yolov5数据增强的示例
时间: 2023-09-08 13:16:23 浏览: 52
以下是使用YoloV5进行数据增强的示例代码:
```python
import cv2
import numpy as np
from PIL import Image
from pathlib import Path
import albumentations as A
from albumentations.pytorch.transforms import ToTensorV2
from tqdm import tqdm
# 定义数据增强函数
def get_augmentations(phase):
if phase == 'train':
return A.Compose([
A.RandomSizedCrop(min_max_height=(800, 800), height=1024, width=1024, p=0.5),
A.OneOf([
A.HorizontalFlip(p=1),
A.VerticalFlip(p=1),
A.RandomRotate90(p=1),
A.Rotate(limit=20, border_mode=cv2.BORDER_CONSTANT, value=0, p=1),
A.RandomBrightnessContrast(p=1),
A.RGBShift(r_shift_limit=15, g_shift_limit=15, b_shift_limit=15, p=1),
A.Blur(blur_limit=3, p=1),
A.GaussNoise(var_limit=(10.0, 50.0), p=1),
], p=0.5),
A.Resize(height=512, width=512, p=1),
ToTensorV2(p=1.0),
], bbox_params=A.BboxParams(format='coco', label_fields=['labels']))
else:
return A.Compose([
A.Resize(height=512, width=512, p=1),
ToTensorV2(p=1.0),
], bbox_params=A.BboxParams(format='coco', label_fields=['labels']))
# 定义数据加载函数
def load_image_and_annotations(image_path, annotations_path):
image = np.array(Image.open(image_path).convert('RGB'))
annotations = np.loadtxt(annotations_path)
labels = annotations[:, 0].astype(np.int64)
bboxes = annotations[:, 1:]
return image, {'labels': labels, 'bboxes': bboxes}
# 定义输出函数
def save_image_and_annotations(image, annotations, output_dir, basename):
output_image_path = Path(output_dir) / (basename + '.jpg')
output_annotations_path = Path(output_dir) / (basename + '.txt')
Image.fromarray(image).save(output_image_path)
np.savetxt(output_annotations_path, annotations, fmt='%d %.6f %.6f %.6f %.6f')
# 定义数据增强的主函数
def augment_images(input_dir, output_dir, phase):
input_dir = Path(input_dir)
output_dir = Path(output_dir)
output_dir.mkdir(parents=True, exist_ok=True)
augmentations = get_augmentations(phase)
image_paths = sorted(input_dir.glob('*.jpg'))
annotation_paths = sorted(input_dir.glob('*.txt'))
for image_path, annotation_path in tqdm(zip(image_paths, annotation_paths), total=len(image_paths)):
image, annotations = load_image_and_annotations(image_path, annotation_path)
transformed = augmentations(**{'image': image, 'bboxes': annotations['bboxes'], 'labels': annotations['labels']})
transformed_image = transformed['image']
transformed_annotations = np.hstack((np.expand_dims(transformed['labels'], axis=1), transformed['bboxes']))
save_image_and_annotations(transformed_image, transformed_annotations, output_dir, image_path.stem)
```
在上述代码中,我们首先定义了`get_augmentations()`函数,用于返回训练和验证阶段的数据增强函数。接下来,我们定义了`load_image_and_annotations()`函数,用于加载图像和标注。然后,我们定义了`save_image_and_annotations()`函数,用于保存增强后的图像和标注。最后,我们定义了`augment_images()`函数,它接受输入和输出目录以及阶段参数,并对输入目录中的所有图像进行数据增强,然后将增强后的图像和标注保存到输出目录中。
我们可以使用以下命令来运行数据增强的示例代码:
```python
input_dir = 'path/to/input/dir'
output_dir = 'path/to/output/dir'
# 训练集增强
augment_images(input_dir, output_dir, phase='train')
# 验证集增强
augment_images(input_dir, output_dir, phase='val')
```
其中,`input_dir`是包含原始图像和标注的目录,`output_dir`是增强后的图像和标注保存的目录。`phase`参数可以设置为`'train'`或`'val'`,用于指定增强的阶段。
相关推荐
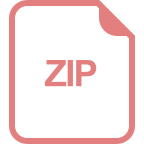
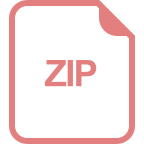














