.push_back(field);
}
// 将fields中的数据按照CSV格式写入csv文件中
for (const std::string& field : fields)
{
csvFile << "\"" << field << "\",";
}
csvFile << std::endl;
}
txtFile.close();
csvFile.close();
std::cout << "Conversion completed!" << std::endl;
}
```
在上述代码中,`txtFilePath`是要读取的txt文件路径,`csvFilePath`是要生成的csv文件路径。您需要根据实际情况修改分隔符,例如上述代码使用的是逗号作为分隔符。
最后,您可以调用这个函数来进行转换:
```cpp
std::string txtFilePath = "path_to_txt_file.txt";
std::string csvFilePath = "path_to_csv_file.csv";
convertTxtToCsv(txtFilePath, csvFilePath);
```
请将`path_to_txt_file.txt`替换为您要读取的txt文件的实际路径,将`path_to_csv_file.csv`替换为您要生成的csv文件的实际路径。
这样,您就可以使用C++读取txt文本内容并将其写入生成的csv表格中了。
阅读全文
相关推荐
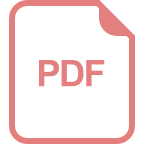
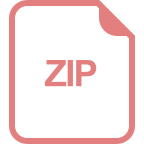












