使用OGR / GDAL确定shapefile和栅格在Python中是否重叠
时间: 2024-03-05 20:52:38 浏览: 23
在Python中,可以使用OGR/GDAL库来确定shapefile和栅格是否重叠。具体步骤如下:
1. 导入必要的库
```python
from osgeo import ogr, gdal
```
2. 打开shapefile,并获取其空间参考
```python
shapefile = ogr.Open("path/to/shapefile.shp")
layer = shapefile.GetLayer()
spatialRef = layer.GetSpatialRef()
```
3. 打开栅格文件,并获取其空间参考和变换矩阵
```python
rasterfile = gdal.Open("path/to/rasterfile.tif")
geotransform = rasterfile.GetGeoTransform()
spatialRef = rasterfile.GetProjection()
```
4. 将shapefile的空间参考和栅格的空间参考进行比较
```python
if spatialRef == layer.GetSpatialRef().ExportToWkt():
print("The shapefile and raster have the same spatial reference.")
else:
print("The shapefile and raster have different spatial references.")
```
5. 获取shapefile的范围,并将其转换为栅格坐标系中的范围
```python
# 获取shapefile的范围
extent = layer.GetExtent()
# 将shapefile的范围转换为栅格坐标系中的范围
ulx, uly = gdal.ApplyGeoTransform(geotransform, extent[0], extent[3])
lrx, lry = gdal.ApplyGeoTransform(geotransform, extent[1], extent[2])
```
6. 获取栅格的范围
```python
xsize = rasterfile.RasterXSize
ysize = rasterfile.RasterYSize
x_min = geotransform[0]
y_max = geotransform[3]
x_max = x_min + geotransform[1] * xsize
y_min = y_max + geotransform[5] * ysize
```
7. 判断shapefile和栅格是否重叠
```python
if ulx < x_max and lrx > x_min and uly > y_min and lry < y_max:
print("The shapefile and raster overlap.")
else:
print("The shapefile and raster do not overlap.")
```
注意,上述代码中的shapefile和rasterfile的路径需要根据实际情况进行修改。另外,由于shapefile和栅格的范围可能不完全重合,因此上述代码中的重叠判断是基于两者的边界框进行的。如果需要更精确的重叠判断,可以使用更复杂的方法,例如将栅格转换为矢量数据,然后进行空间分析。
相关推荐
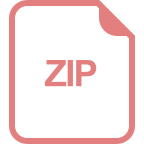
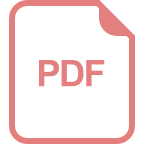
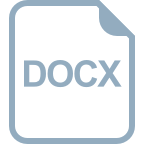
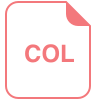
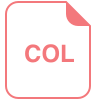












