python 识别图像中的黑线,获取距离视野底部一定距离的黑线上的点的像素坐标
时间: 2024-04-20 20:22:51 浏览: 34
要识别图像中的黑线并获取距离视野底部一定距离的黑线上的点的像素坐标,你可以使用以下步骤:
1. 导入必要的库:
```python
import cv2
import numpy as np
```
2. 加载图像:
```python
image = cv2.imread('image.jpg')
```
3. 将图像转换为灰度图像:
```python
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
4. 对图像进行边缘检测:
```python
edges = cv2.Canny(gray, 50, 150)
```
5. 对边缘图像进行霍夫直线变换检测直线:
```python
lines = cv2.HoughLinesP(edges, 1, np.pi/180, threshold=100, minLineLength=100, maxLineGap=10)
```
6. 遍历检测到的直线,并找到距离视野底部一定距离的黑线上的点的像素坐标:
```python
distance_from_bottom = 100 # 距离视野底部的距离
for line in lines:
x1, y1, x2, y2 = line[0]
if y1 > (image.shape[0] - distance_from_bottom) and y2 > (image.shape[0] - distance_from_bottom):
# 在此处可以根据需要对点进行进一步处理,例如保存坐标或绘制到图像上
cv2.circle(image, (x1, y1), 5, (0, 255, 0), -1)
cv2.circle(image, (x2, y2), 5, (0, 255, 0), -1)
```
7. 显示结果:
```python
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,上述代码仅为一种基本的方法,结果可能因图像质量、阈值设定等因素而有所变化。你可能需要根据实际情况进行参数调整和进一步的图像处理。
相关推荐
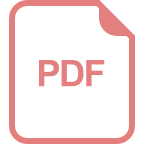
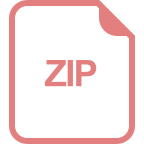














