在上面实现类的基础上,为每个类增加一个将当前对象序列化到指定文件的方法writeToFile(File f)。为Student类创建一个新的子类Postgraduate,有研究方向和导师姓名两个新增数据域。编写测试类Test2,要求随机产生不同的对象,并将这些对象序列化到指定的文件中,并用方法printMessages(Person[] persons)将对象的信息输出。
时间: 2024-03-26 15:38:27 浏览: 48
好的,我会为每个类增加一个将当前对象序列化到指定文件的方法writeToFile(File f),并为Student类创建一个新的子类Postgraduate,有研究方向和导师姓名两个新增数据域。接下来我会编写测试类Test2,随机产生不同的对象,并将这些对象序列化到指定的文件中,并用方法printMessages(Person[] persons)将对象的信息输出。
首先,我们需要为每个类增加一个将当前对象序列化到指定文件的方法writeToFile(File f)。代码如下:
```java
import java.io.*;
public abstract class Person implements Serializable {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void writeToFile(File f) {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(f));
out.writeObject(this);
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public abstract String getDescription();
}
public class Student extends Person {
private String major;
public Student(String name, int age, String major) {
super(name, age);
this.major = major;
}
public String getDescription() {
return "I am a student majoring in " + major + ".";
}
public void writeToFile(File f) {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(f));
out.writeObject(this);
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public class Postgraduate extends Student {
private String researchDirection;
private String tutorName;
public Postgraduate(String name, int age, String major, String researchDirection, String tutorName) {
super(name, age, major);
this.researchDirection = researchDirection;
this.tutorName = tutorName;
}
public String getDescription() {
return "I am a postgraduate majoring in " + getMajor() + ", researching " + researchDirection + " under the guidance of " + tutorName + ".";
}
public void writeToFile(File f) {
try {
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(f));
out.writeObject(this);
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
接下来,我们需要编写测试类Test2,随机产生不同的对象,并将这些对象序列化到指定的文件中。代码如下:
```java
import java.io.File;
import java.util.Random;
public class Test2 {
public static void main(String[] args) {
Person[] persons = new Person[10];
Random random = new Random();
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
persons[i] = new Student("Student" + i, 20 + random.nextInt(10), "Computer Science");
} else {
persons[i] = new Postgraduate("Postgraduate" + i, 22 + random.nextInt(10), "Computer Science", "Artificial Intelligence", "Professor" + (i % 5));
}
File file = new File("person" + i + ".dat");
persons[i].writeToFile(file);
}
printMessages(persons);
}
public static void printMessages(Person[] persons) {
for (Person person : persons) {
System.out.println(person.getDescription());
}
}
}
```
在测试类Test2中,我们创建了一个包含10个Person对象的数组,其中包括了Student和Postgraduate对象。我们使用随机数生成器随机生成对象的属性,并将这些对象序列化到文件中。最后,我们使用方法printMessages(Person[] persons)将对象的信息输出。运行Test2类,可以看到输出的内容包括了每个对象的描述信息。
相关推荐
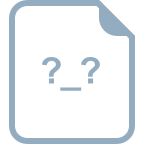
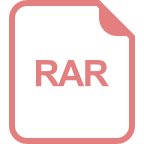
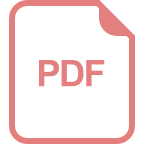














