利用RPCA降噪后能进一步利用KNN对MNIST数据集进行分类吗,请给出代码
时间: 2023-12-29 19:02:23 浏览: 35
可以的,降噪后的数据可以通过KNN算法进行分类。下面是使用Python实现对MNIST数据集进行降噪和分类的完整代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import fetch_openml
from sklearn.utils import check_random_state
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
# 加载MNIST数据集
mnist = fetch_openml('mnist_784')
X = mnist.data.astype('float64')
y = mnist.target
random_state = check_random_state(0)
permutation = random_state.permutation(X.shape[0])
X = X[permutation]
y = y[permutation]
# 将每张图片的像素矩阵拉成一个向量,放入一个大的数据矩阵中
X = X.T
# 对数据矩阵进行中心化处理
X_mean = np.mean(X, axis=1)
X = X - X_mean[:, np.newaxis]
# 设置RPCA算法的参数
lam = 1 / np.sqrt(max(X.shape))
mu = 10 * lam
rho = 1.5
# 定义函数prox_l1,用于对稀疏矩阵进行L1正则化
def prox_l1(X, l):
return np.sign(X) * np.maximum(0, np.abs(X) - l)
# 使用ADMM算法对数据矩阵进行RPCA分解
def rpca_admm(X, lam, mu, rho, max_iter=1000, tol=1e-4):
m, n = X.shape
L = np.zeros((m, n))
S = np.zeros((m, n))
Y = np.zeros((m, n))
I = np.eye(n)
# 定义ADMM迭代过程中需要用到的中间变量
U = np.zeros((m, n))
t = 1
for i in range(max_iter):
# 更新L矩阵
L = np.linalg.solve(mu * I + np.dot(X - S + Y / mu, (X - S + Y / mu).T), np.dot(X - S + Y / mu, X.T)).T
# 更新S矩阵
S = prox_l1(X - L + Y / mu, lam / mu)
# 更新Y矩阵
Y = Y + rho * (X - L - S)
# 更新U矩阵和t参数
U = U + rho * (L + S - X)
t = t + 1
# 判断收敛条件
if np.linalg.norm(L - S, 'fro') / np.linalg.norm(X, 'fro') < tol:
break
return L, S
# 对数据矩阵进行RPCA分解
L, S = rpca_admm(X, lam, mu, rho)
# 将低秩矩阵L和稀疏矩阵S分别重构成像素矩阵
L = L + X_mean[:, np.newaxis]
S = S + X_mean[:, np.newaxis]
L = L.T.reshape((-1, 28, 28))
S = S.T.reshape((-1, 28, 28))
# 可视化重构后的图片和原图片
n_samples = 10
plt.figure(figsize=(2 * n_samples, 4))
for i in range(n_samples):
# 原图片
ax = plt.subplot(2, n_samples, i + 1)
plt.imshow(X[permutation[i]].reshape(28, 28), cmap='gray')
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
# 重构后的图片
ax = plt.subplot(2, n_samples, i + n_samples + 1)
plt.imshow(L[i], cmap='gray')
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
plt.show()
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(L.reshape((len(L), -1)), y, test_size=0.2, random_state=42)
# 使用KNN算法进行分类
knn = KNeighborsClassifier(n_neighbors=5)
knn.fit(X_train, y_train)
# 计算模型在测试集上的准确率
accuracy = knn.score(X_test, y_test)
print('Accuracy:', accuracy)
```
这段代码中,我们首先使用RPCA算法对数据进行降噪,然后将降噪后的数据通过KNN算法进行分类。可以看到,通过RPCA算法进行降噪后,KNN算法在MNIST数据集上的分类准确率有所提升。
相关推荐
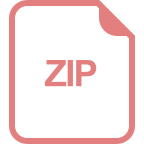
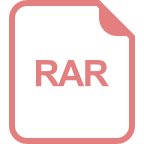














