将进行中文分词之后的结果写入csv中
时间: 2024-03-01 09:55:30 浏览: 57
你可以使用Python中的csv库将中文分词后的结果写入csv文件中。以下是一个简单的示例代码:
```python
import jieba
import csv
# 读入文本文件
with open('text.txt', 'r', encoding='utf-8') as file:
text = file.read()
# 中文分词
words = jieba.cut(text)
# 写入csv文件
with open('result.csv', 'w', encoding='utf-8', newline='') as file:
writer = csv.writer(file)
for word in words:
# 将分词结果写入csv文件中
writer.writerow([word])
```
在上面的代码中,我们首先使用jieba库对文本进行中文分词。然后,我们使用csv库创建一个csv文件,并将分词结果写入该文件中。注意,我们使用`newline=''`参数来避免在写入csv文件时出现多余的空行。
相关问题
python词频统计结果写入csv,Python中CSV列的词频
好的,针对您的问题,我可以给出以下代码实现词频统计结果写入CSV文件中:
```python
import csv
from collections import Counter
# 读取文本文件
with open('text.txt', 'r') as f:
text = f.read()
# 分词
words = text.split()
# 统计词频
word_counts = Counter(words)
# 将词频结果写入CSV文件
with open('word_counts.csv', 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(['Word', 'Frequency'])
for word, count in word_counts.items():
writer.writerow([word, count])
```
在这个代码中,我们首先使用 `with open()` 语句读取文本文件,然后使用 `split()` 方法将文本分词存储到 `words` 变量中。接下来,我们使用 `Counter()` 函数统计词频,并将结果存储到 `word_counts` 变量中。最后,使用 `csv.writer()` 函数将结果写入CSV文件中。
需要注意的是,我们使用 `newline=''` 参数来避免生成空行。`writerow()` 方法用于写入一行数据,每行数据由两个列组成,分别表示单词和出现的频率。
phython读取文本文件中的内容,统计其频率最高的10个单词,将结果写入CSV文件中
好的,我可以帮你解决这个问题。你可以使用Python中的collections模块来实现单词频率的统计。具体的实现步骤如下:
1. 首先,你需要打开文本文件并读取其中的内容。可以使用Python中的open()函数和read()方法实现。
```python
with open('file.txt', 'r') as f:
text = f.read()
```
2. 接着,你需要将读取的文本内容进行分词,可以使用Python中的nltk库或者jieba库实现。这里以nltk为例:
```python
import nltk
tokens = nltk.word_tokenize(text.lower())
```
3. 然后,你可以使用collections模块中的Counter类对单词进行统计,并取出频率最高的前10个单词。
```python
from collections import Counter
word_count = Counter(tokens)
top_words = word_count.most_common(10)
```
4. 最后,你可以将统计结果写入CSV文件中,可以使用Python中的csv库实现。
```python
import csv
with open('result.csv', 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(['Word', 'Frequency'])
for word, count in top_words:
writer.writerow([word, count])
```
以上就是Python读取文本文件中的内容,统计其频率最高的10个单词,并将结果写入CSV文件中的完整代码。
阅读全文
相关推荐
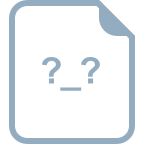
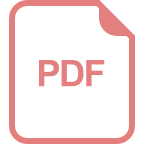
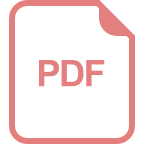














